Why can't I see the output if I don't sleep at the end?
The following code implements the use of two goroutines to alternately print elements in the linked list. However, it suffers from a rather strange problem where the printed result is not visible without the final time. sleep. Theoretically, stdout has no buffer. Can anyone provide some guidance?
import ( "context" "fmt" "sync" ) type ListNode struct { val int next *ListNode } func NewLinkedList() (head *ListNode) { var cur *ListNode for i := 0; i < 100; i++ { if cur == nil { cur = &ListNode{val: i} head = cur } else { cur.next = &ListNode{val: i} cur = cur.next } } return } func main() { ll := NewLinkedList() wg := sync.WaitGroup{} var a = make(chan *ListNode, 1) var b = make(chan *ListNode, 1) ctx, cancel := context.WithCancel(context.Background()) worker := func(name string, input, output chan *ListNode) { wg.Add(1) defer wg.Done() for { select { case n := <-input: if n == nil { break } fmt.Printf("%s: %d\n", name, n.val) if n.next != nil { output <- n.next } else { cancel() break } case <-ctx.Done(): break } } } go worker("a", a, b) go worker("b", b, a) a <- ll wg.Wait() //time.Sleep(time.Millisecond) }
Correct answer
You must call wg.Add(1)
on the main Goroutine because the waitgroup counter is incremented before the 2 started Goroutinesmain ()
Reaching wg.Wait()
is a valid scenario. If its counter is 0, wg.Wait()
does not block, main()
returns, and therefore the entire application terminates:
wg.Add(1) go worker("a", a, b) wg.Add(1) go worker("a", a, b)
(of course, starting from Remove wg.Add(1)
from staff.)
The above is the detailed content of Why can't I see the output if I don't sleep at the end?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Since its inception in 2009, Bitcoin has become a leader in the cryptocurrency world and its price has experienced huge fluctuations. To provide a comprehensive historical overview, this article compiles Bitcoin price data from 2009 to 2025, covering major market events, changes in market sentiment, and important factors influencing price movements.
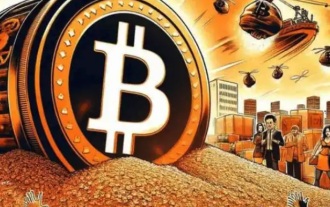
Bitcoin, as a cryptocurrency, has experienced significant market volatility since its inception. This article will provide an overview of the historical price of Bitcoin since its birth to help readers understand its price trends and key moments. By analyzing Bitcoin's historical price data, we can understand the market's assessment of its value, factors affecting its fluctuations, and provide a basis for future investment decisions.
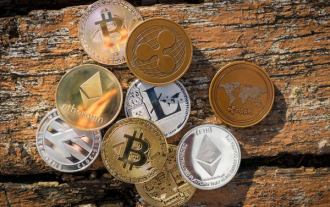
Since its creation in 2009, Bitcoin’s price has experienced several major fluctuations, rising to $69,044.77 in November 2021 and falling to $3,191.22 in December 2018. As of December 2024, the latest price has exceeded $100,204.
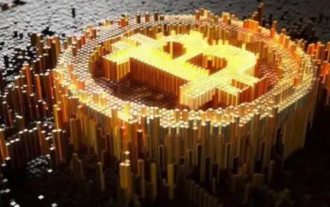
Real-time Bitcoin USD Price Factors that affect Bitcoin price Indicators for predicting future Bitcoin prices Here are some key information about the price of Bitcoin in 2018-2024:
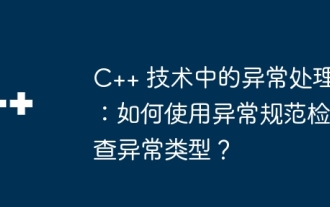
Exception specifications in C++ can specify the types of exceptions that may be thrown by a function to ensure correct handling of exceptions. To use exception specifications, use the noexcept keyword in a function declaration, followed by a list of exception types. For example, in the divide function, use noexcept(std::invalid_argument) to specify that only invalid_argument exceptions may be thrown, ensuring that other exception types will cause compiler errors.
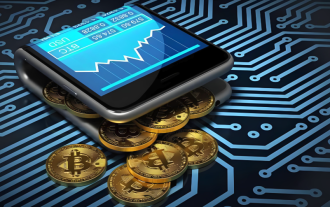
Important Node for Bitcoin Historical Price January 3, 2009: Genesis Block was generated, the first Bitcoin was generated, with a value of USD 0. October 5: The first Bitcoin transaction, a programmer bought two pizzas with 10,000 bitcoins, equivalent to $0.008. February 9, 2010: The Mt. Gox exchange went online and became the main platform for early Bitcoin trading. May 22: Bitcoin breaks through $1 for the first time. July 17: Bitcoin price plunged to $0.008, hitting an all-time low. February 9, 2011: Bitcoin price breaks through $10 for the first time. April 10: Mt. Go
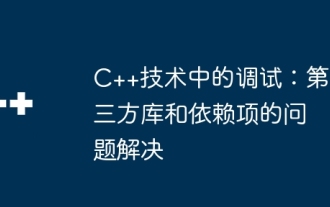
In C++ debugging, the solution to the third-party library dependency problem is as follows: verify that the dependency exists and is installed correctly; check whether the link flag is correctly specified; use the -L option to specify the library path; consider using dynamic linking; update the compiler version to resolve the dependency Compatibility issues; use a debugger to inspect the code line by line; check log files to understand the source of errors; update third-party libraries to the latest version; seek external support in the forum or contact the library maintainer.
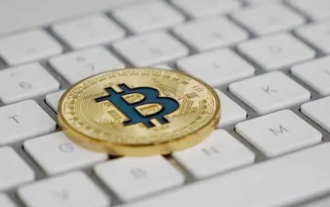
Virtual currency funding rates are fees charged to traders holding positions in derivatives trading. It reflects a premium or discount between the spot market price and the futures contract price when the contract expires. When the spot price is higher than the futures price, the capital rate is negative, which means that traders who short positions pay fees to traders who long positions. On the contrary, when the spot price is lower than the futures price, the capital rate is positive, which means that traders who do long positions pay fees to traders who do short positions.
