Method to achieve front-end and back-end interaction: use ajax
Title: Ajax realizes front-end and back-end interaction and code examples
Introduction:
Ajax (Asynchronous JavaScript and XML) is a way to implement front-end and back-end interaction in Web applications End-to-end interaction technology. By using Ajax, the front-end page can exchange data with the back-end server without refreshing, which greatly improves the user experience and the response speed of the web page. This article will introduce how to use Ajax to achieve front-end and back-end interaction, and provide specific code examples.
1. The basic principle of Ajax
The basic principle of Ajax is to use the browser's XMLHttpRequest object for communication. When the page needs to obtain data from the server, it creates an XMLHttpRequest object and initiates an asynchronous request to the server. After the server receives the request, it processes the data and returns the results to the front-end page in the form of XML, JSON, etc. The front-end page then processes the returned data through the callback function to dynamically update the page content.
2. Ajax workflow
- Create an XMLHttpRequest object: Create an XMLHttpRequest object in JavaScript and initiate a request to the server through it.
- Send a request to the server: Call the open method of the XMLHttpRequest object to define parameters such as the request method, URL, and whether it is asynchronous, and then call the send method to send the request.
- Server-side processing request: After receiving the request, the server-side performs corresponding data processing and logical operations.
- Return data to the front-end page: After the server processes the request, the result is returned to the front-end page in the form of XML, JSON, etc.
- Front-end page processing returns data: The front-end processes the data returned by the server through the callback function and updates the page content as needed.
3. Ajax implementation example
The following is an example of using Ajax to achieve front-end and back-end interaction. Suppose we need to implement a simple login function.
- Introduce the jQuery library into the front-end page to use the ajax method it provides. You can add the following code in the head tag:
<script src="https://cdn.bootcdn.net/ajax/libs/jquery/3.6.0/jquery.min.js"></script>
- Create a login form in the HTML page and add the id to obtain the form data. The sample code is as follows:
<form id="loginForm"> <input type="text" id="username" name="username" placeholder="请输入用户名"> <input type="password" id="password" name="password" placeholder="请输入密码"> <button type="submit">登录</button> </form> <div id="result"></div>
- Send a login request through Ajax in JavaScript and process the data returned by the server. The sample code is as follows:
$(document).ready(function() { $('#loginForm').submit(function(event) { event.preventDefault(); // 阻止表单默认提交事件 // 获取输入框的值 var username = $('#username').val(); var password = $('#password').val(); // 发送Ajax请求 $.ajax({ type: 'POST', url: 'login.php', // 后端处理登录的接口地址 data: { username: username, password: password }, success: function(response) { // 处理服务器返回的数据 if (response.success) { $('#result').text('登录成功'); } else { $('#result').text('登录失败,请检查用户名和密码'); } }, error: function(xhr, status, error) { // 处理请求错误 $('#result').text('请求失败,请稍后重试'); } }); }); });
- Handle the login request in the backend server. The example uses PHP to implement back-end logic. The example code is as follows (login.php):
<?php $username = $_POST['username']; $password = $_POST['password']; // 在这里编写登录验证的逻辑 // ... // 假设登录验证结果保存在$success中 $success = true; // 返回登录结果 $response = array('success' => $success); echo json_encode($response); ?>
Through the above code example, we can implement a simple login function. When the user enters the user name and password on the front-end page and clicks the login button, the login request is sent to the back-end for processing through Ajax. The back-end verifies the user name and password and returns the login result to the front-end. The front-end page updates and displays corresponding prompt information based on the login result.
Conclusion:
Realizing front-end and back-end interaction through Ajax can realize partial page updates, improve the user's interactive experience and the page's response speed. Through the code examples provided in this article, you can learn how to use Ajax to implement front-end and back-end interaction and process return data. In actual development, you can flexibly use Ajax technology to implement more complex functions according to specific needs.
The above is the detailed content of Method to achieve front-end and back-end interaction: use ajax. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


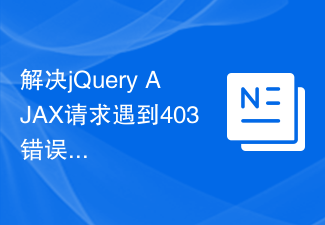
Title: Methods and code examples to resolve 403 errors in jQuery AJAX requests. The 403 error refers to a request that the server prohibits access to a resource. This error usually occurs because the request lacks permissions or is rejected by the server. When making jQueryAJAX requests, you sometimes encounter this situation. This article will introduce how to solve this problem and provide code examples. Solution: Check permissions: First ensure that the requested URL address is correct and verify that you have sufficient permissions to access the resource.
