How to sort an array using sort in Vue
How to use sort in vue, you need specific code examples
Vue.js is a popular front-end framework that provides many convenient methods and instructions for processing data. One of the common requirements is to sort arrays, and the sort method of Vue.js can meet this requirement very well. This article will introduce how to use the sort method of Vue.js to sort an array and provide specific code examples.
First, we need to create a Vue instance and define an array in its data option. Assume that our array is an unordered array containing some numbers, as shown below:
new Vue({ el: '#app', data: { numbers: [5, 2, 8, 1, 9] } })
Next, we can use the sort method in the method of the Vue instance to sort the array. The sort method is a method of JavaScript native array objects. It can accept a comparison function as a parameter for sorting. In the methods option in the Vue instance, we can define a sortArray method to sort the array.
new Vue({ el: '#app', data: { numbers: [5, 2, 8, 1, 9] }, methods: { sortArray: function() { this.numbers.sort(function(a, b) { return a - b; }); } } })
In the sortArray method, we use JavaScript's sorting rules to compare array elements. If a is less than b, the sort method will return a negative value, so that a will be ranked in front of b; if a is greater than b, the sort method will return a positive value, so that a will be ranked behind b. Therefore, ascending sorting can be achieved by returning a - b;.
In the Vue instance, we can call the sortArray method by using the v-on directive in the HTML template to trigger the array sorting operation. For example, we can call the sortArray method on a button's click event.
<div id="app"> <button v-on:click="sortArray">排序数组</button> <ul> <li v-for="number in numbers">{{ number }}</li> </ul> </div>
In the above code, we use the v-on instruction to bind the click event to the button, and use the sortArray method as the event handler. When the button is clicked, the sortArray method is called to sort the array. Finally, we use the v-for directive to display the sorted array on the page.
The complete sample code is as follows:
<!DOCTYPE html> <html> <head> <title>Vue Sort Example</title> <script src="https://cdn.jsdelivr.net/npm/vue/dist/vue.js"></script> </head> <body> <div id="app"> <button v-on:click="sortArray">排序数组</button> <ul> <li v-for="number in numbers">{{ number }}</li> </ul> </div> <script> new Vue({ el: '#app', data: { numbers: [5, 2, 8, 1, 9] }, methods: { sortArray: function() { this.numbers.sort(function(a, b) { return a - b; }); } } }) </script> </body> </html>
The above is how to sort the array using the sort method of Vue.js, and achieve ascending order of the array by triggering the button click event. Through this example, you can learn how to use the sort method to sort an array in Vue.js, and understand how to call methods and bind events in a Vue instance. Hope this helps!
The above is the detailed content of How to sort an array using sort in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


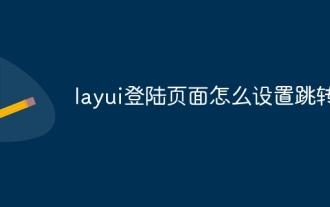
Layui login page jump setting steps: Add jump code: Add judgment in the login form submit button click event, and jump to the specified page through window.location.href after successful login. Modify the form configuration: add a hidden input field to the form element of lay-filter="login", with the name "redirect" and the value being the target page address.
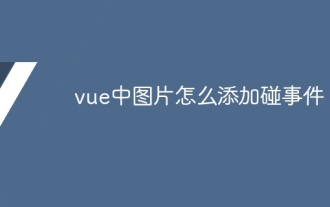
How to add click event to image in Vue? Import the Vue instance. Create a Vue instance. Add images to HTML templates. Add click events using the v-on:click directive. Define the handleClick method in the Vue instance.
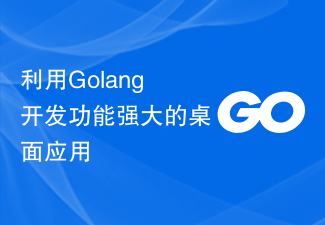
Use Golang to develop powerful desktop applications. With the continuous development of the Internet, people have become inseparable from various types of desktop applications. For developers, it is crucial to use efficient programming languages to develop powerful desktop applications. This article will introduce how to use Golang (Go language) to develop powerful desktop applications and provide some specific code examples. Golang is an open source programming language developed by Google. It has the characteristics of simplicity, efficiency, strong concurrency, etc., and is very suitable for
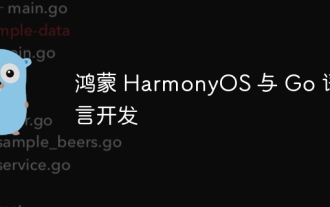
Introduction to HarmonyOS and Go language development HarmonyOS is a distributed operating system developed by Huawei, and Go is a modern programming language. The combination of the two provides a powerful solution for developing distributed applications. This article will introduce how to use Go language for development in HarmonyOS, and deepen understanding through practical cases. Installation and Setup To use Go language to develop HarmonyOS applications, you need to install GoSDK and HarmonyOSSDK first. The specific steps are as follows: #Install GoSDKgogetgithub.com/golang/go#Set PATH
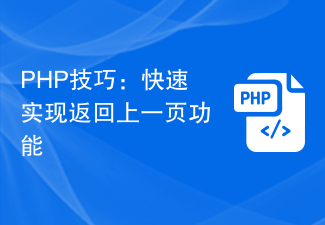
PHP Tips: Quickly implement the function of returning to the previous page. In web development, we often encounter the need to implement the function of returning to the previous page. Such operations can improve the user experience and make it easier for users to navigate between web pages. In PHP, we can achieve this function through some simple code. This article will introduce how to quickly implement the function of returning to the previous page and provide specific PHP code examples. In PHP, we can use $_SERVER['HTTP_REFERER'] to get the URL of the previous page
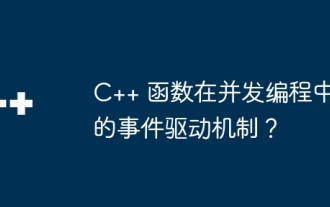
The event-driven mechanism in concurrent programming responds to external events by executing callback functions when events occur. In C++, the event-driven mechanism can be implemented with function pointers: function pointers can register callback functions to be executed when events occur. Lambda expressions can also implement event callbacks, allowing the creation of anonymous function objects. The actual case uses function pointers to implement GUI button click events, calling the callback function and printing messages when the event occurs.
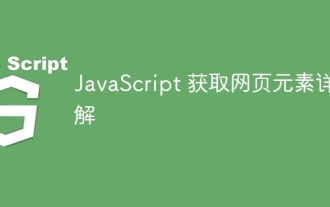
Answer: JavaScript provides a variety of methods for obtaining web page elements, including using ids, tag names, class names, and CSS selectors. Detailed description: getElementById(id): Get elements based on unique id. getElementsByTagName(tag): Gets the element group with the specified tag name. getElementsByClassName(class): Gets the element group with the specified class name. querySelector(selector): Use CSS selector to get the first matching element. querySelectorAll(selector): Get all matches using CSS selector
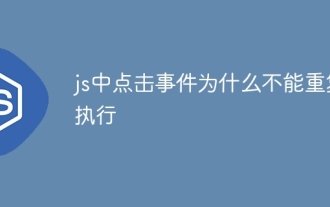
Click events in JavaScript cannot be executed repeatedly because of the event bubbling mechanism. To solve this problem, you can take the following measures: Use event capture: Specify an event listener to fire before the event bubbles up. Handing over events: Use event.stopPropagation() to stop event bubbling. Use a timer: trigger the event listener again after some time.
