In-depth analysis of JVM memory model: master the core concepts
JVM memory model revealed: To understand its core concepts, specific code examples are needed
Introduction:
The Java Virtual Machine (JVM) serves as the execution environment for Java programs. Responsible for converting Java bytecode into machine code and executing it. In Java development, we often encounter memory-related problems, such as memory leaks, memory overflows, etc. Understanding the core concepts of the JVM memory model is the key to solving these problems. This article will reveal the JVM memory model from the perspectives of stack, heap, method area, etc., and help readers better understand through specific code examples.
1. Stack
The stack is the thread private memory area in the JVM. Each thread will have an independent stack. The stack is managed in the form of method calls. Each method call creates a new stack frame (Frame) on the stack. The stack frame contains the method's local variable table (Local Variable Table), operand stack (Operand Stack), dynamic linking (Dynamic Linking), method return address (Return Address) and other information.
The following is a simple sample code that demonstrates the basic characteristics of stack memory:
public class StackDemo { public static void main(String[] args) { int a = 1; int b = 2; int sum = add(a, b); System.out.println("sum: " + sum); } public static int add(int a, int b) { return a + b; } }
In this example, when the add method is executed, the JVM will create a new one on the stack stack frame, and store the method parameters a and b in the local variable table. When execution is completed, the stack frame will be popped and the corresponding memory will be released.
2. Heap
The heap is a thread-shared memory area in the JVM, used to store instances of objects. In a Java program, all objects created through the new keyword will be stored on the heap. The JVM manages heap memory through the garbage collection mechanism and automatically recycles objects that are no longer used.
The following is a simple sample code that demonstrates the basic characteristics of heap memory:
public class HeapDemo { public static void main(String[] args) { MyClass obj1 = new MyClass(); MyClass obj2 = new MyClass(); } } class MyClass { private int myVariable; public MyClass() { // 构造方法 } }
In this example, two MyClass objects created through the new keyword will be stored on the heap. When an object is no longer referenced, the garbage collection mechanism automatically reclaims it.
3. Method Area
The method area is a thread-shared memory area in the JVM, used to store loaded class information, constant pools, static variables, compiler-compiled code, etc. . The method area is created when the JVM starts and its size is fixed.
The following is a simple sample code that demonstrates the basic features of the method area:
public class MethodAreaDemo { public static void main(String[] args) { String str1 = "Hello"; String str2 = "World"; String message = str1 + str2; System.out.println(message); } }
In this example, the strings "Hello" and "World" are both stored in the method area in the constant pool. When two strings are added, the JVM will create a new string object on the heap to store the combined result.
Conclusion:
Understanding the core concepts of the JVM memory model is very important for Java developers. The stack, heap, and method area are respectively responsible for different memory management tasks. Some common memory problems can be avoided through reasonable use and optimization. This article uses specific code examples to help readers better understand the core concepts of the JVM memory model. However, it should be noted that the JVM memory model is a very large topic. This article only briefly introduces part of it. Readers can learn more through further study.
The above is the detailed content of In-depth analysis of JVM memory model: master the core concepts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
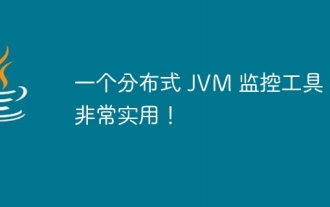
This project is designed to facilitate developers to monitor multiple remote host JVMs faster. If your project is Spring boot, it is very easy to integrate. Just introduce the jar package. If it is not Spring boot, don’t be discouraged. You can quickly initialize a Spring boot program and introduce it yourself. Jar package is enough

When multiple goroutines access the same data concurrently, the concurrent access operations must be serialized. In Go, the serialization of reads and writes can be guaranteed through channel communication or other synchronization primitives.
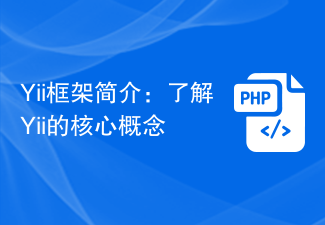
The Yii framework is a high-performance, highly scalable, and highly maintainable PHP development framework that is highly efficient and reliable when developing Web applications. The main advantage of the Yii framework is its unique features and development methods, while also integrating many practical tools and functions. The core concept of the Yii framework, the MVC pattern, Yii adopts the MVC (Model-View-Controller) pattern, which is a pattern that divides the application into three independent parts, namely the business logic processing model and the user interface presentation model.
