What functional programming languages are there?
Functional programming language is a programming paradigm whose core idea is to treat calculations as operations on functions. Functional programming languages are different from traditional imperative programming languages. They emphasize minimizing the state and variability of the program and realizing program functions by converting and combining data. Several common functional programming languages will be introduced below, with corresponding code examples.
- Haskell:
Haskell is a strongly statically typed purely functional programming language with lazy evaluation characteristics. Its code example is as follows:
-- 求阶乘 factorial :: Integer -> Integer factorial 0 = 1 factorial n = n * factorial (n - 1) main :: IO () main = do putStrLn "请输入一个正整数:" n <- readLn putStrLn ("阶乘结果为:" ++ show (factorial n))
- Lisp:
Lisp is one of the earliest functional programming languages. It uses S-expressions to represent code and data and allows functions to be passed as arguments. Here is a simple Lisp example:
; 定义阶乘函数 (defun factorial (n) (if (<= n 1) 1 (* n (factorial (- n 1))))) ; 调用阶乘函数 (print (factorial 5))
- Clojure:
Clojure is a Lisp-based functional programming language that runs on the Java Virtual Machine and provides Concurrent programming support. Here is an example from Clojure:
; 定义阶乘函数 (defn factorial [n] (if (<= n 1) 1 (* n (factorial (- n 1))))) ; 调用阶乘函数 (println (factorial 5))
- Erlang:
Erlang is a concurrent programming language that is widely used in the development of high-availability systems. Here is an example in Erlang:
% 定义阶乘函数 factorial(0) -> 1; factorial(N) -> N * factorial(N - 1). % 调用阶乘函数 io:format("~p~n", [factorial(5)]).
- Swift:
Swift is a modern multi-paradigm programming language that supports functional programming. The following is a Swift example:
// 定义阶乘函数 func factorial(_ n: Int) -> Int { if n <= 1 { return 1 } return n * factorial(n - 1) } // 调用阶乘函数 let result = factorial(5) print(result)
The above are code examples for several common functional programming languages. Through these examples, we can learn about the syntax and features of different functional programming languages and how to use them to implement a functional programming style. Of course, in addition to the functional programming languages mentioned above, there are many other languages that also support or partially support functional programming, such as Python, JavaScript, etc.
The above is the detailed content of What functional programming languages are there?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


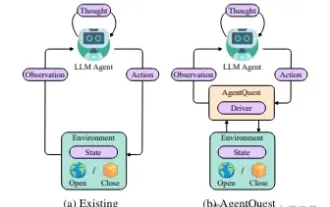
Based on the continuous optimization of large models, LLM agents - these powerful algorithmic entities have shown the potential to solve complex multi-step reasoning tasks. From natural language processing to deep learning, LLM agents are gradually becoming the focus of research and industry. They can not only understand and generate human language, but also formulate strategies, perform tasks in diverse environments, and even use API calls and coding to Build solutions. In this context, the introduction of the AgentQuest framework is a milestone. It not only provides a modular benchmarking platform for the evaluation and advancement of LLM agents, but also provides researchers with a Powerful tools to track and improve the performance of these agents at a more granular level
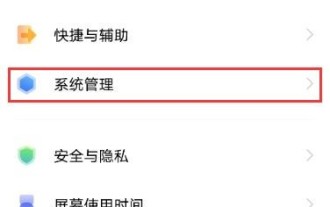
1. Click [System Management] in the phone settings menu. 2. Click the [Language] option. 3. Select the system language you want to use.
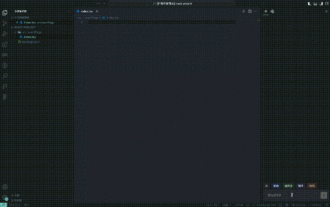
1. Code specifications during background reconstruction work: During the B-end front-end development process, developers will always face the pain point of repeated development. The element modules of many CRUD pages are basically similar, but they still need to be developed manually, and time is spent on simple element construction. This reduces the development efficiency of business requirements. At the same time, because the coding styles of different developers are inconsistent, it makes it more expensive for others to get started during iterations. AI replaces simple brainpower: With the continuous development of large AI models, it has simple understanding capabilities and can convert language into instructions. General instructions for building basic pages can meet the needs of daily basic page building and improve the efficiency of business development in general scenarios. 2. Generate link list. B-side page lists, forms, and details can all be generated. Links can be roughly divided into the following categories:
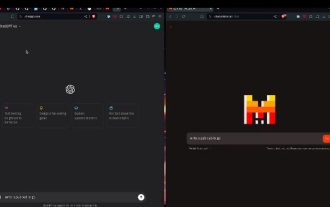
Produced by 51CTO technology stack (WeChat ID: blog51cto) Mistral released its first code model Codestral-22B! What’s crazy about this model is not only that it’s trained on over 80 programming languages, including Swift, etc. that many code models ignore. Their speeds are not exactly the same. It is required to write a "publish/subscribe" system using Go language. The GPT-4o here is being output, and Codestral is handing in the paper so fast that it’s hard to see! Since the model has just been launched, it has not yet been publicly tested. But according to the person in charge of Mistral, Codestral is currently the best-performing open source code model. Friends who are interested in the picture can move to: - Hug the face: https
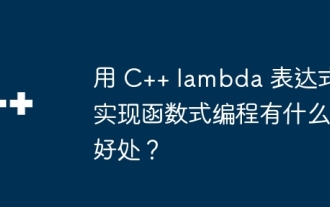
C++ lambda expressions bring advantages to functional programming, including: Simplicity: Anonymous inline functions improve code readability. Code reuse: Lambda expressions can be passed or stored to facilitate code reuse. Encapsulation: Provides a way to encapsulate a piece of code without creating a separate function. Practical case: filtering odd numbers in the list. Calculate the sum of elements in a list. Lambda expressions achieve the simplicity, reusability, and encapsulation of functional programming.
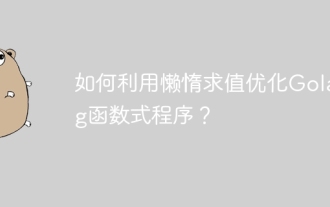
Lazy evaluation can be implemented in Go by using lazy data structures: creating a wrapper type that encapsulates the actual value and only evaluates it when needed. Optimize the calculation of Fibonacci sequences in functional programs, deferring the calculation of intermediate values until actually needed. This can eliminate unnecessary overhead and improve the performance of functional programs.
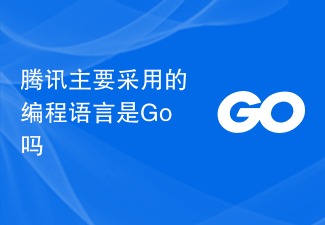
Title: Does Tencent mainly use Go language? Exploring the programming language selection in Tencent’s technology stack In recent years, with the rapid development of Go language around the world, more and more technology companies have begun to choose Go language as one of their main programming languages. As China's leading technology company, will Tencent also incorporate Go language into its technology stack and become one of its main programming languages? In this article, we will explore whether Tencent mainly uses Go language in technology development and give specific code examples for analysis. First, we need

There are five common mistakes and pitfalls to be aware of when using functional programming in Go: Avoid accidental modification of references and ensure that newly created variables are returned. To resolve concurrency issues, use synchronization mechanisms or avoid capturing external mutable state. Use partial functionalization sparingly to improve code readability and maintainability. Always handle errors in functions to ensure the robustness of your application. Consider the performance impact and optimize your code using inline functions, flattened data structures, and batching of operations.
