


Kivy screen manager kivy\properties.pyx KeyError and AttributeError: 'super' object has no attribute '__getattr__'. Did you mean: '__setattr__'?
I ran into a problem while trying to implement a screen manager using kivy. As you can see the "chat" id is defined in main.kv so I can't understand why the error occurs. I'm new to kivy and have never used a screen manager before.. please help!
app_cleaned.py
from kivy.app import app from kivy.lang import builder from kivy.uix.label import label from kivy.core.window import window from kivy.uix.screenmanager import screen, screenmanager from kivy.clock import mainthread from range_key_dict import rangekeydict from math import inf from threading import thread from powerbot import chatbot #remove from kivy.clock import clock window.size = (500, 500) class signupscreen(screen): pass class mainscreen(screen): pass class message(label): pass class user: def __init__(self, years_lifting, weight, height, unavailable_equipment, unavailable_muscle_groups, aim): # lifting experience classification expclassdict = rangekeydict({ (0.0, 1.9): 1, # beginner (2.0, 3.9): 2, # intermediate (4, inf): 3 # advanced }) expclass = expclassdict[years_lifting] # bmi classification bmiclassdict = rangekeydict({ (0.0, 9.9): 1, # severely underweight (10.0, 18.5): 2, # underweight (18.6, 24.9): 3, # healthy weight (25.0, 34.9): 2, # overweight (35.0, inf): 1, # severely overweight }) bmi = weight/(height/100)**2 # calculate bmi bmiclass = bmiclassdict[bmi] self.experience_level = expclass self.bmi_level = bmiclass self.unavailable_equipment = unavailable_equipment self.unavailable_muscle_groups = unavailable_muscle_groups self.aim = aim class exampleapp(app): def build(self): sm = screenmanager() # load the signup.kv file and add its content to the signupscreen builder.load_file('signup.kv') # create the signupscreen instance and add it to the screenmanager signup_screen = signupscreen(name='signup') sm.add_widget(signup_screen) # load the main application screen from main.kv builder.load_file('main.kv') main_screen = mainscreen(name='main') sm.add_widget(main_screen) return sm def switch_to_main_screen(self): self.root.current = 'main' def __init__(self, **kwargs): super().__init__(**kwargs) self.ai = chatbot("powerbot") def on_start(self): print("root ids:", self.root.ids) # assuming you expect 'chat' to be available here, you can print its contents as well if 'chat' in self.root.ids: print("chat widget:", self.root.ids.chat) self.root.current = 'signup' initial_messages = ["powerbot is initializing, please wait (this could take a minute)"] for message in initial_messages: self.system_message(message) message = "000000" thread = thread(target=self.background_message_receiver,args=(message,)) thread.start() self.root.ids.sv.scroll_y = 0 def sign_up(self, years_lifting, weight, height): # convert input values to appropriate data types (e.g., int, float) years_lifting = float(years_lifting) weight = float(weight) height = float(height) # create a user object with the provided sign-up details user = user(years_lifting, weight, height) # pass other sign-up details as needed self.root.ids.years_lifting_input.text = '' self.root.ids.weight_input.text = '' self.root.ids.height_input.text = '' def background_message_receiver(self, message): response = self.ai.message_to_bot(message) self.incoming_message(response) def send_message(self, message): self.root.ids.ti.text = "" if message: m = message(text=f"[color=dd2020]you[/color] > {message}") self.root.ids.chat.add_widget(m) self.root.ids.ti.focus = true thread = thread(target=self.background_message_receiver,args=(message,)) thread.start() @mainthread def incoming_message(self, message): m = message(text=f"[color=20dd20]powerbot[/color] > {message}") self.root.ids.chat.add_widget(m) self.root.ids.ti.focus = true def system_message(self, message): m = message(text=f"[color=ffffff]system[/color] > {message}") self.root.ids.chat.add_widget(m) self.root.ids.ti.focus = true # execute if __name__ == '__main__': exampleapp().run()
main.kv
<message>: size_hint: 1, none text_size: self.width, none size: self.texture_size markup: true <mainscreen>: boxlayout: orientation: 'vertical' padding: 10 scrollview: id: sv boxlayout: id: chat # add the id for the chat messages spacing: 5 padding: 10 orientation: 'vertical' size_hint_y: none height: self.minimum_height widget: # used as a spacer, push message to bottom size_hint_y: none height: sv.height boxlayout: size_hint_y: none height: 40 spacing: 10 textinput: id: ti multiline: false on_text_validate: app.send_message(self.text) button: text: 'submit' size_hint_x: none width: 75 on_release: app.send_message(ti.text)
Register.kv
<SignupScreen>: BoxLayout: orientation: 'vertical' padding: 10 Button: text: 'Continue' size_hint_y: None height: '48dp' on_release: app.switch_to_main_screen()
I've seen other questions here about similar problems, but unfortunately I'm not proficient enough with kivy to apply the solution to my specific scenario.