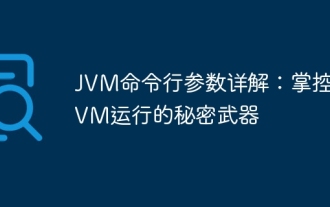
JVM command line parameters allow you to adjust JVM behavior at a fine-grained level. The common parameters include: Set the Java heap size (-Xms, -Xmx) Set the new generation size (-Xmn) Enable the parallel garbage collector (-XX:+UseParallelGC) Reduce the memory usage of the Survivor area (-XX:-ReduceSurvivorSetInMemory) Eliminate redundancy Eliminate garbage collection (-XX:-EliminateRedundantGCs) Print garbage collection information (-XX:+PrintGC) Use the G1 garbage collector (-XX:-UseG1GC) Set the maximum garbage collection pause time (-XX:MaxGCPau
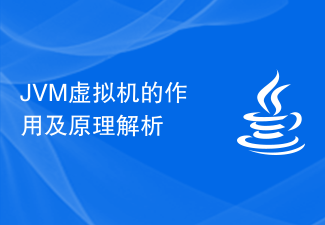
An introduction to the analysis of the functions and principles of the JVM virtual machine: The JVM (JavaVirtualMachine) virtual machine is one of the core components of the Java programming language, and it is one of the biggest selling points of Java. The role of the JVM is to compile Java source code into bytecodes and be responsible for executing these bytecodes. This article will introduce the role of JVM and how it works, and provide some code examples to help readers understand better. Function: The main function of JVM is to solve the problem of portability of Java programs on different platforms.
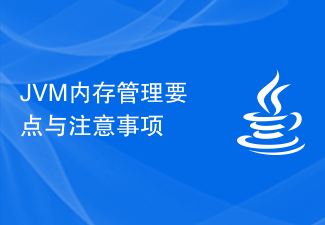
Key points and precautions for mastering JVM memory usage JVM (JavaVirtualMachine) is the environment in which Java applications run, and the most important one is the memory management of the JVM. Properly managing JVM memory can not only improve application performance, but also avoid problems such as memory leaks and memory overflows. This article will introduce the key points and considerations of JVM memory usage and provide some specific code examples. JVM memory partitions JVM memory is mainly divided into the following areas: Heap (He
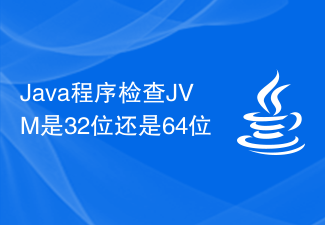
Before writing a java program to check whether the JVM is 32-bit or 64-bit, let us first discuss about the JVM. JVM is a java virtual machine, responsible for executing bytecode. It is part of the Java Runtime Environment (JRE). We all know that java is platform independent, but JVM is platform dependent. We need separate JVM for each operating system. If we have the bytecode of any java source code, we can easily run it on any platform due to JVM. The entire process of java file execution is as follows - First, we save the java source code with .java extension and the compiler converts it into bytecode with .class extension. This happens at compile time. Now, at runtime, J
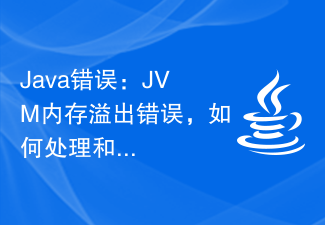
Java is a popular programming language. During the development of Java applications, you may encounter JVM memory overflow errors. This error usually causes the application to crash, affecting the user experience. This article will explore the causes of JVM memory overflow errors and how to deal with and avoid such errors. What is JVM memory overflow error? The Java Virtual Machine (JVM) is the running environment for Java applications. In the JVM, memory is divided into multiple areas, including heap, method area, stack, etc. The heap is used to store created objects
