Front-end programming tool: Promise solves asynchronous problems
Front-end Promise: A powerful tool to solve asynchronous programming problems, specific code examples are required
1. Introduction
In front-end development, we often encounter Situations that require asynchronous operations, such as sending requests to obtain data, file reading, timers, etc. Asynchronous programming often leads to code logic that is complex and difficult to maintain. In order to solve this problem, JavaScript introduced the Promise object, which became a powerful tool for handling asynchronous operations. This article will introduce the basic concepts and common methods of Promise, and use specific code examples to demonstrate the power of Promise in solving asynchronous programming problems.
2. The basic concept of Promise
Promise is an object used to process asynchronous operations. It can be regarded as a container, which stores the results of asynchronous operations. A Promise object has three states: pending (in progress), fulfilled (successful) and rejected (failed). When the asynchronous operation is completed, the state of the Promise object will change from pending to fulfilled or rejected.
3. Common methods of Promise
- Promise.resolve(value)
The Promise.resolve method returns a new Promise object whose status is fulfilled and carries The specified value value. - Promise.reject(reason)
The Promise.reject method returns a new Promise object whose status is rejected and carries the specified error message reason. - Promise.prototype.then(onFulfilled, onRejected)
The Promise.prototype.then method is used to specify the callback function when the state of the Promise object changes. It receives two parameters: onFulfilled and onRejected, which respectively represent the callback when the asynchronous operation succeeds and the callback when it fails. - Promise.prototype.catch(onRejected)
The Promise.prototype.catch method is used to catch errors in asynchronous operations. It is equivalent to .then(null, onRejected).
5. Specific code examples
In order to better understand the usage of Promise, let’s look at a specific example. Suppose there is a requirement: after the user clicks a button, the server is asynchronously requested to return data, and then the next step is decided based on the result of the returned data. The following is a code example using Promise:
// 模拟异步请求 function fetchData() { return new Promise((resolve, reject) => { setTimeout(() => { const data = { message: 'Hello World' }; resolve(data); }, 2000); }); } // 用户点击按钮后的操作 function handleClick() { fetchData() .then((response) => { console.log(response.message); // 根据返回数据的结果决定下一步的操作 if (response.message === 'Hello World') { return Promise.resolve('操作成功'); } else { throw new Error('操作失败'); } }) .then((result) => { console.log(result); }) .catch((error) => { console.error(error); }); } // 用户点击按钮时触发 document.querySelector('button').addEventListener('click', handleClick);
In the above code, the fetchData function simulates an asynchronous request and it returns a Promise object. When the user clicks the button, the data is obtained by calling the fetchData function. In the then method, we can decide the next step based on the returned data. If the message field in the returned data is 'Hello World', a Promise object with a fulfilled status is returned and the result of "operation successful" is printed; if not, an error is thrown and the error is captured in the catch method.
This example demonstrates Promise's ability to handle asynchronous operations. We can chain multiple asynchronous operations by calling the then method, and catch errors through the catch method, making the code logic clear and easy to maintain.
6. Summary
This article introduces the basic concepts and common methods of front-end Promise, and demonstrates the advantages of Promise in solving asynchronous programming problems through a specific code example. By using Promises, we can better handle asynchronous operations and avoid callback hell and code clutter. I hope that through the introduction of this article, readers can have a deeper understanding of Promise and use it flexibly in actual development.
The above is the detailed content of Front-end programming tool: Promise solves asynchronous problems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


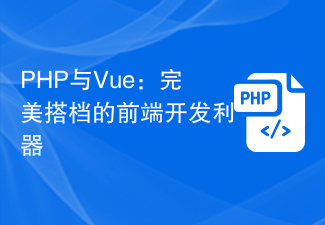
PHP and Vue: a perfect pairing of front-end development tools. In today's era of rapid development of the Internet, front-end development has become increasingly important. As users have higher and higher requirements for the experience of websites and applications, front-end developers need to use more efficient and flexible tools to create responsive and interactive interfaces. As two important technologies in the field of front-end development, PHP and Vue.js can be regarded as perfect tools when paired together. This article will explore the combination of PHP and Vue, as well as detailed code examples to help readers better understand and apply these two
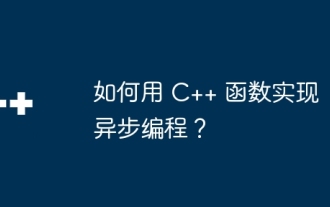
Summary: Asynchronous programming in C++ allows multitasking without waiting for time-consuming operations. Use function pointers to create pointers to functions. The callback function is called when the asynchronous operation completes. Libraries such as boost::asio provide asynchronous programming support. The practical case demonstrates how to use function pointers and boost::asio to implement asynchronous network requests.
