The most powerful thing about HTML5 is the processing of media files. For example, video playback can be achieved by using a simple vedio tag. Similarly, there is a corresponding tag for processing audio files in HTML5, that is, the audio tag
HTML5 has been out for so long, but the audio tag in it has only been used once, and of course it is just to insert this tag. to the page. This time I just took advantage of helping a friend to create a few pages and practice using the audio tag.
First you need to insert an audio tag into the page. Note that it is best not to set loop='loop' here. This attribute is used to set loop playback, because when you need to use the ended attribute later, if loop is set to If looping, the ended attribute will always be false.
autoplay='autoplay' sets the page to automatically play music after loading. The preload and autoplay attributes have the same effect. If the autoplay attribute appears in the tag, the preload attribute will be ignored.
controls='controls' sets the control bar for displaying music.
XML/HTML CodeCopy content to clipboard
- <audio src="music/Yesterday Once More .mp3" id="aud" autoplay="autoplay" controls="controls" preload="auto">
- Your browser does not support the audio attribute, please change the browser to browse.
-
audio>
After you have this tag, congratulations, your page can now play music. But this would make the page too monotonous, so I added some things to the page so that the lyrics can be displayed on the page synchronously and the music to be played can also be selected. So first to achieve this effect, we have to download some lyrics files in lrc format, and then you need to format the music. Because the music file at the beginning looked like this
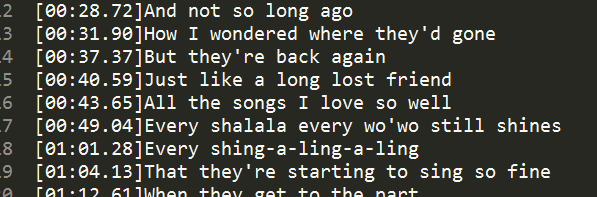
We need to insert each lyric into a two-digit array. After formatting, the lyrics will become like this.
Attached here is the code for formatting lyrics
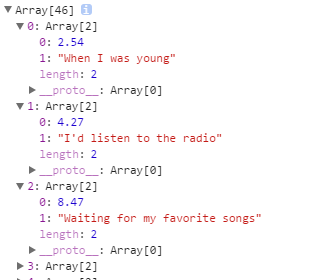
XML/HTML CodeCopy content to clipboard
- //Lyrics synchronization part
- function parseLyric(text) {
- //Separate the text into line by line and store it in the array
-
var lines = text.split('n'),
- //Regular expression used to match time, the matching result is similar to [xx:xx.xx]
-
pattern = /[d{2}:d{2}.d{2}]/g,
- //Array to save the final result
-
result = [];
- //Remove lines without time
- while (!pattern.test(lines[0])) {
-
lineslines = lines.slice(1);
- };
- //When using 'n' to generate the array above, the last element in the result is an empty element, which will be removed here
-
lines[lines.length - 1].length === 0 && lines.pop();
- lines.forEach(function(v /*array element value*/ , i /*element index*/ , a /*array itself*/ ) {
- //Extract time [xx:xx.xx]
-
var time = v.match(pattern),
- //Extract lyrics
-
vvalue = v.replace(pattern, '');
- //Because there may be multiple times in one line, time may be in the form of [xx:xx.xx][xx:xx.xx][xx:xx.xx], which needs to be further separated
- time.forEach(function(v1, i1, a1) {
- //Remove the square brackets in the time to get xx:xx.xx
-
var t = v1.slice(1, -1).split(':');
- //Push the result into the final array
- result.push([parseInt(t[0], 10) * 60 parseFloat(t[1]), value]);
- });
- });
- //Finally, sort the elements in the result array by time so that the lyrics can be displayed normally after saving
- result.sort(function(a, b) {
- return a[0] - b[0];
- });
- return result;
- }
At this point we can easily use the lyrics of each piece of music. We need a function to obtain the lyrics and display them on the page synchronously, so that the music can be switched normally. The code is attached below.
