


Running the executable on the command line is fine, but running it through another program results in unresponsiveness
Just run the executable file and parameters in the Windows command prompt:
cgx_STATIC.exe -b C:\Users\m3\AppData\Local\Temp\shot-277325955.fbd
However, when running the same executable through Golang, the executable becomes unresponsive after the executable creates some output files.
<code> // Run an executable and print its log into a file. func RunWithLogFile(pthExe string, arg []string, fLog *os.File) error { cmd := exec.Command(pthExe, arg...) stdout, err := cmd.StdoutPipe() if err != nil { return err } stderr, err := cmd.StderrPipe() if err != nil { return err } err = cmd.Start() if err != nil { return err } // Stream logs: // https://stackoverflow.com/a/48849811/3405291 scannerOut := bufio.NewScanner(stdout) scannerErr := bufio.NewScanner(stderr) scannerOut.Split(bufio.ScanRunes) scannerErr.Split(bufio.ScanRunes) for scannerOut.Scan() { _, err = fLog.WriteString(scannerOut.Text()) if err != nil { return err } } for scannerErr.Scan() { _, err = fLog.WriteString(scannerErr.Text()) if err != nil { return err } } if scannerOut.Err() != nil { return err } if scannerErr.Err() != nil { return err } err = cmd.Wait() return err } </code>
I'm wondering if there's some kind of bug in the Go code above or it's not suitable for running an executable?
Single goroutine
Following @BurakSerdar's suggestion, I read from stdout and stderr in separate goroutines, but the problem was not solved:
<code> // Run an executable and print its log into a file. func RunWithLogFile(pthExe string, arg []string, fLog *os.File) error { cmd := exec.Command(pthExe, arg...) stdout, err := cmd.StdoutPipe() if err != nil { return err } stderr, err := cmd.StderrPipe() if err != nil { return err } var wg sync.WaitGroup wg.Add(2) go streamToLogFile(stdout, fLog, &wg) go streamToLogFile(stderr, fLog, &wg) err = cmd.Start() if err != nil { return err } wg.Wait() err = cmd.Wait() return err } func streamToLogFile(output io.ReadCloser, fLog *os.File, wg *sync.WaitGroup) { defer wg.Done() scanner := bufio.NewScanner(output) scanner.Split(bufio.ScanRunes) for scanner.Scan() { _, err := fLog.WriteString(scanner.Text()) if err != nil { log.Printf("error: write to log file: %s", err.Error()) } } err := scanner.Err() if err != nil { log.Printf("error: write to log file: %s", err.Error()) } } </code>
Correct answer
The unresponsiveness issue was solved by running the executable with administratorpermissions. I'm doing this:
A C# code runs a Go code and the Go code runs a C Code, i.e. external executable.
C executable calls some OpenGL GLUT calls. Maybe they need admin rights.
Launching Go code via C# solves this problem as follows: https://www.php.cn/link/ac90e5f00f7542d99231f63fb0dfeecf< /a>
<code> public static void RunLogic(string exePath, string args, PostProcess pp) { cmd = new Process(); try { cmd.StartInfo.FileName = exePath; cmd.StartInfo.Arguments = args; cmd.StartInfo.UseShellExecute = true; cmd.StartInfo.CreateNoWindow = false; cmd.StartInfo.RedirectStandardOutput = false; cmd.StartInfo.RedirectStandardError = false; cmd.StartInfo.RedirectStandardInput = false; // Vista or higher check. // https://www.php.cn/link/ac90e5f00f7542d99231f63fb0dfeecf if (System.Environment.OSVersion.Version.Major >= 6) { // Run with admin privileges to avoid a non-responsive executable. cmd.StartInfo.Verb = "runas"; } cmd.EnableRaisingEvents = true; cmd.Exited += new EventHandler(cmd_Exited); cmd.Exited += new EventHandler(pp); cmd.Start(); } catch (Exception ex) { RhinoApp.WriteLine("Error on process start: {0}", ex.Message); } } </code>
The above is the detailed content of Running the executable on the command line is fine, but running it through another program results in unresponsiveness. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


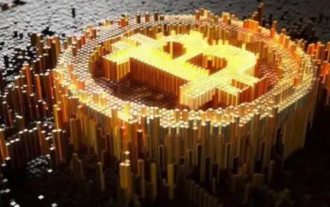
Real-time Bitcoin USD Price Factors that affect Bitcoin price Indicators for predicting future Bitcoin prices Here are some key information about the price of Bitcoin in 2018-2024:
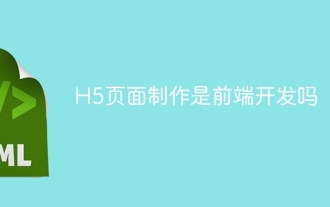
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
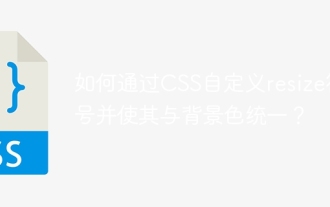
The method of customizing resize symbols in CSS is unified with background colors. In daily development, we often encounter situations where we need to customize user interface details, such as adjusting...
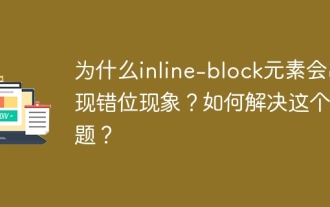
Regarding the reasons and solutions for misaligned display of inline-block elements. When writing web page layout, we often encounter some seemingly strange display problems. Compare...
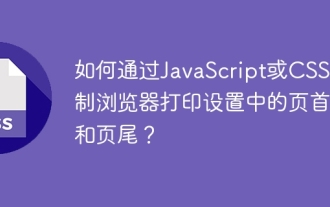
How to use JavaScript or CSS to control the top and end of the page in the browser's printing settings. In the browser's printing settings, there is an option to control whether the display is...
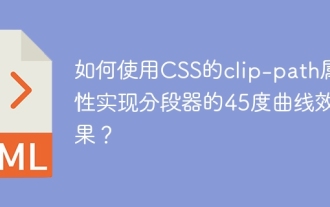
How to achieve the 45-degree curve effect of segmenter? In the process of implementing the segmenter, how to make the right border turn into a 45-degree curve when clicking the left button, and the point...
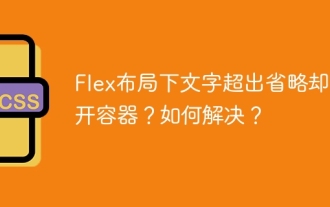
The problem of container opening due to excessive omission of text under Flex layout and solutions are used...
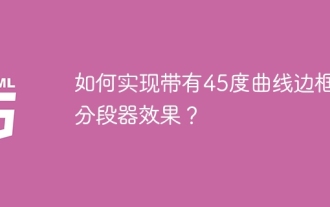
Tips for Implementing Segmenter Effects In user interface design, segmenter is a common navigation element, especially in mobile applications and responsive web pages. ...
