`docker system df` and `/system/df` (docker api endpoint)
php editor Yuzai is here to introduce to you two commands in docker: `docker system df` and `/system/df` (docker api endpoint). Both commands are used to view the docker system resource usage, but their usage and result display methods are slightly different. `docker system df` is a docker command that can be run directly in the terminal. It will display the usage of various resources in the docker system (including images, containers, data volumes, etc.), as well as the overall resource usage. And `/system/df` is a docker API endpoint, and you need to obtain relevant information by calling the API. Its return result is similar to `docker system df`, but it is more suitable for programmatically obtaining docker system resource usage.
Question content
I am writing a program in Go to get the total disk usage in GB from my docker host. For this I use func DiskUsage()
from go lib:
- https://pkg.go.dev/github.com/docker/docker/client#Client.DiskUsage.
View the code, the function is calling the docker api endpoint /system/df
:
- https://docs.docker.com/engine/api/v1.43/#tag/System/operation/SystemDataUsage
However, when I use this library with the calculation of GB using the command docker system df
, I notice a strange behavior:
-
docker system df
Output:$ docker system df TYPE TOTAL ACTIVE SIZE RECLAIMABLE Images 223 4 21.02GB 20.7GB (98%) Containers 6 0 0B 0B Local Volumes 13 1 536.4MB 340.4MB (63%) Build Cache 954 0 13.51GB 13.51GB
Copy after login - My Go application output:
$ go run ./cmd/main.go Images: TOTAL (223), 17GB Build Cache: TOTAL (954), 29GB
Copy after login
As you can see, there is a difference between the two outputs. I need help understanding if there is something wrong with my calculations that get the data from the /system/df
endpoint. Thanks:)
Go Application:
package main import ( "context" "fmt" "github.com/docker/docker/api/types" "github.com/docker/docker/client" ) func main() { cli, err := client.NewClientWithOpts(client.FromEnv) if err != nil { panic(err) } diskUsg, err := cli.DiskUsage(context.Background(), types.DiskUsageOptions{}) if err != nil { panic(err) } b := float64(0) for _, ch := range diskUsg.BuildCache { b = b + float64(ch.Size) } b2 := float64(0) for _, ch := range diskUsg.Images { if ch.Size > ch.SharedSize { b2 = b2 + (float64(ch.Size) - float64(ch.SharedSize)) continue } b2 = b2 + (float64(ch.SharedSize) - float64(ch.Size)) } fmt.Printf("Images: TOTAL (%d), %2.fGB\n", len(diskUsg.Images), float64(b2)/(1<<30)) fmt.Printf("Build Cache: TOTAL (%d), %2.fGB\n", len(diskUsg.BuildCache), float64(b)/(1<<30)) }
Solution
Based on Docker source code:
system df
Command: https://github.com/docker/cli/blob/v24.0.5/cli/command/system/df.go- Output format: https://github.com/docker/cli/blob/v24.0.5/cli/command/formatter/disk_usage.go
You should be able to reproduce exactly what docker system df
does using the following code:
go.mod
module 76982562-docker-system-df-vs-system-df-docker-api-endpoint go 1.21.0 require ( github.com/docker/cli v24.0.5+incompatible github.com/docker/docker v24.0.5+incompatible )
main.go
<code>package main import ( "context" "fmt" "os" "github.com/docker/cli/cli/command/formatter" "github.com/docker/docker/api/types" "github.com/docker/docker/client" "github.com/docker/go-units" ) func main() { cli, err := client.NewClientWithOpts(client.FromEnv) if err != nil { panic(err) } diskUsage, err := cli.DiskUsage(context.Background(), types.DiskUsageOptions{}) if err != nil { panic(err) } var bsz int64 for _, bc := range diskUsage.BuildCache { if !bc.Shared { bsz += bc.Size } } fmt.Printf("Images: TOTAL (%d), %s\n", len(diskUsage.Images), units.HumanSize(float64(diskUsage.LayersSize))) fmt.Printf("Build Cache: TOTAL (%d), %s\n", len(diskUsage.BuildCache), units.HumanSize(float64(bsz))) } </code>
- For images, the
docker
library directly providesdiskUsage.LayersSize
to represent the total size, so you don’t have to calculate it yourself - For the build cache you need to exclude shared projects (
if !bc.Shared
)
To convert the size in the correct units, I strongly recommend using github.com/docker/go-units
(e.g. units.HumanSize(float64(diskUsage.LayersSize))
) . This will save you the unit conversion nightmare!
The above is the detailed content of `docker system df` and `/system/df` (docker api endpoint). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


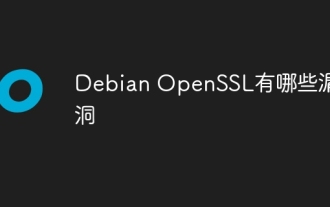
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
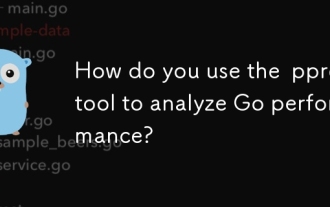
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
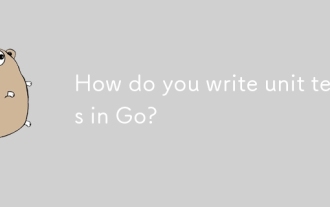
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
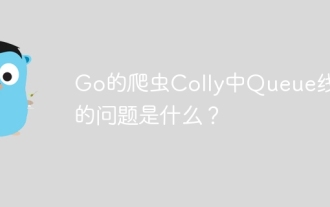
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
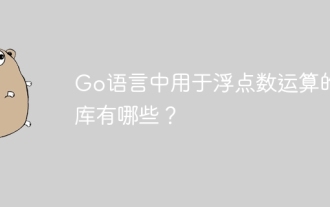
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
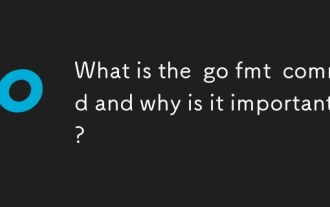
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
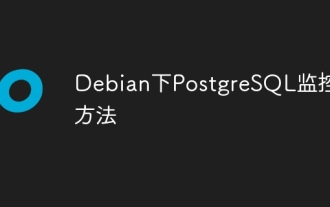
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
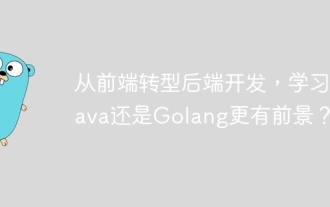
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
