How to convert bytes to float32 array in Go?
I am writing a float32 array of numbers in byte format from a Python script to an Elasticache Redis cluster, and then from Elasticache in a Go script Read bytes (as string). How to convert bytes-as-string back to original float32 array in Go script?
Python example:
import numpy as np import redis a = np.array([1.1, 2.2, 3.3], dtype=np.float32) a_bytes = a.tobytes(order="C") #I have also tried order="F" with no luck print(a_bytes) #Output: b'\xcd\xcc\x8c?\xcd\xcc\x0c@33S@' redis_client = redis.cluster.RedisCluster(host=<elasticache config endpoint>, port=6379) redis_client.mset_nonatomic({"key1": a_bytes})
Here is an example of what I tried in Go (playground)
package main import ( "fmt" "math" "strconv" ) func main() { // aBytesStr is an example value retrieved from Elasticache // aBytesStr is type string, not raw bytes var aBytesStr string = "\xcd\xcc\x8c?\xcd\xcc\x0c@33S@" aHex := fmt.Sprintf("%X", aBytesStr) fmt.Println(aHex) // Output: CDCC8C3FCDCC0C4033335340 var aArr [3]float32 for i := 0; i < 3; i++ { aHex1 := aHex[i*8 : i*8+8] aParsed, err := strconv.ParseUint(aHex1, 16, 32) if err != nil { return } aArr[i] = math.Float32frombits(uint32(aParsed)) } fmt.Println(aArr) // Expected output: [1.1 2.2 3.3] // Actual output [-4.289679e+08 -4.2791936e+08 4.17524e-08] }
Correct answer
The example code you used is "convert hex to string"; you have raw bytes (I think based on aHex:CDCC8C3FCDCC0C4033335340
), so it's simpler to convert directly (although you could convert the bytes to a hex string and then do the conversion, which would just add unnecessary work/complexity).
From this answer we get (Playground):
func GetFloatArray(aBytes []byte) []float32 { aArr := make([]float32, 3) for i := 0; i < 3; i++ { aArr[i] = BytesFloat32(aBytes[i*4:]) } return aArr } func BytesFloat32(bytes []byte) float32 { bits := binary.LittleEndian.Uint32(bytes) float := math.Float32frombits(bits) return float }
Updated reference comments:
I'm still a little confused by what you received, so let's try these two possibilities.
If the redis query returns raw data (bytes) as a go string (i.e. "\xcd\xcc\x8c?\xcd\xcc\x0c@33S@"
), then you can Convert to []byte
(Playground)
func main() { var aBytesStr string = "\xcd\xcc\x8c?\xcd\xcc\x0c@33S@" fmt.Println(GetFloatArray([]byte(aBytesStr))) }
If Redis returns a string containing ASCII (/UTF-8) representation (i.e. CDCC
= []byte{0x41, 0x44, 0x43, 0x43}
), the final An easy way might be to decode this using encoding/hex( playground)
func main() { aHex := "CDCC8C3FCDCC0C4033335340" b, err := hex.DecodeString(aHex) if err != nil { panic(err) } fmt.Println(GetFloatArray(b)) }
Please note that your original approach may work, however, as pointed out in the comments above, you need to deal with Endianness so the following will work (playground - you can improve Efficiency, my purpose is for clarity):
byte1 := aHex[i*8 : i*8+2] byte2 := aHex[i*8+2 : i*8+4] byte3 := aHex[i*8+4 : i*8+6] byte4 := aHex[i*8+6 : i*8+8] aParsed, err := strconv.ParseUint(byte4+byte3+byte2+byte1, 16, 32)
However, this makes assumptions about the CPU running the code, which means the previous answer is preferable.
The above is the detailed content of How to convert bytes to float32 array in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Since its inception in 2009, Bitcoin has become a leader in the cryptocurrency world and its price has experienced huge fluctuations. To provide a comprehensive historical overview, this article compiles Bitcoin price data from 2009 to 2025, covering major market events, changes in market sentiment, and important factors influencing price movements.

