


How to convert map slice to structure slice with different properties
php editor Xigua introduces you how to convert mapping slices into structural slices with different attributes. In programming, we often encounter situations where we need to convert a map slice into a structure slice with different properties. This transformation helps us better organize and manage data. In this article, we'll introduce a simple yet effective way to implement this transformation, making your code more efficient and flexible. Let’s take a look!
Question content
I am using an api and I need to pass it a struct fragment. I have a map, so I need to convert it to a structure.
package main import "fmt" func main() { a := []map[string]interface{}{} b := make(map[string]interface{}) c := make(map[string]interface{}) b["prop1"] = "foo" b["prop2"] = "bar" a = append(a, b) c["prop3"] = "baz" c["prop4"] = "foobar" a = append(a, c) fmt.println(a) }
[map[prop1:foo prop2:bar] map[prop3:baz prop4:foobar]]
So in this example I have a slice of map a
which contains b
and c
which are maps of strings with different keys.
I wish to convert a
into a struct slice, where the first element is a struct with prop1
and prop2
as properties, and the second Each element is a structure with prop3
and prop4
as attributes.
is it possible?
I looked at https://github.com/mitchellh/mapstruct but I can't get it to work for my use case. I've seen this answer: https://stackoverflow.com/a/26746461/3390419
Explains how to use the library:
mapstructure.Decode(myData, &result)
However, this seems to assume that the structure where result
is an instance is predefined, whereas in my case the structure is dynamic.
Workaround
What you can do is first loop through each map individually, using each map's key-value pairs to build the corresponding reflect.structfield
value slice. Once you have such a slice ready, you can pass it to reflect.structof
which will return a reflect.type
value representing the dynamic struct type, and then You can pass this to reflect.new
to create a reflect.value
which will represent an instance of the dynamic structure (actually a pointer to the structure).
For example
var result []any for _, m := range a { fields := make([]reflect.StructField, 0, len(m)) for k, v := range m { f := reflect.StructField{ Name: k, Type: reflect.TypeOf(v), // allow for other types, not just strings } fields = append(fields, f) } st := reflect.StructOf(fields) // new struct type sv := reflect.New(st) // new struct value for k, v := range m { sv.Elem(). // dereference struct pointer FieldByName(k). // get the relevant field Set(reflect.ValueOf(v)) // set the value of the field } result = append(result, sv.Interface()) }
https://www.php.cn/link/3722e31eaa9efae6938cc5c435365dfd
The above is the detailed content of How to convert map slice to structure slice with different properties. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


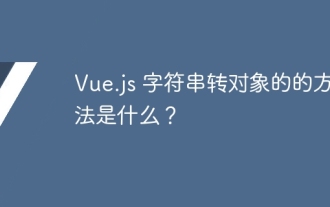
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.
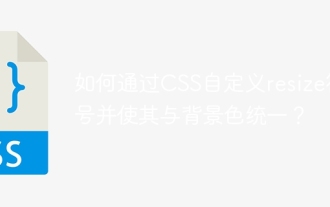
The method of customizing resize symbols in CSS is unified with background colors. In daily development, we often encounter situations where we need to customize user interface details, such as adjusting...
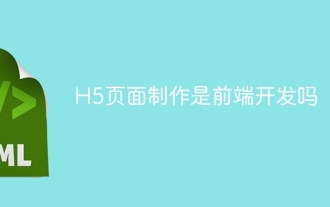
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
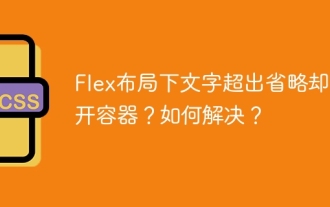
The problem of container opening due to excessive omission of text under Flex layout and solutions are used...
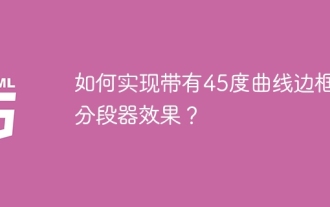
Tips for Implementing Segmenter Effects In user interface design, segmenter is a common navigation element, especially in mobile applications and responsive web pages. ...
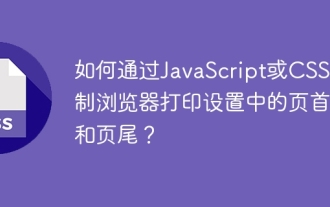
How to use JavaScript or CSS to control the top and end of the page in the browser's printing settings. In the browser's printing settings, there is an option to control whether the display is...
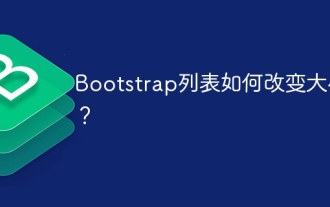
The size of a Bootstrap list depends on the size of the container that contains the list, not the list itself. Using Bootstrap's grid system or Flexbox can control the size of the container, thereby indirectly resizing the list items.
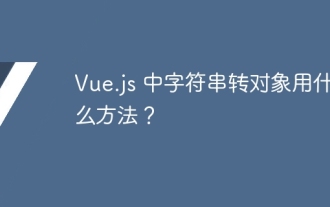
When converting strings to objects in Vue.js, JSON.parse() is preferred for standard JSON strings. For non-standard JSON strings, the string can be processed by using regular expressions and reduce methods according to the format or decoded URL-encoded. Select the appropriate method according to the string format and pay attention to security and encoding issues to avoid bugs.