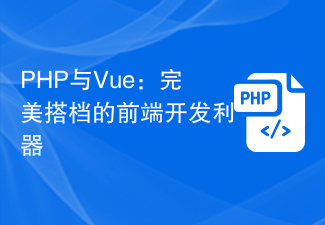
PHP and Vue: a perfect pairing of front-end development tools. In today's era of rapid development of the Internet, front-end development has become increasingly important. As users have higher and higher requirements for the experience of websites and applications, front-end developers need to use more efficient and flexible tools to create responsive and interactive interfaces. As two important technologies in the field of front-end development, PHP and Vue.js can be regarded as perfect tools when paired together. This article will explore the combination of PHP and Vue, as well as detailed code examples to help readers better understand and apply these two
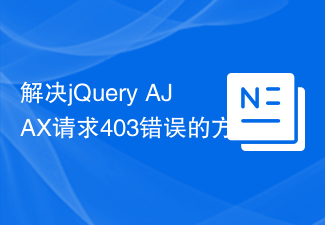
jQuery is a popular JavaScript library used to simplify client-side development. AJAX is a technology that sends asynchronous requests and interacts with the server without reloading the entire web page. However, when using jQuery to make AJAX requests, you sometimes encounter 403 errors. 403 errors are usually server-denied access errors, possibly due to security policy or permission issues. In this article, we will discuss how to resolve jQueryAJAX request encountering 403 error
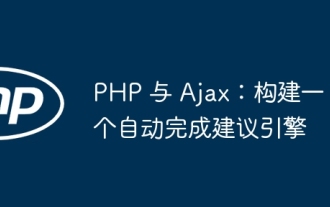
Build an autocomplete suggestion engine using PHP and Ajax: Server-side script: handles Ajax requests and returns suggestions (autocomplete.php). Client script: Send Ajax request and display suggestions (autocomplete.js). Practical case: Include script in HTML page and specify search-input element identifier.
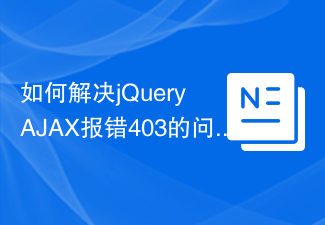
How to solve the problem of jQueryAJAX error 403? When developing web applications, jQuery is often used to send asynchronous requests. However, sometimes you may encounter error code 403 when using jQueryAJAX, indicating that access is forbidden by the server. This is usually caused by server-side security settings, but there are ways to work around it. This article will introduce how to solve the problem of jQueryAJAX error 403 and provide specific code examples. 1. to make
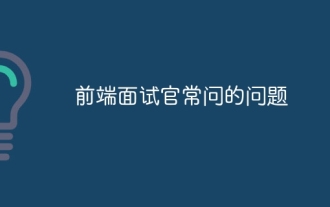
In front-end development interviews, common questions cover a wide range of topics, including HTML/CSS basics, JavaScript basics, frameworks and libraries, project experience, algorithms and data structures, performance optimization, cross-domain requests, front-end engineering, design patterns, and new technologies and trends. . Interviewer questions are designed to assess the candidate's technical skills, project experience, and understanding of industry trends. Therefore, candidates should be fully prepared in these areas to demonstrate their abilities and expertise.
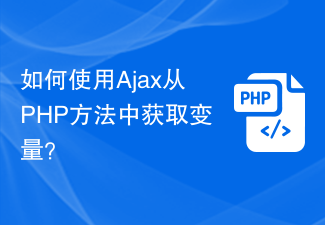
Using Ajax to obtain variables from PHP methods is a common scenario in web development. Through Ajax, the page can be dynamically obtained without refreshing the data. In this article, we will introduce how to use Ajax to get variables from PHP methods, and provide specific code examples. First, we need to write a PHP file to handle the Ajax request and return the required variables. Here is sample code for a simple PHP file getData.php:
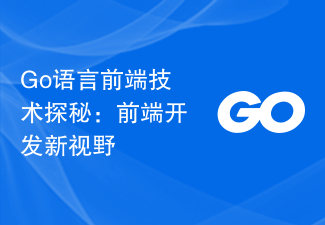
As a fast and efficient programming language, Go language is widely popular in the field of back-end development. However, few people associate Go language with front-end development. In fact, using Go language for front-end development can not only improve efficiency, but also bring new horizons to developers. This article will explore the possibility of using the Go language for front-end development and provide specific code examples to help readers better understand this area. In traditional front-end development, JavaScript, HTML, and CSS are often used to build user interfaces
