


C language function and sample code to implement exponentiation function
The implementation principle and sample code of C language exponentiation function
Title: The implementation principle and sample code of C language exponentiation function
Introduction:
In computer programming, exponentiation is a common operation. C language is a widely used programming language. In order to simplify the process of exponentiation operation, we can write a exponentiation function. This article will introduce the implementation principle of the power function and provide a specific example code. I hope that the explanation in this article can help readers better understand and use the power function.
1. Implementation principles of exponentiation functions
There are two commonly used implementation principles of exponentiation functions: loop iteration and recursion. The specific details of these two implementation principles will be introduced below.
- The implementation principle of loop iteration
Loop iteration is a simple and intuitive method that implements exponentiation operations through multiple loop multiplications. The specific implementation process is as follows:
double power_iterative(double base, int exponent) { double result = 1.0; while (exponent > 0) { if (exponent % 2 != 0) { result *= base; } base *= base; exponent /= 2; } return result; }
In the above code, a loop is used to iteratively calculate the result of the power. When the exponent is an odd number, the base is multiplied by the result; after each loop, the base is squared and the exponent is divided by 2. When the index is 0, the loop ends and the final result is returned.
- The implementation principle of recursion
Recursion is a way for a function to call itself to implement exponentiation. The specific implementation process is as follows:
double power_recursive(double base, int exponent) { if (exponent == 0) { return 1.0; } if (exponent < 0) { return 1.0 / power_recursive(base, -exponent); } double half = power_recursive(base, exponent / 2); if (exponent % 2 == 0) { return half * half; } else { return base * half * half; } }
In the above code, the exponentiation function realizes the calculation of exponentiation by continuously halving the exponent and calling itself recursively. When the exponent is 0, 1 is returned; when the exponent is negative, the result is the reciprocal. Reduce the size of the exponent by dividing it by 2, thus reducing the amount of calculations.
2. Sample code
The following is a sample code using the power function, used to calculate the 10th power of 2:
#include <stdio.h> // 使用循环迭代方式实现乘方运算 double power_iterative(double base, int exponent); // 使用递归方式实现乘方运算 double power_recursive(double base, int exponent); int main() { double result_iterative = power_iterative(2, 10); double result_recursive = power_recursive(2, 10); printf("使用循环迭代方式计算结果:%f ", result_iterative); printf("使用递归方式计算结果:%f ", result_recursive); return 0; } double power_iterative(double base, int exponent) { // 省略代码,参考上文的实现 } double power_recursive(double base, int exponent) { // 省略代码,参考上文的实现 }
Output result:
Use loop iteration method Calculation result: 1024.000000
Calculation result using recursion: 1024.000000
In this sample code, we use loop iteration and recursion to calculate 2 to the 10th power, and print the results.
Conclusion:
This article introduces the implementation principle of the power function and provides a specific example code. Through the implementation of the exponentiation function, we can simplify the process of exponentiation operation and make the code more concise and readable. I hope the explanation in this article can help readers better understand and use the power function.
The above is the detailed content of C language function and sample code to implement exponentiation function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


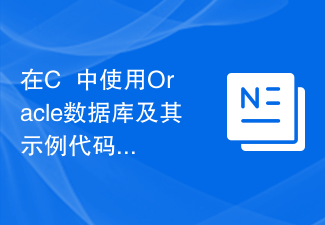
Oracle is a powerful relational database management system. Using Oracle database in C++ can help us manage the database more efficiently. This article will introduce how to use Oracle database and related sample code in C++. 1. Install and configure the Oracle database driver. Before using the Oracle database, you need to install the corresponding Oracle driver. Oracle officially provides ODBC drivers, which we can download and install from the official website. After installation is complete
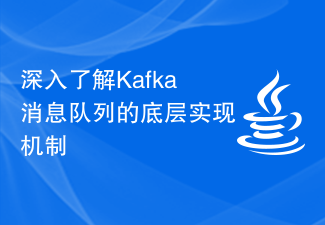
Overview of the underlying implementation principles of Kafka message queue Kafka is a distributed, scalable message queue system that can handle large amounts of data and has high throughput and low latency. Kafka was originally developed by LinkedIn and is now a top-level project of the Apache Software Foundation. Architecture Kafka is a distributed system consisting of multiple servers. Each server is called a node, and each node is an independent process. Nodes are connected through a network to form a cluster. K
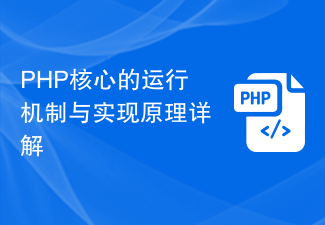
PHP is a popular open source server-side scripting language that is heavily used for web development. It can handle dynamic data and control HTML output, but how to achieve this? Then, this article will introduce the core operating mechanism and implementation principles of PHP, and use specific code examples to further illustrate its operating process. PHP source code interpretation PHP source code is a program written in C language. After compilation, it generates the executable file php.exe. For PHP used in Web development, it is generally executed through A