Correct answer
Your chat
id is defined in the mainscreen
class, but you are trying to Access it in self.root.ids
of #exampleapp. Since the id is defined in the
rule, you must access it through the
mainscreen instance. One way is to just save a reference to the
mainscreen instance. In the
build() method you can change:
# load the main application screen from main.kv
builder.load_file('main.kv')
main_screen = mainscreen(name='main')
sm.add_widget(main_screen)
# load the main application screen from main.kv builder.load_file('main.kv') self.main_screen = mainscreen(name='main') sm.add_widget(self.main_screen)
exampleapp method, you can access the
chat id like this:
self.main_screen.ids.chat
The above is the detailed content of Kivy screen manager kivy\properties.pyx KeyError and AttributeError: 'super' object has no attribute '__getattr__'. Did you mean: '__setattr__'?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


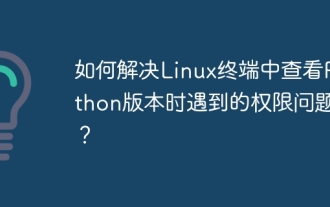
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
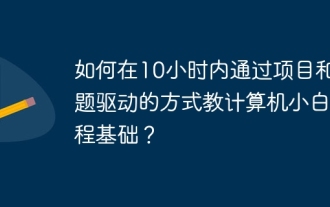
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
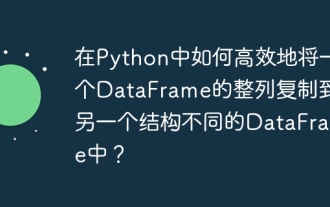
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
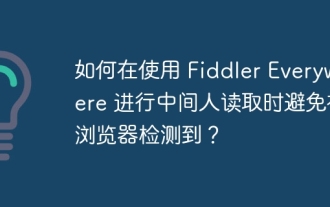
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
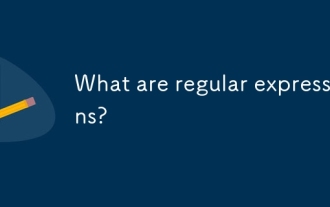
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
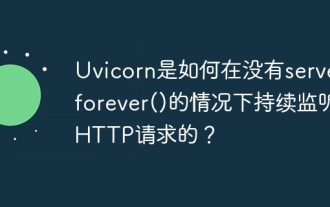
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
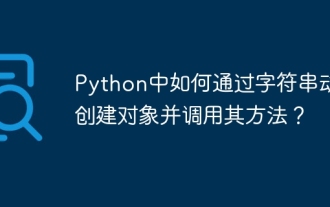
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
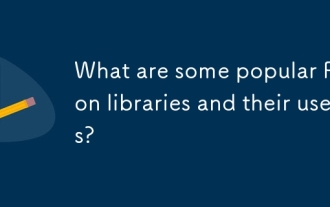
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
