Lock the map for concurrent access to the map
Feb 09, 2024 am 11:06 AMphp editor Baicao is here to introduce to you a very useful technique, which is to lock the map for concurrent access. This technique can help developers avoid conflicts and data errors when accessing the map concurrently. By using a locking mechanism, developers can ensure that each request is made in order and that no data clutter occurs. This is very important for developing map operations, especially when multiple users are accessing the map at the same time. Let’s take a look at how to implement this technique!
Question content
I have a map: map[string]map[string]*Struct and I need to read/write it in multiple Go routines.
What is the best way to achieve this? Mutex or RWMutex? And where to place it?
If I use RWMutex, should I Lock or RLock before performing operations involving reading and writing?
I tried using rwmutex in the root map, but I'm not sure if that's the best way to solve this problem.
I also tried "locking" before reading and writing, but sometimes I get "concurrent writes" panics.
Solution
You can use RWLock. If the operation involves writing (whether it is reading or writing only), you need to use Lock, if it only involves reading, RLock/RUnlock.
Lock can also be considered an exclusive lock. RLock, on the other hand, is non-exclusive. An RLock can be acquired even if the RWMutex is locked for reading, but goroutine execution will be blocked if the resource is locked exclusively by the Lock method:
1 |
|
On the other hand, the Lock method blocks goroutine execution until all readers and writers unlock the resource (using the RUnlock/Unlock method). Lock is exclusive because only one goroutine can access the resource (whether reading or writing) until the Unlock method is called.
Typical method:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 |
|
The above is the detailed content of Lock the map for concurrent access to the map. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
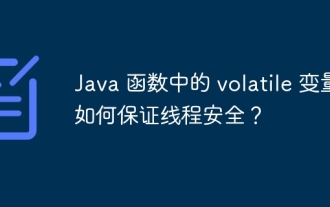
How to ensure thread safety of volatile variables in Java functions?
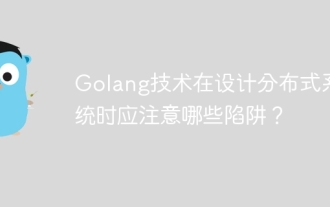
What pitfalls should we pay attention to when designing distributed systems with Golang technology?
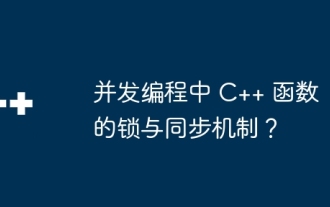
Locking and synchronization mechanism of C++ functions in concurrent programming?
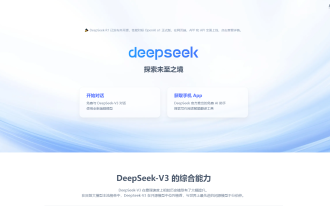
How to solve the problem of busy servers for deepseek
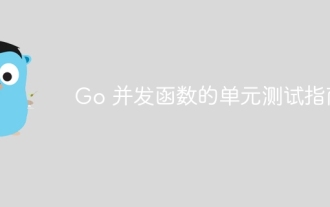
A guide to unit testing Go concurrent functions
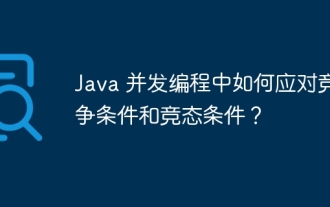
How to deal with race conditions and race conditions in Java concurrent programming?
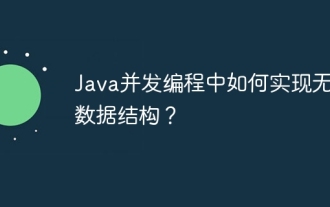
How to implement lock-free data structures in Java concurrent programming?
