How to correctly use the exit function in C language
How to use the exit function in C language, you need specific code examples
In C language, we often need to terminate the execution of the program early in the program, or in a certain Exit the program under certain conditions. C language provides the exit() function to implement this function. This article will introduce the usage of exit() function and provide corresponding code examples.
The exit() function is a standard library function in C language, which is included in the header file
The prototype of the exit() function is as follows:
1 |
|
Among them, status is the status code when the program exits. As a general rule, a status code of 0 means that the program exited normally, and a status code other than 0 means that the program exited abnormally or an error occurred.
The following is a simple sample code:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
In the above code, first we receive an integer input by the user through the scanf() function. Then, we use if-else statements to judge based on the value entered by the user. If the input number is less than 0, an error message is output, and the exit(1) function is called to exit the program, and status code 1 is returned; if the input number is greater than or equal to 0, a normal exit message is output, and the exit(0) function is called to exit the program. , return status code 0.
It should be noted that the call to the exit() function will immediately terminate the execution of the program, so you need to ensure that the code before the exit() function is called has been completed, such as closing files, releasing memory, etc. In addition, when the exit() function exits the program, it will call various cleanup functions (such as the function registered with atexit()) to ensure the aftermath before the program ends.
In summary, the exit() function is a function in C language that is used to terminate program execution early and return a status code. By calling the exit() function, we can flexibly control the exit of the program in the program and respond to different exceptions. I hope the content of this article can help you better understand and use the exit() function.
The above is the detailed content of How to correctly use the exit function in C language. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


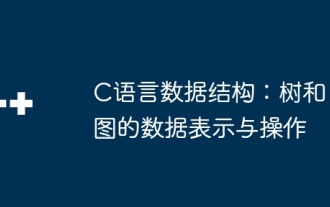
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
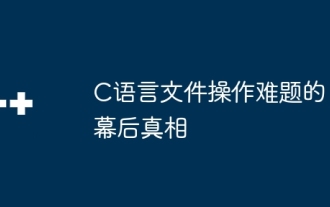
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
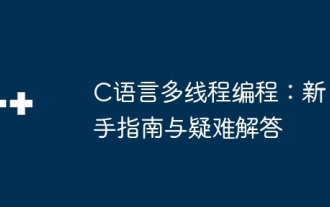
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
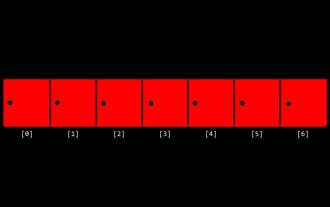
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
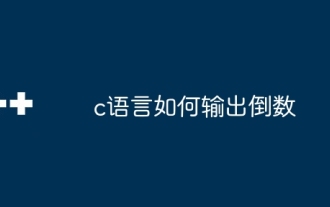
How to output a countdown in C? Answer: Use loop statements. Steps: 1. Define the variable n and store the countdown number to output; 2. Use the while loop to continuously print n until n is less than 1; 3. In the loop body, print out the value of n; 4. At the end of the loop, subtract n by 1 to output the next smaller reciprocal.
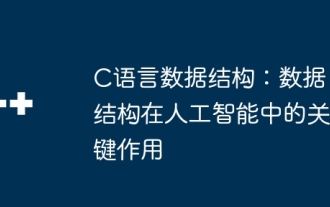
C Language Data Structure: Overview of the Key Role of Data Structure in Artificial Intelligence In the field of artificial intelligence, data structures are crucial to processing large amounts of data. Data structures provide an effective way to organize and manage data, optimize algorithms and improve program efficiency. Common data structures Commonly used data structures in C language include: arrays: a set of consecutively stored data items with the same type. Structure: A data type that organizes different types of data together and gives them a name. Linked List: A linear data structure in which data items are connected together by pointers. Stack: Data structure that follows the last-in first-out (LIFO) principle. Queue: Data structure that follows the first-in first-out (FIFO) principle. Practical case: Adjacent table in graph theory is artificial intelligence
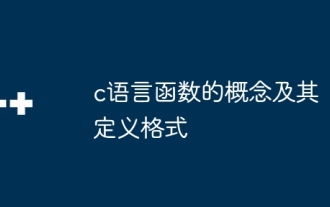
C language functions are reusable code blocks, receive parameters for processing, and return results. It is similar to the Swiss Army Knife, powerful and requires careful use. Functions include elements such as defining formats, parameters, return values, and function bodies. Advanced usage includes function pointers, recursive functions, and callback functions. Common errors are type mismatch and forgetting to declare prototypes. Debugging skills include printing variables and using a debugger. Performance optimization uses inline functions. Function design should follow the principle of single responsibility. Proficiency in C language functions can significantly improve programming efficiency and code quality.
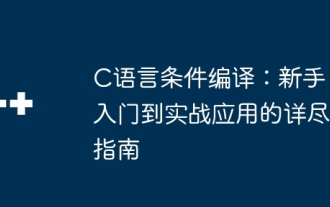
C language conditional compilation is a mechanism for selectively compiling code blocks based on compile-time conditions. The introductory methods include: using #if and #else directives to select code blocks based on conditions. Commonly used conditional expressions include STDC, _WIN32 and linux. Practical case: Print different messages according to the operating system. Use different data types according to the number of digits of the system. Different header files are supported according to the compiler. Conditional compilation enhances the portability and flexibility of the code, making it adaptable to compiler, operating system, and CPU architecture changes.
