CRUD with Postgres and MongoDB?
php Editor Xigua will take you through this article to learn how to use Postgres and MongoDB for CRUD operations. Postgres is a relational database, while MongoDB is a document database. CRUD operations refer to the process of creating (Create), reading (Read), updating (Update) and deleting (Delete) data. By combining these two different types of databases, you can choose the most suitable database for data operations based on different needs to improve efficiency and flexibility. Next, let us explore the application of these two databases in CRUD operations!
Question content
I am fairly new to Go and backends, and am participating in the Go internship program. We built a CRUD that connects to a psql database and now I'm told to connect to mongoDB, which we will use for development and PSQL will be used for production. Do I have to create a new handler for mongo from scratch or can I use the same handler and somehow determine the type of database being used and use the logic accordingly? For example, I have a handler for the user registration endpoint:
func (ctrl *UserController) Register(c *gin.Context) { var user models.User if err := c.BindJSON(&user); err != nil { c.AbortWithStatusJSON(http.StatusUnprocessableEntity, gin.H{ "error": true, "message": err.Error(), }) return } err := ctrl.userService.Register(&user) if err != nil { c.AbortWithStatusJSON(http.StatusUnprocessableEntity, gin.H{ "error": true, "message": err.Error(), }) return } c.JSON(http.StatusCreated, gin.H{ "message": "successfully created an user", }) } func (svc *UserService) Register(user *models.User) error { if svc.userRepo.CheckIfEmailExists(user.Email) { return errors.New("user already registered") } hash, err := svc.generatePasswordHash(user.Password) if err != nil { return errors.New("err can't register user") } user.Password = hash return svc.userRepo.Insert(user) } func (repo *UserRepository) CheckIfEmailExists(mail string) bool { var user models.User err := repo.dbClient.Debug().Model(models.User{}).Where("email = ?", mail).Find(&user).Error return errors.Is(err, gorm.ErrRecordNotFound) } func (repo *UserRepository) Insert(user *models.User) error { err := repo.dbClient.Debug().Model(models.User{}).Create(user).Error if err != nil { log.Printf("failed to insert user: %v\n", err) return err } return nil }
I built the insert, register and CheckIfEmailExists functions for mongo:
func (repo *UserRepository) InsertInMongo(user *models.UserB) error { coll := repo.mongoClient.DB.Collection("users") _, err := coll.InsertOne(context.TODO(), user) if err != nil { log.Printf("failed to insert user: %v\n", err) return err } return nil } func (svc *UserService) RegisterToMongo(user *models.UserB) error { check := svc.userRepo.CheckIfEmailExistsInMongo(user.Email) if check { return errors.New("user already registered") } hash, err := svc.generatePasswordHash(user.Password) if err != nil { return errors.New("err can't register user") } user.Password = hash return svc.userRepo.InsertInMongo(user) } func (repo *UserRepository) CheckIfEmailExistsInMongo(email string) bool { coll := repo.mongoClient.Collection filter := bson.D{{Key: "email", Value: email}} count, err := coll.CountDocuments(context.TODO(), filter) if err != nil { panic(err) } if count != 0 { return true } return false }
Solution
You did not provide code on how to create UserService.
Ideally, you should have an interface that looks like this:
type UserRepository interface { CheckIfEmailExists(mail string) bool Insert(user *models.User) error … }
Then, the UserService should be created like this:
// We use the interface as param func NewUserService(userRepo UserRepository) UserService { return UserService{ UserRepo: userRepo } }
And you will have two separate repositories, both implementing the UserRepository
interface - this means they should have methods with the same name and same signature (parameters, return type) as the interface:
mongo_user_repository.go
Type MongoUserRepo struct { … } func (repo MongoUserRepo) CheckIfEmailExists(mail string) bool { … some mongo logic here } func (repo MongoUserRepo) Insert(user *models.User) error { … some mongo logic here }
postgres_user_repository.go
type PostgresUserRepo struct { … } func (repo PostgresUserRepo) CheckIfEmailExists(mail string) bool { … some postgres logic here } func (repo PostgresUserRepo) Insert(user *models.User) error { … some postgres logic here }
You can pass any of these depending on the use case, as in the (very bad) example below:
main.go
… var userService UserService if os.Getenv(“environment”) == “prod” { userService = NewUserService(postgreUserRepo) } else if os.Getenv(“environment”) == “dev” { userService = NewUserService(mongoUserRepo) }
The above is the detailed content of CRUD with Postgres and MongoDB?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


