Basic syntax and definition of Java interface classes
Basic syntax and definition methods of Java interface classes
In Java programming, an interface (Interface) is a special class used to define a set of methods Specification, but does not provide specific implementation. Interface classes play an important role in code design, helping to implement code modularization and providing flexible extensibility and polymorphism. This article will introduce the basic syntax and definition method of interface classes, with specific code examples.
1. Declaration and definition of interface class
In Java, you can use the keyword "interface" to declare and define an interface class. An interface class is an abstract class that cannot be instantiated and can only be used by classes that implement the interface.
The syntax of the interface class is as follows:
public interface 接口名 { // 定义接口方法 方法返回类型 方法名(参数列表); // ... }
2. Characteristics and precautions of the interface class
- The methods defined in the interface class default It is an abstract method and does not contain specific implementation content, only the method signature. Therefore, the "abstract" keyword cannot be used to modify methods in interface classes.
- Constants can be defined in interface classes, but ordinary variables and instance methods cannot be included.
- A class can implement one or more interface classes and are associated through the "implements" keyword.
- The class that implements the interface class must implement all abstract methods in the interface class, otherwise the class needs to be declared as an abstract class.
- Methods in interface classes are public by default, that is, using the "public" modifier, they can be accessed directly through the interface name.
3. Example of definition of interface class
The following uses an example to specifically illustrate the definition and use of interface class.
Define an interface class (Animal.java):
public interface Animal { // 声明抽象方法 void eat(); void sleep(); }
Implement the interface class (Cat.java):
public class Cat implements Animal { // 实现接口中的抽象方法 @Override public void eat() { System.out.println("Cat is eating."); } @Override public void sleep() { System.out.println("Cat is sleeping."); } }
Implementation interface class (Dog.java):
public class Dog implements Animal { // 实现接口中的抽象方法 @Override public void eat() { System.out.println("Dog is eating."); } @Override public void sleep() { System.out.println("Dog is sleeping."); } }
Test class (Main.java):
public class Main { public static void main(String[] args) { Animal cat = new Cat(); // 定义一个接口类对象 Animal dog = new Dog(); // 定义一个接口类对象 cat.eat(); // 调用接口方法 cat.sleep(); dog.eat(); dog.sleep(); } }
Program running results :
Cat is eating. Cat is sleeping. Dog is eating. Dog is sleeping.
In the above example, we defined an interface class Animal, and implemented the abstract methods in the interface in the implementation classes Cat and Dog. By creating an object of the interface class and calling the corresponding method, the specific function is completed.
To sum up, this article introduces the basic syntax and definition method of Java interface classes in detail, including the declaration and definition of interfaces, characteristics and precautions, and demonstrates the use of interface classes through sample code. Interface classes are widely used in Java programming. They can provide code modularization and flexible scalability, and bring a lot of convenience to our programming work.
The above is the detailed content of Basic syntax and definition of Java interface classes. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


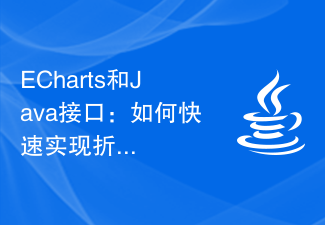
ECharts and Java interface: How to quickly implement statistical charts such as line charts, bar charts, and pie charts. Specific code examples are required. With the advent of the Internet era, data analysis has become more and more important. Statistical charts are a very intuitive and powerful display method. Charts can display data more clearly, allowing people to better understand the connotation and patterns of the data. In Java development, we can use ECharts and Java interfaces to quickly display various statistical charts. ECharts is a software developed by Baidu
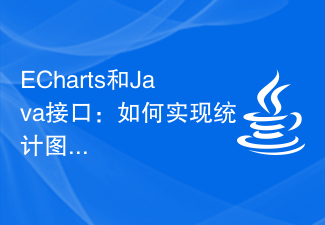
ECharts is a powerful, flexible and customizable open source chart library that can be used for data visualization and large-screen display. In the era of big data, the data export and sharing functions of statistical charts have become increasingly important. This article will introduce how to implement the statistical chart data export and sharing functions of ECharts through the Java interface, and provide specific code examples. 1. Introduction to ECharts ECharts is a data visualization library based on JavaScript and Canvas open sourced by Baidu, with rich charts.
