Basic setup for Golang SQL unit testing using dockertest
I am using dockertest to execute sql unit tests. This is just a simple connection to *sqlx.db
, but when connecting to the database it somehow generates the error error: eof
. I can't identify the error, I may have misconfigured it.
import ( "fmt" "log" "os" "testing" _ "github.com/lib/pq" "github.com/jmoiron/sqlx" "github.com/ory/dockertest/v3" "github.com/ory/dockertest/v3/docker" ) var ( host = "localhost" user = "postgres" password = "postgres" dbName = "db_test" port = "5437" dsn = "host=%s port=%s user=%s password=%s dbname=%s sslmode=disable timezone=UTC connect_timeout=30" ) var resource *dockertest.Resource var pool *dockertest.Pool var testDB *sqlx.DB var testRepo Repo func TestMain(m *testing.M) { // connect to docker; fail if docker not running p, err := dockertest.NewPool("") if err != nil { log.Fatalf("could not connect to docker; is it running? %s", err) } pool = p opts := dockertest.RunOptions{ Repository: "postgres", Tag: "14.5", // same as docker compose Env: []string{ "POSTGRES_USER=" + user, "POSTGRES_PASSWORD=" + password, "POSTGRES_DB=" + dbName, }, ExposedPorts: []string{"5432"}, PortBindings: map[docker.Port][]docker.PortBinding{ "5432": { {HostIP: "0.0.0.0", HostPort: port}, }, }, } resource, err = pool.RunWithOptions(&opts) if err != nil { // _ = pool.Purge(resource) log.Fatalf("could not start resource: %s", err) } if err := pool.Retry(func() error { var err error testDB, err = sqlx.Connect("postgres", fmt.Sprintf(dsn, host, port, user, password, dbName)) if err != nil { log.Println("Error:", err) return err } return testDB.Ping() }); err != nil { _ = pool.Purge(resource) log.Fatalf("could not connect to database: %s", err) } err = createTables() if err != nil { log.Fatalf("error creating tables: %s", err) } code := m.Run() if err := pool.Purge(resource); err != nil { log.Fatalf("could not purge resource: %s", err) } testRepo = &repo{db: testDB} os.Exit(code) } func createTables() error { tableSQL, err := os.ReadFile("./testdata/tables.sql") if err != nil { fmt.Println(err) return err } _, err = testDB.Exec(string(tableSQL)) if err != nil { fmt.Println(err) return err } return nil } func Test_pingDB(t *testing.T) { err := testDB.Ping() if err != nil { t.Error("can't ping database") } }
Correct answer
The default maximum wait time for pool.Retry
is one minute一个>. Just a guess, maybe your postgres database container won't start within a minute.
Try to increase the MaxWait time, for example pool.MaxWait = 20 * time.Minute
The above is the detailed content of Basic setup for Golang SQL unit testing using dockertest. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


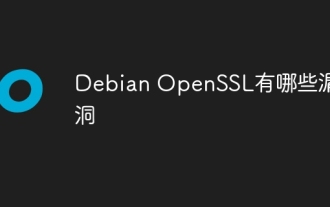
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
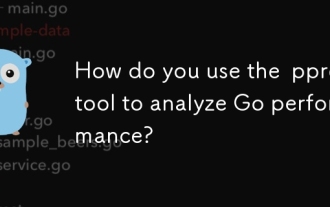
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
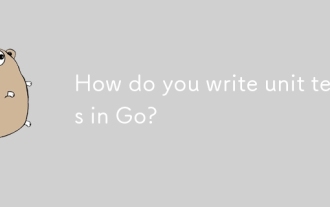
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
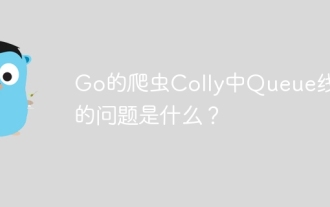
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
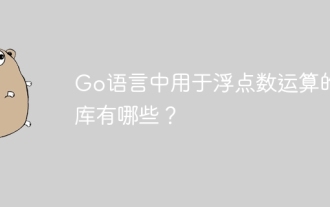
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
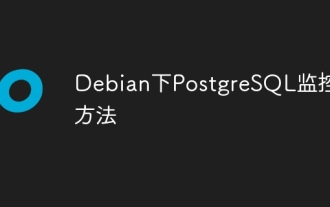
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
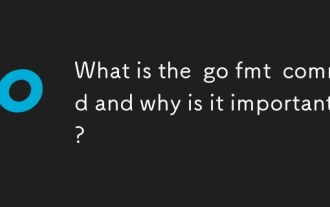
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
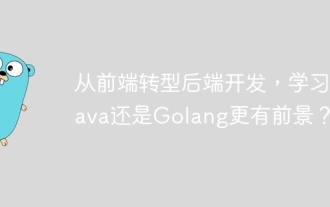
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