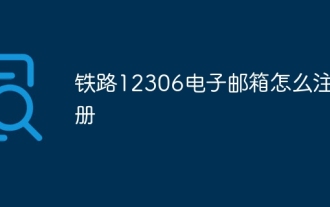
The steps to register the Railway 12306 email address are as follows: Visit the 12306 website and click "Register"; select "Email Registration" and fill in the email, name, mobile phone and other information; set the password and security questions according to the prompts; enter the email verification code and mobile phone verification code for verification Information; click "Complete Registration".
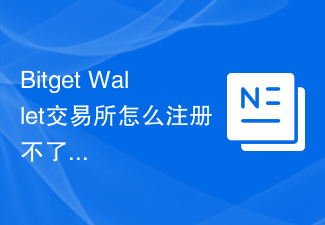
There are various reasons for being unable to register for the BitgetWallet exchange, including account restrictions, unsupported regions, network issues, system maintenance and technical failures. To register for the BitgetWallet exchange, please visit the official website, fill in the information, agree to the terms, complete registration and verify your identity.
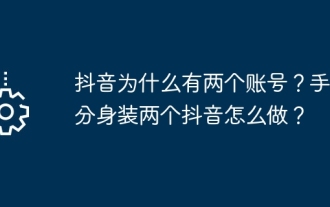
In the digital age, social media has become an integral part of people's lives. Douyin, as one of the most popular short video platforms in China, has attracted a large number of users. Some users even registered two accounts. So, why does Douyin have two accounts? This article will answer this question for you and explain how to install two Douyin accounts on your phone. 1. Why does Douyin have two accounts? Functional differentiation: Some users will differentiate accounts based on content type or function. For example, one account is used to share daily life, and another account is used to demonstrate professional skills. 2. Privacy protection: Some users hope to protect their privacy through two accounts, separate life and work, and avoid information leakage. 3. Interaction needs: Some users may register two due to interaction needs
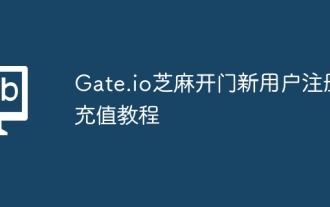
How to register and recharge Gate.io account: 1. Visit Gate.io official website and click "Register". 2. Select the registration method (email or mobile phone), set a password, and agree to the terms. 3. Log in to your account and click "Recharge". 4. Select legal currency or cryptocurrency to recharge, enter the amount, and select the recharge method. 5. Obtain the recharge address and transfer funds from external wallets or exchanges.

In order to enhance user interaction and improve user experience, the Douyin platform has launched Spark, an interesting interactive mechanism. Users can activate and upgrade their sparks through a series of actions on Douyin. Different colors represent different achievements and honors. Understanding the color changing rules of Douyin Spark can help users better participate and interact, and enjoy the social fun brought by Douyin. 1. What is the detailed explanation of Douyin’s spark color changing rules? 1. Behavior activates users’ interactive behaviors, such as likes, comments, shares, etc., which can activate sparks. 2. Level improvement As user interaction increases, the sparks will gradually upgrade and the color will change accordingly. 3. Color change The color change of sparks is usually related to the user's interaction frequency, interaction quality, and enthusiasm for participating in activities. 4. The task is completed

DeepSeek's official website is now launching multiple discount activities to provide users with a shopping experience. New users sign up to get a $10 coupon, and enjoy a 15% limited time discount for the entire audience. Recommend friends can also earn rewards, and you can accumulate points for redemption of gifts when shopping. The event deadlines are different. For details, please visit the DeepSeek official website for inquiries.
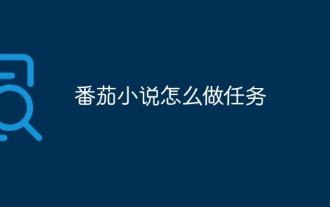
You can earn coins and points by completing tasks on Tomato Novels. Methods include: completing new user registration tasks. Check in daily. Read the assigned novel chapter. Leave a comment on the specified novel chapter. Invite friends to register. Share novels on social platforms.
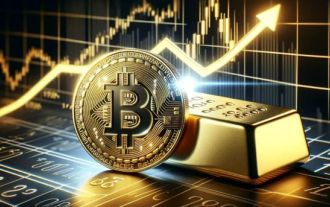
Gate.io Sesame Open is the world's leading blockchain digital asset trading platform, including fiat currency trading, currency trading, leveraged trading, perpetual contracts, ETF leveraged tokens, wealth management, Startup initial public offering and other sections, providing users with security, stability, openness and transparency.
