Wait for function to complete in golang
In golang, waiting for a function to complete is a common programming requirement. Whether you are waiting for a goroutine to complete or waiting for data in a channel to arrive, you need to use a suitable waiting method to handle it. In this article, we will introduce you to some methods and techniques to wait for function completion in golang. Whether you are a beginner or an experienced developer, this article will provide you with helpful guidance and sample code to help you better handle waiting for functions to complete scenarios. Let’s take a closer look!
Question content
I have the following code in golang:
func A(){ go print("hello") } func main() { A() // here I want to wait for the print to happen B() }
How to ensure that b() is only executed after printing has occurred?
Solution
Use sync.mutex
var l sync.mutex func a() { go func() { print("hello") l.unlock() }() } func b() { print("world") } func testlock(t *testing.t) { l.lock() a() l.lock() // here i want to wait for the print to happen b() l.unlock() }
Use sync.waitgroup
var wg sync.waitgroup func a() { go func() { print("hello") wg.done() }() } func b() { print("world") } func testlock(t *testing.t) { wg.add(1) a() wg.wait() // here i want to wait for the print to happen b() }
Use chan
func A() chan struct{} { c := make(chan struct{}) go func() { print("hello") c <- struct{}{} }() return c } func B() { print("world") } func TestLock(t *testing.T) { c := A() // here I want to wait for the print to happen <-c B() }
The above is the detailed content of Wait for function to complete in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


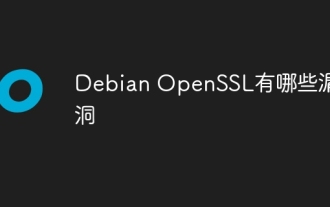
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
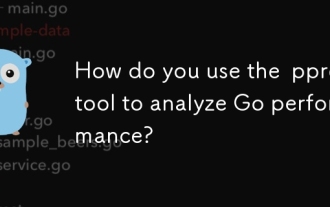
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
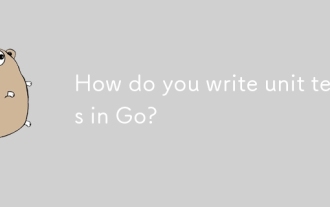
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
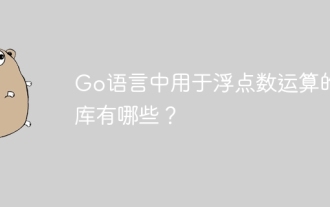
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
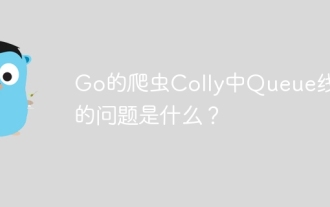
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
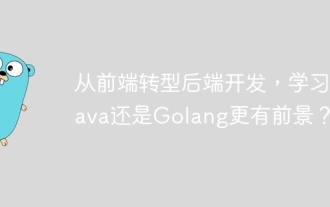
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
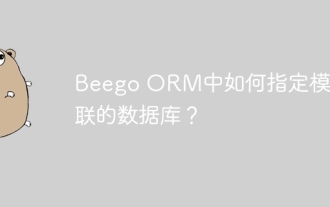
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
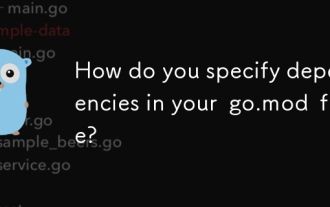
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
