


A detailed description of the five states of Java threads and their characteristics and performance in multi-threaded environments
Details the five states of Java threads and their characteristics and performance in a multi-threaded environment
Java is an object-oriented programming language, and its multi-threading The features allow us to perform multiple tasks at the same time, improving the concurrency and responsiveness of the program. In Java, threads have five different states, namely new state (New), runnable state (Runnable), blocked state (Blocked), waiting state (Waiting) and terminated state (Terminated). This article will introduce the characteristics of these five states in detail, and show the performance in a multi-threaded environment through specific code examples.
1. New state (New)
The state in which a thread is created but has not yet started running is called the new state. In the newly created state, the thread's start() method has not yet been called, so execution has not actually started yet. At this point, the thread object has been created, but the operating system has not allocated execution resources to it.
2. Runnable state (Runnable)
After the thread is called by the start() method, it enters the runnable state. The thread in this state is using the CPU to perform its tasks, but may be suspended due to other high-priority threads, running out of time slices, or waiting for input/output. In the runnable state, threads have the following characteristics:
- The thread in this state is the basic unit of operating system scheduling.
- Multiple threads execute concurrently, and CPU time slices are allocated to each thread so that they execute alternately.
- Thread scheduling is uncontrollable because it is determined by the operating system.
The following is a simple code example showing the runnable status of two threads:
class MyRunnable implements Runnable{ public void run(){ for(int i=0; i<10; i++){ System.out.println(Thread.currentThread().getName() + ": " + i); } } } public class Main { public static void main(String[] args) { Thread t1 = new Thread(new MyRunnable()); Thread t2 = new Thread(new MyRunnable()); t1.start(); t2.start(); } }
In the above example, we created two threads t1 and t2, and start them simultaneously. Since both threads run concurrently, their output will alternate.
3. Blocked state (Blocked)
The thread enters the blocking state because it cannot obtain certain resources or waits for certain conditions to be met. A thread in a blocked state will not consume CPU time until it obtains resources or enters a runnable state when conditions are met.
The following is a simple code example showing the blocking state of a thread:
public class Main { public static void main(String[] args) { Object lock = new Object(); Thread t1 = new Thread(() -> { synchronized (lock) { try { System.out.println("Thread 1 is waiting"); lock.wait(); System.out.println("Thread 1 is running again"); } catch (InterruptedException e) { e.printStackTrace(); } } }); Thread t2 = new Thread(() -> { try { Thread.sleep(2000); synchronized (lock) { System.out.println("Thread 2 is waking up Thread 1"); lock.notify(); } } catch (InterruptedException e) { e.printStackTrace(); } }); t1.start(); t2.start(); } }
In the above example, we created two threads t1 and t2, t1 is in the execution process Enter the waiting state by calling the wait() method until t2 wakes it up through the notify() method. The reason t1 is blocked here is that it cannot continue execution until t2 issues notification. When t2 sends a notification, t1 unblocks and re-enters the runnable state.
4. Waiting state (Waiting)
The thread enters the waiting state because it needs to wait for other threads to take some specific actions. A thread in a waiting state waits until it is notified or interrupted.
The following is a simple code example showing the waiting state of a thread:
public class Main { public static void main(String[] args) { Object lock = new Object(); Thread t1 = new Thread(() -> { synchronized (lock) { System.out.println("Thread 1 is waiting"); try { lock.wait(); // 进入等待状态 } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Thread 1 is running again"); } }); Thread t2 = new Thread(() -> { try { Thread.sleep(2000); synchronized (lock) { System.out.println("Thread 2 is waking up Thread 1"); lock.notify(); // 唤醒等待的线程 } } catch (InterruptedException e) { e.printStackTrace(); } }); t1.start(); t2.start(); } }
In the above example, we make the t1 thread enter the waiting state through the lock.wait() method, Until the t2 thread notifies it through the lock.notify() method.
5. Terminated state (Terminated)
When a thread completes its task or exits due to an exception, it enters the terminated state. A thread in the terminated state is no longer executing and cannot be started again.
The following is a simple code example showing the termination status of a thread:
public class Main { public static void main(String[] args) { Thread t1 = new Thread(() -> { for(int i=0; i<10; i++){ System.out.println(Thread.currentThread().getName() + ": " + i); } }); t1.start(); try { t1.join(); // 确保线程执行完 } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Thread 1 is terminated"); } }
In the above example, we created a thread t1 and started it. Then use the t1.join() method to ensure that the thread continues to execute subsequent code after it has finished executing.
To sum up, this article introduces the five states of Java threads and their characteristics and performance in a multi-threaded environment. For multi-threaded programming, it is crucial to understand the transitions and characteristics of thread states. Using appropriate thread states can make the program more efficient and reliable. I hope that through the introduction of this article, readers can better understand the working mechanism of Java threads and correctly use multi-threaded programming in actual projects.