Binary file operations and sample codes in C++ In C++, binary files are files stored in binary format and can contain any type of data, including integers, floating point numbers, characters, structures, etc. At the same time, these binary files can also be processed Read and write operations. This article will introduce you to binary file operations in C++ and provide some sample codes to help you better understand and use binary file operations. To open a file in C++, you can use the file stream object in the fstream library to open a file.
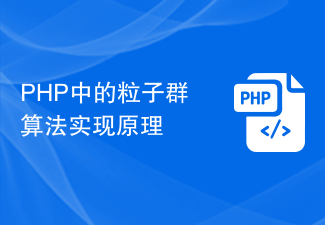
Principle of Particle Swarm Optimization Implementation in PHP Particle Swarm Optimization (PSO) is an optimization algorithm often used to solve complex nonlinear problems. It simulates the foraging behavior of a flock of birds to find the optimal solution. In PHP, we can use the PSO algorithm to quickly solve problems. This article will introduce its implementation principle and give corresponding code examples. Basic Principle of Particle Swarm Optimization The basic principle of particle swarm algorithm is to find the optimal solution through iterative search. There is a group of particles in the algorithm
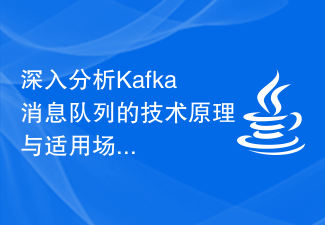
The implementation principle of Kafka message queue Kafka is a distributed publish-subscribe messaging system that can handle large amounts of data and has high reliability and scalability. The implementation principle of Kafka is as follows: 1. Topics and partitions Data in Kafka is stored in topics, and each topic can be divided into multiple partitions. A partition is the smallest storage unit in Kafka, which is an ordered, immutable log file. Producers write data to topics, and consumers read from
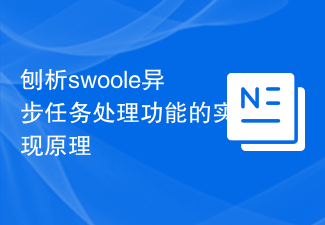
Analyze the implementation principle of swoole's asynchronous task processing function. With the rapid development of Internet technology, the processing of various problems has become more and more complex. In web development, handling a large number of requests and tasks is a common challenge. The traditional synchronous blocking method cannot meet the needs of high concurrency, so asynchronous task processing becomes a solution. As a PHP coroutine network framework, Swoole provides powerful asynchronous task processing functions. This article will use a simple example to analyze its implementation principle. Before we start, we need to make sure we have
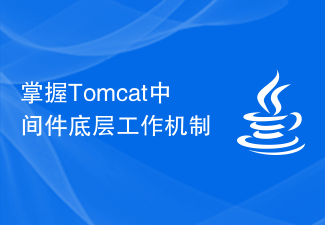
To understand the underlying implementation principles of Tomcat middleware, you need specific code examples. Tomcat is an open source, widely used Java Web server and Servlet container. It is highly scalable and flexible and is commonly used to deploy and run Java Web applications. In order to better understand the underlying implementation principles of Tomcat middleware, we need to explore its core components and operating mechanism. This article will analyze the underlying implementation principles of Tomcat middleware through specific code examples. Tom
