Reverse linked list in one row
php Xiaobian Yuzai introduces you to a common data structure algorithm - "Intra-row reverse linked list". In this algorithm, we need to reverse the order of nodes in a linked list. Through concise and efficient code implementation, we can complete this operation in one line, completely reversing the order of the linked list. This algorithm is very useful in actual programming and can play an important role in both data processing and algorithm design. Let’s learn about this wonderful algorithm together!
Question content
I just found the solution for reverse linked list using one line in go on leetcode. It does work, but I don't understand how to implement it.
That's it:
func reverselist(head *listnode) (prev *listnode) { for head != nil { prev, head, head.next = head, head.next, prev } return }
For example, let the list be [1->2->3->4->5->nil]
.
I know it works like this:
First execute
head.next = prev
(head.next = nil
, so nowhead = [1->nil]
)Then,
prev = head
(In this stepprev = [1->nil]
just likehead
in the previous step )head = head.next
This is magic. Forprev
in the second step of go, usehead = [1->nil]
, but after this stephead = [2->3-> ;4->5->nil]
So when head != nil
it iterates and in the second step prev = [2->1->nil]
, head = [3->4->5->nil]
and so on.
This line can be expressed as:
for head != nil { a := *head prev, a.Next = &a, prev head = head.Next }
Am I right? Why is this happening?
Solution
The variable on the left side of the expression will be assigned to the value on the right side of the expression at that time. This is a clever use of language.
To make it easier to understand, let’s look at an example.
set up
This is our link list: 1 -> 2 -> 3 -> 4 -> None
Before the function is executed,
- The head is *node 1
- prev is zero (uninitialized)
- head.next is *node 2
Step by step
prev, head, head.next = head, head.next, prev
Let’s break it down,
- prev (nil) = head (*node 1)
- head (*Node 1) = head.next (*Node 2)
- head.next (*node 2) = prev (nil)
Next iteration,
- prev (*node 1) = head (*node 2)
- head (*Node 2) = head.next (*Node 3)
- head.next (*node 3) = prev (*node 1)
Summary
Basically, it reverses head.next
to the previous node and moves prev and head to the next node.
Compare this to a textbook algorithm in go to be clear:
func reverseList(head *ListNode) *ListNode { var prev *ListNode for head != nil { nextTemp := head.Next head.Next = prev prev = head head = nextTemp } return prev }
The above is the detailed content of Reverse linked list in one row. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


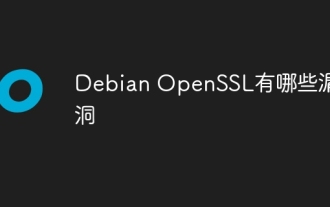
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
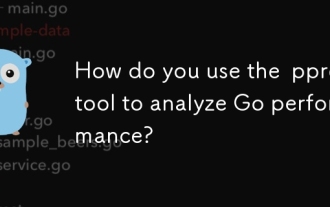
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
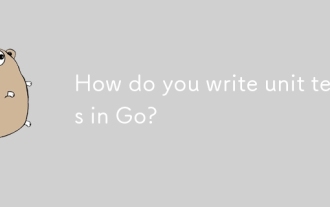
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
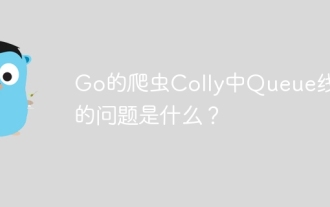
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
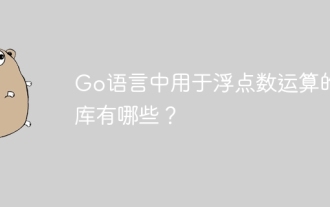
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
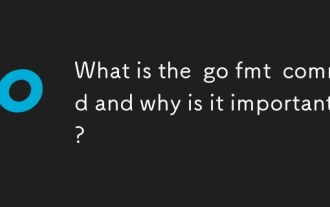
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
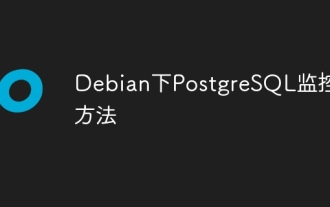
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
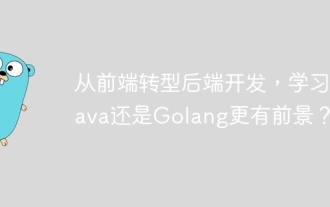
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
