Foreign keys in GORM not updated
When I was using GORM recently, I found a problem - the foreign key was not updated. When I updated the value of the foreign key field in the related table, it was not updated synchronously to the other table. Through investigation and research, I found that this is because GORM does not automatically update foreign key fields by default. This problem has been bothering me for a while, so I decided to share the solution with everyone. In this article, I will show you how to use GORM to correctly update foreign key fields to avoid this problem.
Question content
I have two desks, one is the company
type Company struct { Id uuid.UUID `gorm:"column:id;primaryKey;"` CreatedAt time.Time `gorm:"index;column:createdAt"` UpdatedAt time.Time `gorm:"index;column:updatedAt"` Name string `gorm:"column:name" binding:"required"` }
The other is product_entitlement
type ProductEntitlement struct { ID uuid.UUID CreatedAt time.Time `gorm:"index;column:createdAt"` UpdatedAt time.Time `gorm:"index;column:updatedAt"` Type string `gorm:"column:type" binding:"required"` CompanyID uuid.UUID `gorm:"column:companyId;size:36"` Company Company `gorm:"foreignKey:CompanyID"` }
CompanyID is a foreign key. CompanyID contains the value from Company.Id
After the update insert is completed, new rows will be inserted every time. This is the code we are using
func UpdateEntitlement(c *gin.Context) { cid := c.Param("companyId") id := c.Param("entitlementId") eid := uuid.MustParse(id) log.Println(eid) uid := uuid.MustParse(cid) log.Println(uid) var entitlementRequest entities.ProductEntitlement if err := c.BindJSON(&entitlementRequest); err != nil { log.Println(err) fmt.Println("ERROR: ", err) c.JSON(400, gin.H{"error": "Invalid JSON format"}) return } if err := database.DB.Clauses(clause.OnConflict{ Columns: []clause.Column{{Name: "id"}}, UpdateAll: true, }).Create(&entitlementRequest).Error; err != nil { log.Println(err) } }
But it always fails and gives error
BD820BD3F94A2A45E18ED8E8094EF395I want to update Product_entitlement if the ID exists, else create a new one
The URL is as follows, http://localhost:8080/company/{{companyId}}/product/{{companyId}} and use the PUT method The body is
{"Type": "Automatic"}
Workaround
If it helps someone, we can use the FirstOrCreate
function to check if the id exists and updates it, or creates a new one if it doesn't exist.
To assign a foreign key, we need to assign the value to the related table,
entitlementRequest.CompanyID = uid
func UpdateEntitlement(c *gin.Context) { cid := c.Param("companyId") uid := uuid.MustParse(cid) eid := c.Param("entitlementId") nid := uuid.MustParse(eid) var entitlementRequest entities.ProductEntitlement entitlementRequest.CompanyID = uid if err := c.BindJSON(&entitlementRequest); err != nil { fmt.Println("ERROR: ", err) c.JSON(400, gin.H{"error": "Invalid JSON format"}) return } if err := database.DB.Where("id = ?", nid). Assign(entitlementRequest). FirstOrCreate(&entitlementRequest).Error; err != nil { c.JSON(http.StatusBadRequest, gin.H{"error": "Record not found!"}) return } c.JSON(http.StatusOK, gin.H{"error": "Product entitlement upserted successfully"}) }
The above is the detailed content of Foreign keys in GORM not updated. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


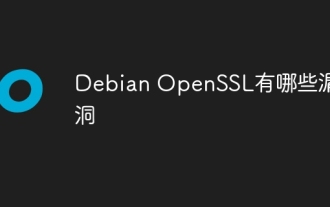
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
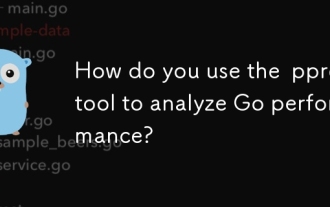
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
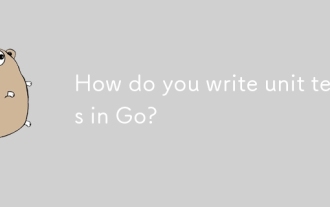
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
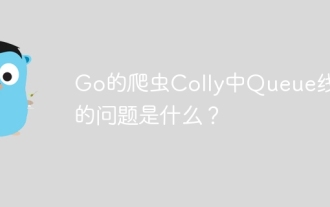
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
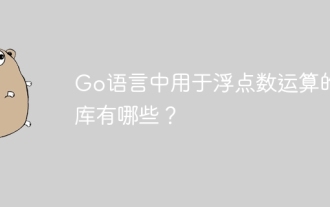
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
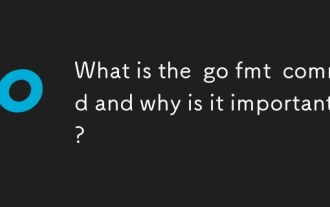
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
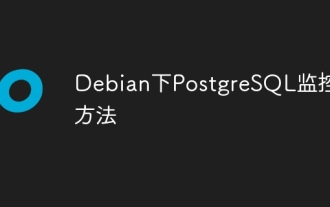
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
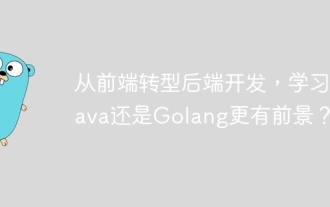
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
