


How to insert a rendered HTML template into a Google Doc using Python
I am facing a challenge to programmatically insert an html template into a google doc using python. I know there is no native/built-in functionality in google docs editor or google docs api that can solve my problem, but I tried some tricks to achieve my goal. Here we are ignoring "where" in the document we should insert, for now just successful insertion is enough.
My method is:
- Upload the html file in google drive with the name
application/vnd.google-apps.document
, because google docs will automatically convert html to document. (Not perfect, but effective) - Use google docs api get() to get the file content (google docs json format).
- Use google docs batchupdate() to update the new content on the target file.
def insert_template_to_file(target_file_id, content): media = MediaIoBaseUpload(BytesIO(content.encode('utf-8')), mimetype='text/html', resumable=True) body = { 'name': 'document_test_html', 'mimeType': 'application/vnd.google-apps.document', 'parents': [DOC_FOLDER_ID] } try: # Create HTML as docs because it automatically convert html to docs content_file = driver_service.files().create(body=body, media_body=media).execute() content_file_id = content_file.get('id') # Collect html content from Google Docs after created doc = docs_service.documents().get(documentId=content_file_id, fields='body').execute() request_content = doc.get('body').get('content') # Insert the content from html to target file result = docs_service.documents().batchUpdate(documentId=target_file_id, body={'requests': request_content}).execute() print(result) # Delete html docs driver_service.files().delete(fileId=content_file_id).execute() print("Content inserted successfuly") except HttpError as error: # Delete html docs even if failed driver_service.files().delete(fileId=content_file_id).execute() print(f"An error occurred: {error}")
The problem is: What I gathered from step 2 does not match what batchupdate() requires. I'm trying to adjust things in step 2 to match step 3, but haven't been successful yet.
Target solution: Get the string with the html code, Insert the rendered html into the target file on google docs. The goal is to append the existing content of the target file to the html, not overwrite it.
Does my approach make sense? Do you have any other ideas to achieve my goal?
Correct answer
I believe your goals are as follows.
- You want to append html data to a google doc by rendering the html.
- You want to use googleapis for python to achieve this.
Unfortunately, at this stage, the json object retrieved by "method:documents.get" does not seem to be directly used as the request body of "method:documents.batchupdate".
However, if you want to append html to an existing google doc, I think you can do it just using the drive api. How about the example script below when this is reflected in the example script?
Sample script:
def insert_template_to_file(target_file_id, content): request = drive_service.files().export(fileId=target_file_id, mimeType="text/html") file = BytesIO() downloader = MediaIoBaseDownload(file, request) done = False while done is False: status, done = downloader.next_chunk() print("Download %d%%" % int(status.progress() * 100)) file.seek(0) current_html = file.read() media = MediaIoBaseUpload(BytesIO(current_html + content.encode('utf-8')), mimetype='text/html', resumable=True) body = {'mimeType': 'application/vnd.google-apps.document'} try: result = drive_service.files().update(fileId=target_file_id, body=body, media_body=media).execute() print(result) print("Content inserted successfuly") except HttpError as error: print(f"An error occurred: {error}")
- In this modified script, the html data is retrieved from the existing google document and the new html is appended to the retrieved html. Also, google docs are updated with updated html. In your case the raw data seems to be html. So I think this method may work.
Notice:
- This script will overwrite the google document of
target_file_id
. So when you test this script, I recommend you use the sample google docs.
references:
The above is the detailed content of How to insert a rendered HTML template into a Google Doc using Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


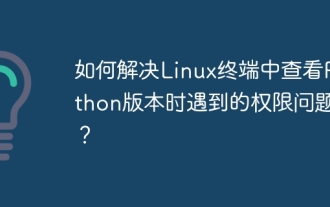
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
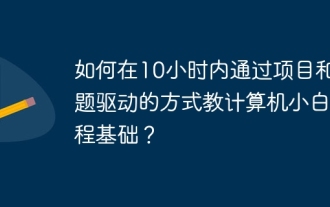
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
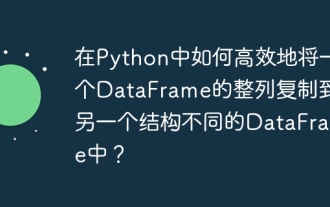
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
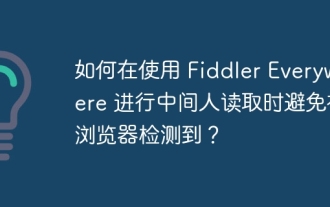
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
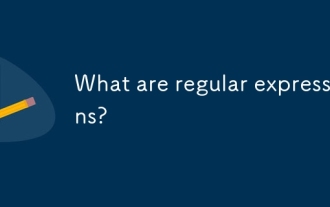
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
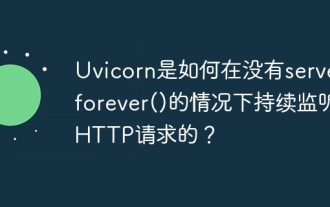
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
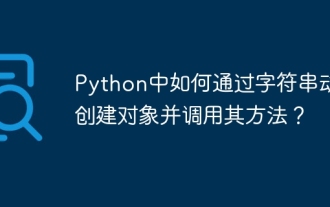
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
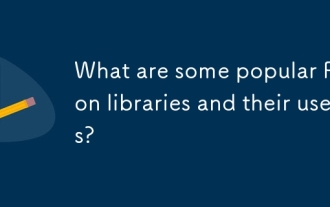
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
