A guide to implementing selection sort in Python
How to use Python for selection sort
Selection sort is a simple but less efficient sorting algorithm. Its basic idea is to select the smallest (or largest) element from the data to be sorted each time and place it at the end of the sorted sequence. Repeat this process multiple times until all data is sorted.
The following will introduce in detail how to use Python for selection sorting and provide specific code examples.
- First, define a selection sort function, named selection_sort, which accepts a list to be sorted as a parameter.
def selection_sort(lst): n = len(lst) for i in range(n-1): min_index = i # 记录当前最小值的索引 for j in range(i+1, n): if lst[j] < lst[min_index]: min_index = j lst[i], lst[min_index] = lst[min_index], lst[i] # 将最小值交换到已排序序列的末尾
- Call the selection_sort function in the main program and pass in the list to be sorted. The following is an example:
lst = [64, 25, 12, 22, 11] selection_sort(lst) print("排序后的列表:", lst)
The output result is:
排序后的列表: [11, 12, 22, 25, 64]
The above is a specific code example of using Python for selection sorting. The execution of the code is further explained below.
In selection sorting, we implement it through two levels of loops. The outer loop controls the starting position of selecting the smallest element from the unsorted subsequence each time, while the inner loop is used to find the smallest element in the current unsorted subsequence. By comparing the current element with the smallest element that has been selected, we can get the index of the smallest element in the subsequence.
After finding the smallest element, we swap it with the last element of the sorted sequence, so that the smallest element is placed at the end of the sorted sequence. By repeating this process, each time selecting the smallest element and placing it at the end of the sorted sequence, we end up with an ordered list.
It should be noted that the time complexity of selection sort is O(n^2), where n is the number of elements to be sorted. Although its efficiency is relatively low, selection sort is still a simple and easy-to-implement sorting algorithm when the data size is small.
I hope the above content will help you understand and use Python for selection sorting. If you have any other questions, please ask.
The above is the detailed content of A guide to implementing selection sort in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
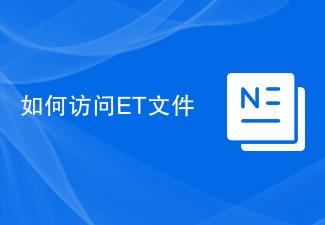
ET file is a very common file format, which is usually generated by the table editor in WPS software. Before getting into ET files, we can first understand what ET files are, and then discuss how to open and edit them. ET files are the file format of WPS spreadsheet software, similar to XLS or XLSX files in Microsoft Excel. WPS spreadsheet is a powerful spreadsheet software that provides Excel-like functions for data processing, data analysis and chart creation.
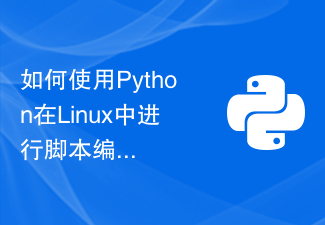
How to use Python to write and execute scripts in Linux In the Linux operating system, we can use Python to write and execute various scripts. Python is a concise and powerful programming language that provides a wealth of libraries and tools to make scripting easier and more efficient. Below we will introduce the basic steps of how to use Python for script writing and execution in Linux, and provide some specific code examples to help you better understand and use it. Install Python
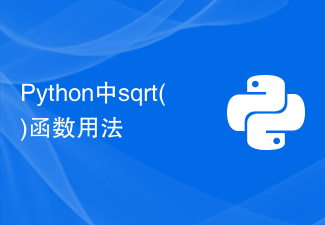
Usage and code examples of the sqrt() function in Python 1. Function and introduction of the sqrt() function In Python programming, the sqrt() function is a function in the math module, and its function is to calculate the square root of a number. The square root means that a number multiplied by itself equals the square of the number, that is, x*x=n, then x is the square root of n. The sqrt() function can be used in the program to calculate the square root. 2. How to use the sqrt() function in Python, sq
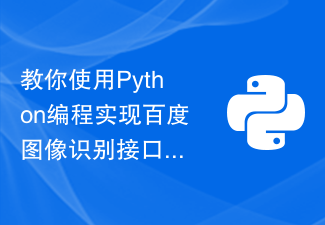
Teach you to use Python programming to implement the docking of Baidu's image recognition interface and realize the image recognition function. In the field of computer vision, image recognition technology is a very important technology. Baidu provides a powerful image recognition interface through which we can easily implement image classification, labeling, face recognition and other functions. This article will teach you how to use the Python programming language to realize the image recognition function by connecting to the Baidu image recognition interface. First, we need to create an application on Baidu Developer Platform and obtain

How to do image processing and recognition in Python Summary: Modern technology has made image processing and recognition an important tool in many fields. Python is an easy-to-learn and use programming language with rich image processing and recognition libraries. This article will introduce how to use Python for image processing and recognition, and provide specific code examples. Image processing: Image processing is the process of performing various operations and transformations on images to improve image quality, extract information from images, etc. PIL library in Python (Pi
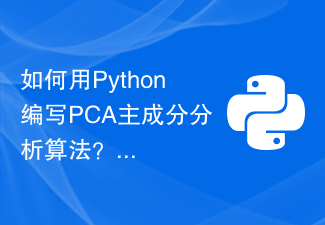
How to write PCA principal component analysis algorithm in Python? PCA (Principal Component Analysis) is a commonly used unsupervised learning algorithm used to reduce the dimensionality of data to better understand and analyze data. In this article, we will learn how to write the PCA principal component analysis algorithm using Python and provide specific code examples. The steps of PCA are as follows: Standardize the data: Zero the mean of each feature of the data and adjust the variance to the same range to ensure
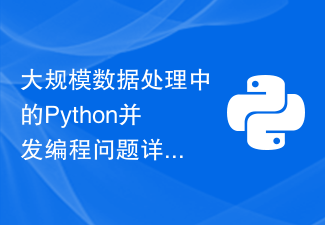
Detailed explanation of Python concurrent programming issues in large-scale data processing In today's era of data explosion, large-scale data processing has become an important task in many fields. For processing massive amounts of data, improving processing efficiency is crucial. In Python, concurrent programming can effectively improve the execution speed of the program, thereby processing large-scale data more efficiently. However, there are also some issues and challenges with concurrent programming, especially in large-scale data processing. Below we will analyze and solve some common Python concurrent programming problems, and
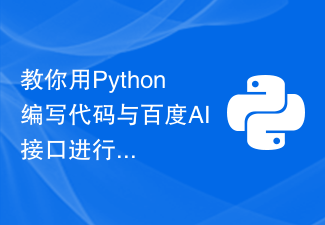
Teach you to use Python to write code and connect with Baidu AI interface 1. Background introduction: With the development of artificial intelligence, Baidu provides a wealth of AI interfaces to meet developers' needs for intelligence. When using these AI interfaces, we can use Python to write code to interface with Baidu AI interfaces to implement various interesting functions. 2. Preparation: Register a Baidu developer account and create a project: Visit the Baidu Smart Cloud official website, register an account in the Developer Center, and create a new project. Get AP
