Concrete type error snippet in Golang
I am trying out error wrapping in go and have a function that returns a wrapped custom error type. What I want to do is iterate over a list of expected errors and test whether the function's output contains these expected errors.
I found that putting the custom error into []error
means that the type of the custom error will be *fmt.wraperror
, which means errors.as( )
almost always returns true.
As an example, consider the following code:
package main import ( "errors" "fmt" ) type anothererror struct { } func (e *anothererror) error() string { return "another error" } type missingattrerror struct { missingattr string } func (e *missingattrerror) error() string { return fmt.sprintf("missing attribute: %s", e.missingattr) } func dosomething() error { e := &missingattrerror{missingattr: "key"} return fmt.errorf("dosomething(): %w", e) } func main() { err := dosomething() expectederrone := &missingattrerror{} expectederrtwo := &anothererror{} expectederrs := []error{expectederrone, expectederrtwo} fmt.printf("is err '%v' type '%t'?: %t\n", err, expectederrone, errors.as(err, &expectederrone)) fmt.printf("is err '%v' type '%t'?: %t\n", err, expectederrtwo, errors.as(err, &expectederrtwo)) for i := range expectederrs { fmt.printf("is err '%v' type '%t'?: %t\n", err, expectederrs[i], errors.as(err, &expectederrs[i])) } }
The output is
is err 'dosomething(): missing attribute: key' type '*main.missingattrerror'?: true is err 'dosomething(): missing attribute: key' type '*main.anothererror'?: false is err 'dosomething(): missing attribute: key' type '*fmt.wraperror'?: true is err 'dosomething(): missing attribute: key' type '*fmt.wraperror'?: true
Ideally I would like the output to be
Is err 'DoSomething(): missing attribute: Key' type '*main.MissingAttrError'?: true Is err 'DoSomething(): missing attribute: Key' type '*main.AnotherError'?: false Is err 'DoSomething(): missing attribute: Key' type '*main.MissingAttrError'?: true Is err 'DoSomething(): missing attribute: Key' type '*main.AnotherError'?: false
The reason for the error is that I want to be able to define a list of expected errors for each test case entry. Suppose I know that providing certain inputs to a function will cause it to follow a path and return errors containing a specific error.
How do I convert the *fmt.wraperror
type from the []error
slice back to the original type so that I can use it with error.as
?
I know I can use to cast it to a specific type. (anothererror)
, but in order to make it work when iterating over slices, I have to do this for every possible error the function might return, no? )
Correct answer
You can cheat using the following method errors.as
:
func main() { err := DoSomething() m := &MissingAttrError{} a := &AnotherError{} expected := []any{&m, &a} for i := range expected { fmt.Printf("Is err '%v' type '%T'?: %t\n", err, expected[i], errors.As(err, expected[i])) } }
The type printed is not what you expect, but errors.as
works as it should.
The reason your example doesn't work is that what you're passing to errors.as
is *error
. Therefore, the wrapped error value (i.e. err
) is assigned directly to the target value. In my example, the value passed to errors.as
is **anothererror
, and err
is not assignable to *anothererror
.
The above is the detailed content of Concrete type error snippet in Golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


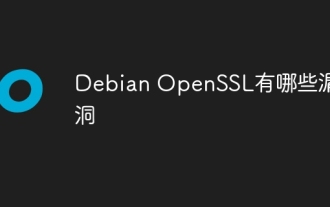
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
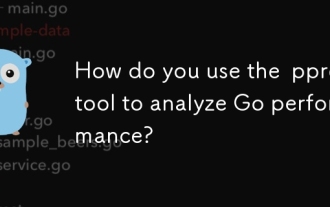
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
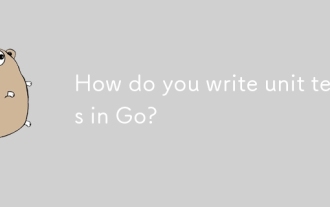
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
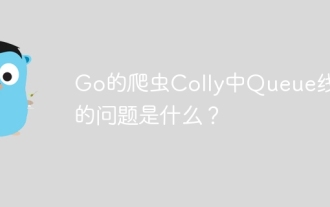
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
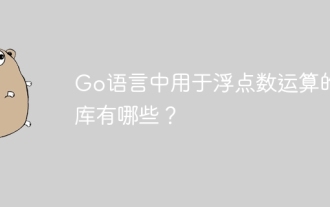
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
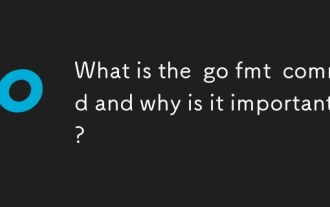
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
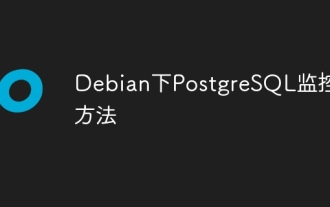
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
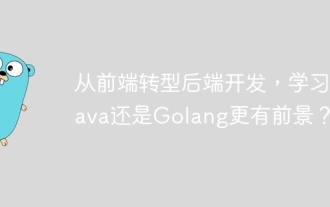
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
