Casting go array using type alias
In the Go language, casting an array using type aliases is a common operation. Type aliases create a new name for an existing type to facilitate type conversion. By using type aliases we can convert an array to an array of another type. This conversion operation is very simple in the Go language and can effectively improve the readability and maintainability of the code. In this article, we will introduce how to cast a Go array using type aliases and provide some examples to help readers better understand this concept.
Question content
Suppose I have a defined type bytes
which is a byte array as shown below. Is there an easy way to convert an array of byte arrays to a byte array and vice versa?
package main type Bytes []byte func main() { x := make([][]byte, 3) y := ([]Bytes)(x) }
Solution
No, unfortunately, go does not allow direct conversion between different types, even if they are aliases. Aliasing is more like giving an existing type a new name, but it doesn't provide any form of automatic conversion.
You need to manually iterate over [][]byte and convert each []byte to type bytes.
The specific operation methods are as follows:
package main import "fmt" type Bytes []byte func main() { x := make([][]byte, 3) // Initializing byte slices for demonstration purposes for i := range x { x[i] = []byte{byte(i), byte(i+1)} } y := make([]Bytes, len(x)) for i, v := range x { y[i] = Bytes(v) } fmt.Println("Hello, 世界", y) }
This code manually iterates x, converting each []byte into a byte and placing it at the corresponding position in y.
The above is the detailed content of Casting go array using type alias. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


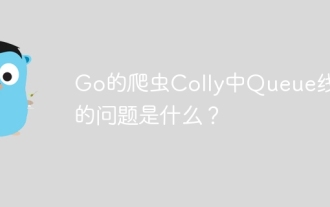
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
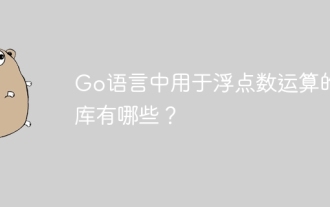
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
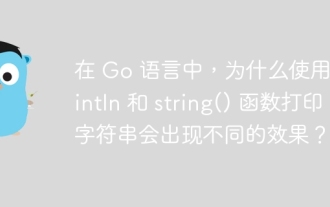
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
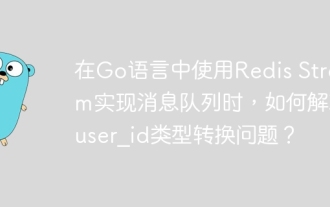
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
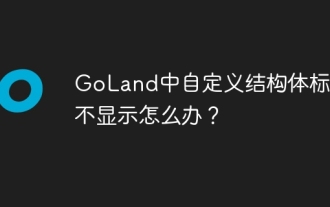
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
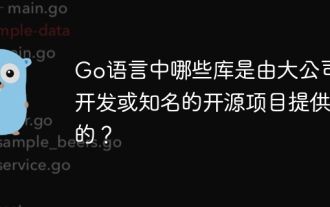
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
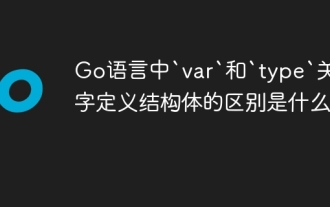
Two ways to define structures in Go language: the difference between var and type keywords. When defining structures, Go language often sees two different ways of writing: First...
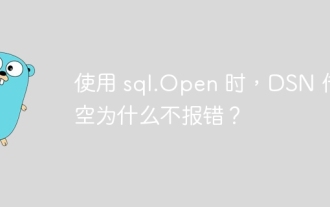
When using sql.Open, why doesn’t the DSN report an error? In Go language, sql.Open...