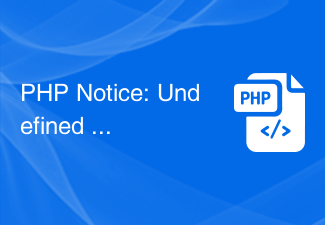
In PHP development, we often encounter the error message PHPNotice:Undefinedvariable. This error message means that we have used an undefined variable in the code. Although this error message will not cause the code to crash, it will affect the readability and maintainability of the code. Below, this article will introduce you to some methods to solve this error. 1. Use the error_reporting(E_ALL) function during the development process. In PHP development, we can
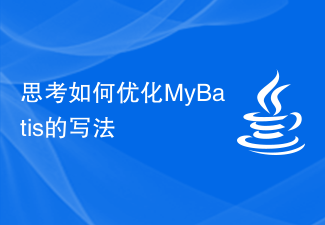
Rethink the way MyBatis is written MyBatis is a very popular Java persistence framework that can help us simplify the writing process of database operations. However, in daily use, we often encounter some confusions and bottlenecks in writing methods. This article will rethink the way MyBatis is written and provide some specific code examples to help readers better understand and apply MyBatis. Use the Mapper interface to replace SQL statements in the traditional MyBatis writing method.
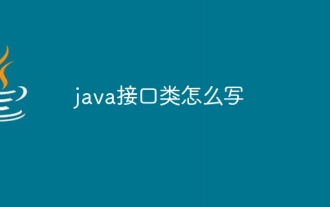
Writing method: 1. Define an interface named MyInterface; 2. Define a method named myMethod() in the MyInterface interface; 3. Create a class named MyClass and implement the MyInterface interface; 4. Create a MyClass class Object and assign its reference to a variable of type MyInterface.
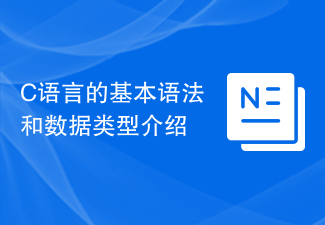
C language is a widely used computer programming language that is efficient, flexible and powerful. To be proficient in programming in C language, you first need to understand its basic syntax and data types. This article will introduce the basic syntax and data types of C language and give examples. 1. Basic syntax 1.1 Comments In C language, comments can be used to explain the code to facilitate understanding and maintenance. Comments can be divided into single-line comments and multi-line comments. //This is a single-line comment/*This is a multi-line comment*/1.2 Keyword C language
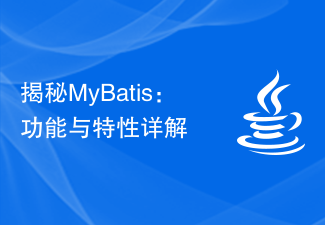
MyBatis is a popular Java persistence layer framework that simplifies the process of database operations, provides control over SQL mapping, and is simple, flexible, and powerful. This article will deeply analyze the functions and characteristics of MyBatis, and explain it in detail through specific code examples. 1. The role of MyBatis 1.1 Simplification of database operations: MyBatis binds SQL statements to Java methods by providing SQL mapping files, shielding the cumbersome operations of traditional JDBC calls.
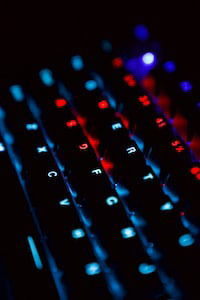
Interface: An implementationless contract interface defines a set of method signatures in Java but does not provide any concrete implementation. It acts as a contract that forces classes that implement the interface to implement its specified methods. The methods in the interface are abstract methods and have no method body. Code example: publicinterfaceAnimal{voideat();voidsleep();} Abstract class: Partially implemented blueprint An abstract class is a parent class that provides a partial implementation that can be inherited by its subclasses. Unlike interfaces, abstract classes can contain concrete implementations and abstract methods. Abstract methods are declared with the abstract keyword and must be overridden by subclasses. Code example: publicabstractcla
