Convert Chrome timestamps in Go
I'm trying to convert a timestamp from a local Chrome sqlite database to local time using Go. I know these timestamps are microseconds starting from 1601/01/01.
Checking the values of lastVisitTime
that I get in the following program on this Chrome Timestamp Conversion website, I seem to be retrieving them from the database correctly.
<code>package main import ( "database/sql" "fmt" "time" _ "github.com/mattn/go-sqlite3" "github.com/local_library/comp" ) var ( dbPath = comp.Expanduser("~/Library/Application Support/Google/Chrome/Default/History") chromeEpochStart = time.Date(1601, 1, 1, 0, 0, 0, 0, time.UTC) ) const ( driverName = "sqlite3" tmpPath = "/tmp/History" query = ` SELECT last_visit_time FROM urls ORDER BY last_visit_time DESC LIMIT 5 ` ) func main() { // Copy to tmp to unlock err := comp.Copy(dbPath, tmpPath) comp.MustBeNil(err) db, err := sql.Open(driverName, tmpPath) comp.MustBeNil(err) rows, err := db.Query(query) comp.MustBeNil(err) for rows.Next() { var lastVisitTime int64 rows.Scan(&lastVisitTime) d := time.Duration(time.Microsecond * time.Duration(lastVisitTime)) t := chromeEpochStart.Add(d) fmt.Println(t, lastVisitTime) } err = rows.Close() comp.MustBeNil(err) err = rows.Err() comp.MustBeNil(err) } </code>
But for some reason my .Add(d)
sets the time to 1601 before, which I've never seen before.
1439-07-05 20:00:21.462742384 +0000 UTC 13350512095172294 1439-07-05 19:58:20.377916384 +0000 UTC 13350511974087468 1439-07-05 19:57:58.539932384 +0000 UTC 13350511952249484 1439-07-05 19:57:48.539540384 +0000 UTC 13350511942249092 1439-07-05 19:52:09.587445384 +0000 UTC 13350511603296997
What's going on here, and more importantly, how do I do this correctly?
Correct answer
Thanks Peter's comment pointed out the overflow in my original code and I found a different approach.
If you convert the Chrome start date to UnixMicro()
, you get a negative offset from the regular epoch time:
<code>chromeMicroOffset := time.Date(1601, 1, 1, 0, 0, 0, 0, time.UTC).UnixMicro() fmt.Println(chromeMicroOffset) </code>
-11644473600000000
Add these to the database values and convert them to nanoseconds in time.Unix()
to get the correct UTC time:
<code>microFromEpoch := chromeMicroOffset + lastVisitTime t := time.Unix(0, microFromEpoch*1000) </code>
Full example of conversion to Pacific Time:
<code>package main import ( "fmt" "time" ) var ( chromeMicroOffset = time.Date(1601, 1, 1, 0, 0, 0, 0, time.UTC).UnixMicro() ) func main() { chromeTimestamp := int64(13350516239099543) microFromEpoch := chromeMicroOffset + chromeTimestamp t := time.Unix(0, microFromEpoch*1000) loc, err := time.LoadLocation("America/Los_Angeles") if err != nil { panic(err) } fmt.Println(t.In(loc)) } </code>
2024-01-23 12:43:59.099543 -0800 PST
The above is the detailed content of Convert Chrome timestamps in Go. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



Since its inception in 2009, Bitcoin has become a leader in the cryptocurrency world and its price has experienced huge fluctuations. To provide a comprehensive historical overview, this article compiles Bitcoin price data from 2009 to 2025, covering major market events, changes in market sentiment, and important factors influencing price movements.
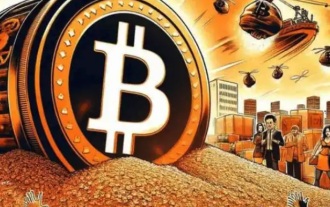
Bitcoin, as a cryptocurrency, has experienced significant market volatility since its inception. This article will provide an overview of the historical price of Bitcoin since its birth to help readers understand its price trends and key moments. By analyzing Bitcoin's historical price data, we can understand the market's assessment of its value, factors affecting its fluctuations, and provide a basis for future investment decisions.
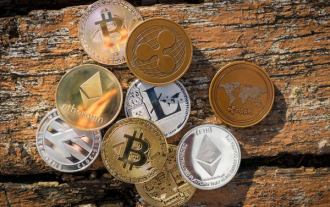
Since its creation in 2009, Bitcoin’s price has experienced several major fluctuations, rising to $69,044.77 in November 2021 and falling to $3,191.22 in December 2018. As of December 2024, the latest price has exceeded $100,204.
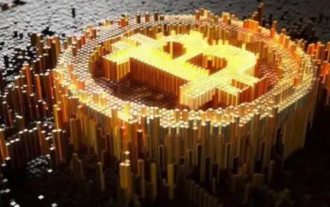
Real-time Bitcoin USD Price Factors that affect Bitcoin price Indicators for predicting future Bitcoin prices Here are some key information about the price of Bitcoin in 2018-2024:
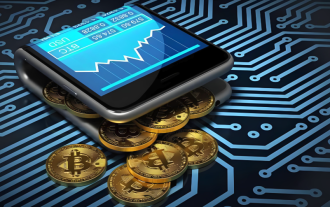
Important Node for Bitcoin Historical Price January 3, 2009: Genesis Block was generated, the first Bitcoin was generated, with a value of USD 0. October 5: The first Bitcoin transaction, a programmer bought two pizzas with 10,000 bitcoins, equivalent to $0.008. February 9, 2010: The Mt. Gox exchange went online and became the main platform for early Bitcoin trading. May 22: Bitcoin breaks through $1 for the first time. July 17: Bitcoin price plunged to $0.008, hitting an all-time low. February 9, 2011: Bitcoin price breaks through $10 for the first time. April 10: Mt. Go
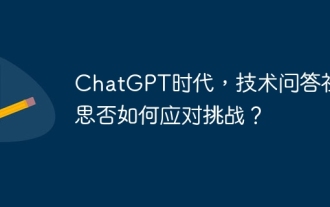
The technical Q&A community in the ChatGPT era: SegmentFault’s response strategy StackOverflow...
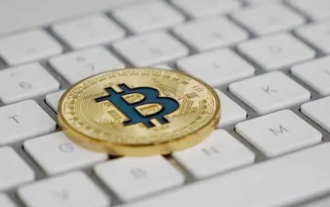
Virtual currency funding rates are fees charged to traders holding positions in derivatives trading. It reflects a premium or discount between the spot market price and the futures contract price when the contract expires. When the spot price is higher than the futures price, the capital rate is negative, which means that traders who short positions pay fees to traders who long positions. On the contrary, when the spot price is lower than the futures price, the capital rate is positive, which means that traders who do long positions pay fees to traders who do short positions.
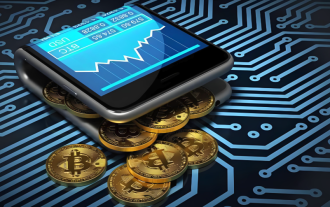
The virtual currency market is constantly evolving and exciting growth is expected in the coming years. In 2025, some cryptocurrencies are expected to stand out and become the most promising investments in the space. This article analyzes some of the most promising virtual currencies in 2025, covering their unique capabilities, growth potential and possibilities that impact the future. These currencies include Ethereum, Bitcoin, Cardano, Polkadot and Binance Coin, which play a key role in the development of decentralized finance, smart contracts and blockchain technologies. Understanding the potential of these virtual currencies, investors can be prepared to seize the opportunities brought by the virtual currency market in 2025.