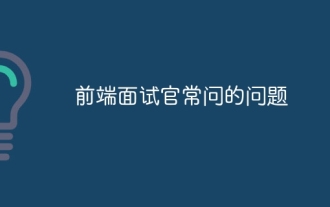
In front-end development interviews, common questions cover a wide range of topics, including HTML/CSS basics, JavaScript basics, frameworks and libraries, project experience, algorithms and data structures, performance optimization, cross-domain requests, front-end engineering, design patterns, and new technologies and trends. . Interviewer questions are designed to assess the candidate's technical skills, project experience, and understanding of industry trends. Therefore, candidates should be fully prepared in these areas to demonstrate their abilities and expertise.
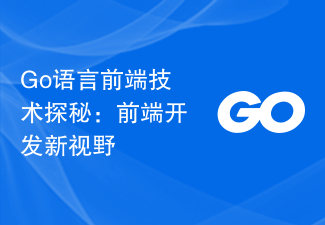
As a fast and efficient programming language, Go language is widely popular in the field of back-end development. However, few people associate Go language with front-end development. In fact, using Go language for front-end development can not only improve efficiency, but also bring new horizons to developers. This article will explore the possibility of using the Go language for front-end development and provide specific code examples to help readers better understand this area. In traditional front-end development, JavaScript, HTML, and CSS are often used to build user interfaces
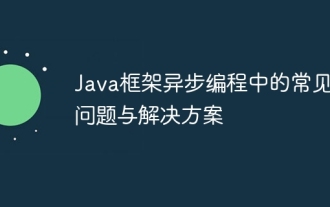
3 common problems and solutions in asynchronous programming in Java frameworks: Callback Hell: Use Promise or CompletableFuture to manage callbacks in a more intuitive style. Resource contention: Use synchronization primitives (such as locks) to protect shared resources, and consider using thread-safe collections (such as ConcurrentHashMap). Unhandled exceptions: Explicitly handle exceptions in tasks and use an exception handling framework (such as CompletableFuture.exceptionally()) to handle exceptions.
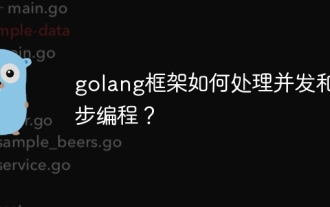
The Go framework uses Go's concurrency and asynchronous features to provide a mechanism for efficiently handling concurrent and asynchronous tasks: 1. Concurrency is achieved through Goroutine, allowing multiple tasks to be executed at the same time; 2. Asynchronous programming is implemented through channels, which can be executed without blocking the main thread. Task; 3. Suitable for practical scenarios, such as concurrent processing of HTTP requests, asynchronous acquisition of database data, etc.
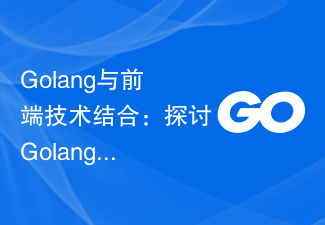
Combination of Golang and front-end technology: To explore how Golang plays a role in the front-end field, specific code examples are needed. With the rapid development of the Internet and mobile applications, front-end technology has become increasingly important. In this field, Golang, as a powerful back-end programming language, can also play an important role. This article will explore how Golang is combined with front-end technology and demonstrate its potential in the front-end field through specific code examples. The role of Golang in the front-end field is as an efficient, concise and easy-to-learn
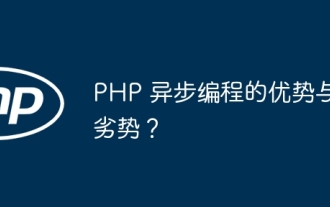
The advantages of asynchronous programming in PHP include higher throughput, lower latency, better resource utilization, and scalability. Disadvantages include complexity, difficulty in debugging, and limited library support. In the actual case, ReactPHP is used to handle WebSocket connections, demonstrating the practical application of asynchronous programming.