XML/HTML CodeCopy content to clipboard
- function fn(sgname){
- $.get('music/' sgname '.lrc',function(data){
-
var str=parseLyric(data);
-
for(var i=0,li;i<str.length;i ){
- li=$('<li>' str[i][1] 'li>');
- $('#gc ul').append(li);
- }
-
$('#aud')[0].ontimeupdate=function(){//video Triggered when the current playback position of the audio changes
-
for (var i = 0, l = str.length; i < l; i ) {
- if (this.currentTime /*Current playback time*/ > str[i][0]) {
- //Display to page
- $('#gc ul').css('top',-i*40 200 'px'); //Move the lyrics up
- $('#gc ul li').css('color','#fff');
- $('#gc ul li:nth-child(' (i 1) ')').css('color','red'); //Highlight which lyric is currently being played
- }
- }
- if(this.ended){ //Determine whether the currently playing music has finished playing
-
var songslen=$('.songs_list li').length;
-
for(var i= 0,val;i<songslen;i ){
- val=$('.songs_list li:nth-child(' (i 1) ')').text();
- if(val==sgname){
- i=(i==(songslen-1))?1:i 2;
- sgname=$('.songs_list li:nth-child(' i ')').text(); //Switch after the music is finished playing A piece of music
- $('#gc ul').empty(); //Clear lyrics
- $('#aud').attr('src','music/' sgname '.mp3');
fn(sgname); -
return; -
} -
} -
} -
}; -
}); -
} fn($('.songs_list li:nth-child(1)').text()); -
Now here your music lyrics can be displayed on the page normally and synchronously. But there is still one thing missing, which is a list of music. I hope to be able to click on the music in this list to play the music. The code is attached below.
HTML code
XML/HTML Code
Copy content to clipboard
- <div class="songs_cnt">
-
<ul class="songs_list">
-
<li>Yesterday Once Moreli>
-
<li>You Are Beautifulli>
-
ul>
-
<button class="sel_song">点<br/><br/>我button>
-
div>
-
<div id="gc">
-
<ul>ul>
-
div>
css代码
XML/HTML Code复制内容到剪贴板
- #gc{
- width: 400px;
- height: 400px;
- background: transparent;
- margin: 100px auto;
- color: #fff;
- font-size: 18px;
- overflow: hidden;
- position: relative;
- }
- #gc ul{
- position: absolute;
- top: 200px;
- }
- #gc ul li{
- text-align: center;
- height: 40px;
- line-height: 40px;
- }
- .songs_cnt{
- float: left;
- margin-top: 200px;
- position: relative;
- }
- .songs_list{
- background-color: rgba(0,0,0,.2);
- border-radius: 5px;
- float: left;
- width: 250px;
- padding: 15px;
- margin-left: -280px;
- }
- .songs_list li{
- height: 40px;
- line-height: 40px;
- font-size: 16px;
- color: rgba(255,255,255,.8);
- cursor: pointer;
- }
- .songs_list li:hover{
- font-size: 20px;
- color: rgba(255,23,140,.6);
- }
- .sel_song{
- position: absolute;
- top: 50%;
- width: 40px;
- height: 80px;
- margin-top: -40px;
- font-size: 16px;
- text-align: center;
- background-color: transparent;
- border: 1px solid #2DCB70;
- font-weight: bold;
- cursor: pointer;
- border-radius: 3px;
- font-family: sans-serif;
- transition:all 2s;
- -moz-transition:all 2s;
- -webkit-transition:all 2s;
- -o-transition:all 2s;
- }
- .sel_song:hover{
- color: #fff;
- background-color: #2DCB70;
- }
- .songs_list li.active{
- color: #f00;
- }
js代码
XML/HTML Code复制内容到剪贴板
- $('.songs_list li:nth-child(1)').addClass('active');
- $('.songs_cnt').mouseenter(function () {
-
var e=event||window.event;
-
var tag= e.target||e.srcElement;
-
if(tag.nodeName=='BUTTON'){
- $('.songs_list').animate({'marginLeft':'0px'},'slow');
- }
- });
- $('.songs_cnt').mouseleave(function () {
- $('.songs_list').animate({'marginLeft':'-280px'},'slow');
- });
- $('.songs_list li').each(function () {
- $(this).click(function () {
- $('#aud').attr('src','music/' $(this).text() '.mp3');
- $('#gc ul').empty();
- fn($(this).text());
- $('.songs_list li').removeClass('active');
- $(this).addClass('active');
- });
- })
好了,到了这里,那么你的这个歌词同步的效果的一些功能差不多都有了,关于HTML5使用Audio标签实现歌词同步的效果今天也就到这里了。更多信息请登录脚本之家网站了解更多!