The above is the detailed content of A detailed description of the five states of Java threads and their characteristics and performance in multi-threaded environments. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


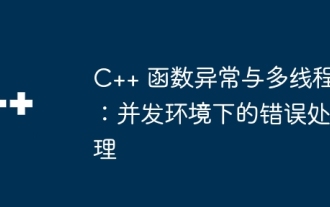
Function exception handling in C++ is particularly important for multi-threaded environments to ensure thread safety and data integrity. The try-catch statement allows you to catch and handle specific types of exceptions when they occur to prevent program crashes or data corruption.
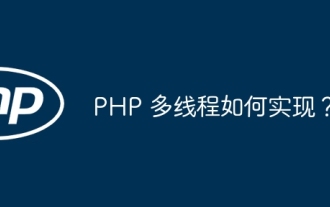
PHP multithreading refers to running multiple tasks simultaneously in one process, which is achieved by creating independently running threads. You can use the Pthreads extension in PHP to simulate multi-threading behavior. After installation, you can use the Thread class to create and start threads. For example, when processing a large amount of data, the data can be divided into multiple blocks and a corresponding number of threads can be created for simultaneous processing to improve efficiency.
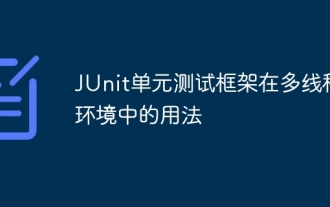
There are two common approaches when using JUnit in a multi-threaded environment: single-threaded testing and multi-threaded testing. Single-threaded tests run on the main thread to avoid concurrency issues, while multi-threaded tests run on worker threads and require a synchronized testing approach to ensure shared resources are not disturbed. Common use cases include testing multi-thread-safe methods, such as using ConcurrentHashMap to store key-value pairs, and concurrent threads to operate on the key-value pairs and verify their correctness, reflecting the application of JUnit in a multi-threaded environment.
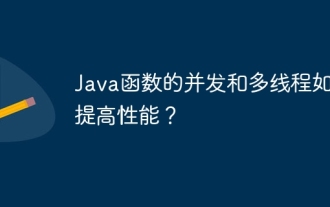
Concurrency and multithreading techniques using Java functions can improve application performance, including the following steps: Understand concurrency and multithreading concepts. Leverage Java's concurrency and multi-threading libraries such as ExecutorService and Callable. Practice cases such as multi-threaded matrix multiplication to greatly shorten execution time. Enjoy the advantages of increased application response speed and optimized processing efficiency brought by concurrency and multi-threading.
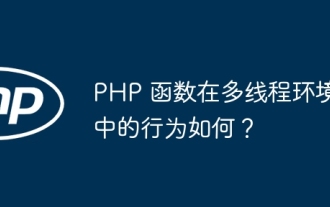
In a multi-threaded environment, the behavior of PHP functions depends on their type: Normal functions: thread-safe, can be executed concurrently. Functions that modify global variables: unsafe, need to use synchronization mechanism. File operation function: unsafe, need to use synchronization mechanism to coordinate access. Database operation function: Unsafe, database system mechanism needs to be used to prevent conflicts.
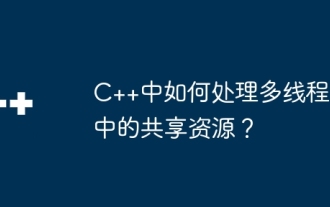
Mutexes are used in C++ to handle multi-threaded shared resources: create mutexes through std::mutex. Use mtx.lock() to obtain a mutex and provide exclusive access to shared resources. Use mtx.unlock() to release the mutex.
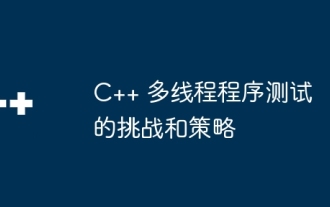
Multi-threaded program testing faces challenges such as non-repeatability, concurrency errors, deadlocks, and lack of visibility. Strategies include: Unit testing: Write unit tests for each thread to verify thread behavior. Multi-threaded simulation: Use a simulation framework to test your program with control over thread scheduling. Data race detection: Use tools to find potential data races, such as valgrind. Debugging: Use a debugger (such as gdb) to examine the runtime program status and find the source of the data race.
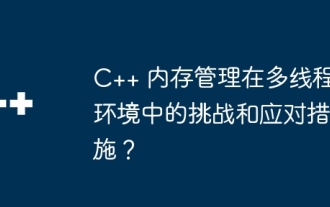
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.
