Variable types of input parameters in functions
php editor Apple introduces you to the variable types of input parameters in functions. In PHP, the parameter types of functions can be fixed or variable. Variable type parameters mean that the function can accept different types of parameters as input, which is very useful in processing data in different scenarios. We can declare variadic parameters in function definitions by using special parameter identifiers such as "...". This allows us to handle various types of data more flexibly and improves code reusability and readability. Whether they are strings, numbers, arrays, or other types, we can easily pass them as parameters to functions and process them accordingly inside the function. This flexible way of handling parameter types makes our code more robust and adaptable, able to cope with various complex business needs.
Question content
I created a function to get the user's last comment on a pull request. I'm using the "github.com/google/go-github/github" package. I want to use it for []*github.issuecomment and []*github.pullrequestcomment types. Is there a way to make the input parameter's type mutable so that I don't have to specify it in the function definition and can call the function with either type?
func getlastuserinteractionpr(comments_array *github.issuecomment or *github.pullrequestcomment)(*github.issuecomment or *github.pullrequestcomment) { }
Use of generics:
func getlastuserinteractionpr(comments_array any)(any) { }
This is an emergency solution since the entire project I'm working on is written in go 1.14 and this functionality is available from go 1.18
When I try to use empty interface {} as input type:
func getLastUserInteractionPRIssue(comments_array interface{})(*github.IssueComment) { comments_array []*github.IssueComment(comments_array); err { fmt.Println("success") } else { fmt.Println("failure") } }
Solution
Do you care about the internal structure of example? issuecomment
?
type issuecomment struct { id *int64 `json:"id,omitempty"` nodeid *string `json:"node_id,omitempty"` body *string `json:"body,omitempty"` user *user `json:"user,omitempty"` reactions *reactions `json:"reactions,omitempty"` createdat *time.time `json:"created_at,omitempty"` updatedat *time.time `json:"updated_at,omitempty"` // authorassociation is the comment author's relationship to the issue's repository. // possible values are "collaborator", "contributor", "first_timer", "first_time_contributor", "member", "owner", or "none". authorassociation *string `json:"author_association,omitempty"` url *string `json:"url,omitempty"` htmlurl *string `json:"html_url,omitempty"` issueurl *string `json:"issue_url,omitempty"` }
For example, do you care about extracting some specific fields from it? pullrequestcomment
is a larger struct (it has more fields), do you care about extracting some fields from it?
Or do you just want the string representation of each? What you do depends largely on what you want to do with the passed value.
If you just want each string
representation, you can use the extreme (and honestly, not very safe - I don't recommend this) example and have your function accept fmt.stringer
Slice of object: p>
func dostuffwithstringifiedcomments(cs []fmt.stringer) { // both issuecomment and pullrequestcomment provide string() // methods and therefore implement fmt.stringer for _, comment := range cs { dosomethingwith(comment.string()) } }
Your slices can now contain objects of either type without any explosions happening. Disadvantage: It may also contain billions of other types, none of which are what you want. Therefore, you need to add a type assertion check:
switch t := comment.(type) { case github.issuecomment: // do good stuff case github.pullrequestcomment: // do other good stuff default: // yell and scream about the value of t }
If you want to extract certain fields, you have to compose a function that takes something like []interface{}
(make it part of an empty interface, instead of empty interface represents a slice), iterate over it and type-check each element of the slice, extracting any fields that make sense as long as the element is of the type you expect:
func DoStuff(comments []interface{}) error { for _, c : = range comments { if ic, ok := c.(*github.IssueComment); ok { // Remove the deref if your slice contains values, not references // Pull out fields and do IssueComment-specific things ProcessIssueComment(ic) } else if prc, ok := c.(*github.PullRequestComment); ok { // Do PRComment-specific things ProcessPullRequestComment(prc) } else { return(fmt.Errorf("I did not want something of type %s", t)) } } return nil }
ALSO: Lobby the project owner (if it's not you) to migrate to the current version of go. 1.14 wasn't released until 2020, but that's an eternity for a go release.
The above is the detailed content of Variable types of input parameters in functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


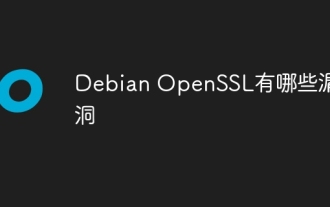
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
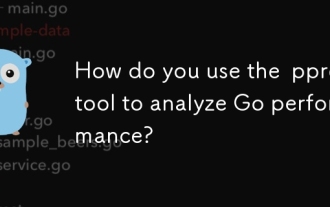
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
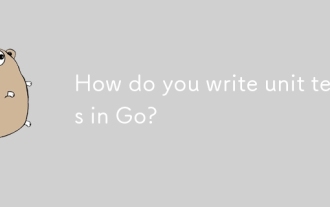
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
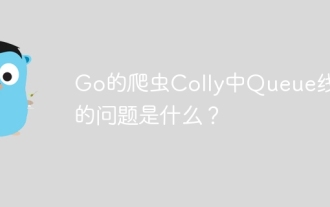
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
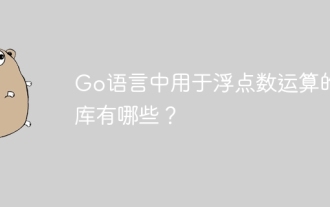
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
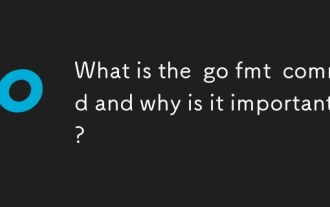
The article discusses the go fmt command in Go programming, which formats code to adhere to official style guidelines. It highlights the importance of go fmt for maintaining code consistency, readability, and reducing style debates. Best practices fo
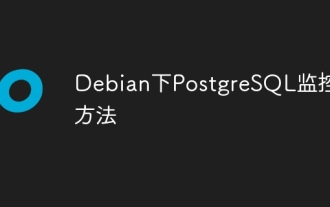
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
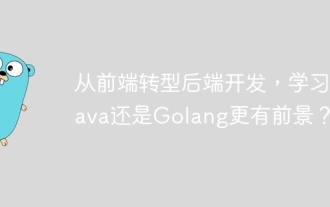
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
