


How can I define a generic function using a custom structure without listing all structures?
In PHP, if we want to use a custom structure to define a common function without having to list all the structures, there is an efficient way to do it. This approach is achieved by using a variable number of parameters. We can accept any number of parameters by using ellipses (...) as parameter names in the function definition. Within the function body, we can use the func_get_args() function to get all the arguments passed to the function. This way, we can use these parameters inside the function without defining all the structures in advance. This flexible approach can help us write more general and reusable functions and improve coding efficiency.
Question content
Suppose I have two different structures:
type one struct { id string // other fields } type two struct { id string // other fields }
Is it possible to define a function that accepts both one
and two
without explicitly listing them as options?
For example I'm looking for something like this:
type ModelWithId struct { Id string } func Test[M ModelWithId](m M) { fmt.PrintLn(m.Id) } one := One { Id: "1" } Test(one) // Prints 1
I don't want to use functest[m one | two](m m)
because I might have 10+ structures and I don't want to have to go back to that function every time I add a new structure to the code base .
Solution
Generics use methods to constrain type parameter behavior, so you need to rewrite the code as:
type one struct { id string } func (o *one) id() string { return o.id }
Then your usage site will become:
type ModelWithId interface { Id() string } func Test[M ModelWithId](m M) { fmt.Println(m.Id()) }
The above is the detailed content of How can I define a generic function using a custom structure without listing all structures?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


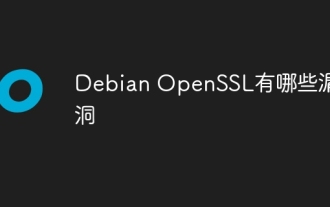
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
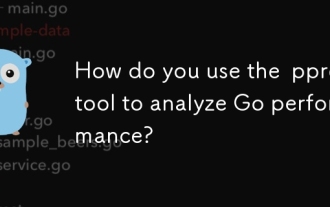
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
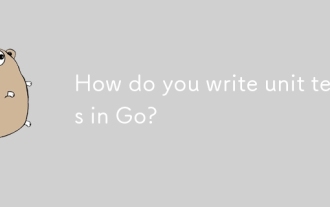
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
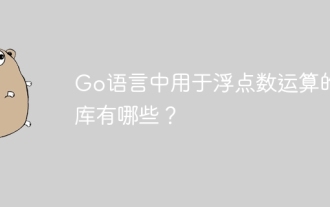
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
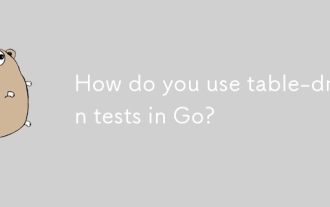
The article discusses using table-driven tests in Go, a method that uses a table of test cases to test functions with multiple inputs and outcomes. It highlights benefits like improved readability, reduced duplication, scalability, consistency, and a
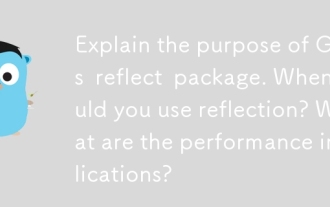
The article discusses Go's reflect package, used for runtime manipulation of code, beneficial for serialization, generic programming, and more. It warns of performance costs like slower execution and higher memory use, advising judicious use and best
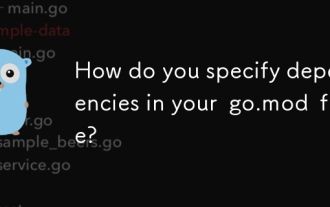
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
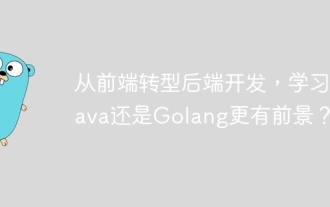
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