1. First, right-click on the blank space of the taskbar at the bottom of Windows 11 and select [Taskbar Settings]. 2. Find [taskbarcorneroverflow] on the right in the taskbar settings. 3. Then find [clock] or [Clock] above it and select to turn it on. Method 2: 1. Press the keyboard shortcut [win+r] to call up run, enter [regedit] and press Enter to confirm. 2. Open the registry editor, find [HKEY_CURRENT_USERControlPanel] in it, and delete it. 3. After deletion, restart the computer and you will be prompted for configuration. When you return to the system, the time will be displayed.
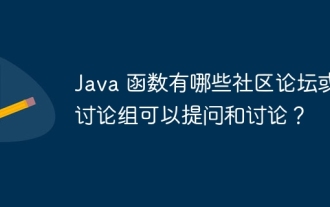
Answer: The following community forums and discussion groups are available for Java functional programming questions: StackOverflow: The world's largest programming Q&A website with a community of Java functional programming experts. JavaFunctionalProgramming: A community forum focused on Java functional programming, providing discussions on concepts, language features, and best practices. Redditr/functionaljava: A subreddit focused on functional programming in Java, focusing on tools, libraries, and technologies. Discord: JavaFunctional Programming: Discord service that provides real-time discussion, code sharing and collaboration
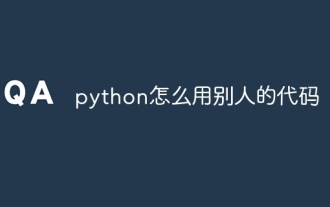
How do I use other people's Python code? Find code repositories: Find the code you need on platforms like PyPI and GitHub. Installation code: Use pip or clone the GitHub repository to install. Import modules: Use the import statement in your script to import installed modules. Working with code: Access functions and classes in modules. (Optional) Adapt the code: Modify the code as needed to fit your project.

What should I do if the time on my win11 computer is always wrong? We all set the time or calendar when using win11 system, but many users are asking that the computer time is always wrong, so what is going on? Users can directly click on the taskbar below, and then find taskbarcorneroverflow to set it up. Let this site introduce to users in detail how to adjust the time error on Win11 computers. How to adjust the computer time error in Windows 11. Method 1: 1. We first right-click on the blank space of the taskbar below and select Taskbar Settings. Method 2: 1. Press the keyboard shortcut win+r to call up run, enter regedit and press Enter to confirm.
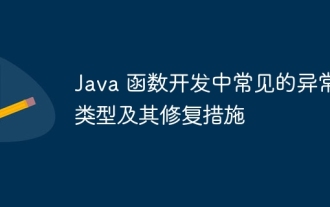
Common exception types and their repair measures in Java function development During the development of Java functions, various exceptions may be encountered, which affect the correct execution of the function. The following are common exception types and their repair measures: 1. NullPointerException Description: Thrown when accessing an object that has not been initialized. Fix: Make sure you check the object for non-null before using it. Sample code: try{Stringname=null;System.out.println(name.length());}catch(NullPointerExceptione){
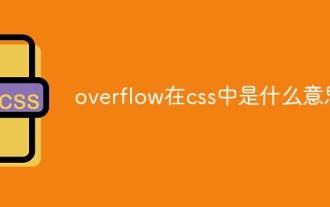
overflow is a property of CSS that is used to control the display mode of element content when it exceeds the container. Available values include: visible: the content is visible, the overflow container is hidden: the overflow content is cut scroll: the scroll bar is displayed to view the overflow content auto: the browser automatically determines Whether to display the scroll bar inherit: inherit the overflow attribute of the parent element
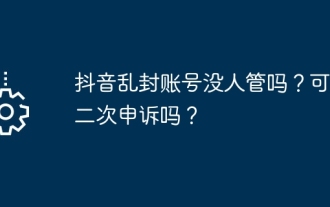
As a world-renowned short video platform, Douyin has a huge user base and content creators. However, as the platform rules are constantly updated and improved, some users may encounter account bans. This has raised public questions about the transparency and fairness of platform management. This article will discuss the issue of Douyin account bans and whether users have ways to appeal after their accounts are banned. There may be many reasons for being banned on the Douyin platform, including but not limited to illegal content, violation of platform regulations, infringement of other people's rights, etc. In order to maintain the order of the platform and the interests of users, Douyin has set up a series of rules and review mechanisms. When some users violate the rules, their accounts may be banned. However, some users may question or be dissatisfied with the reasons for the ban
