


PHP SPL data structures: a toolkit to give your code a new look
PHP SPL Data Structure: Overview
In PHP development, data structure is a crucial aspect, which directly affects the efficiency and readability of the code. The PHP SPL (Standard PHP Library) data structure provides a rich toolkit that can help developers process data more efficiently and improve code quality. In this article, PHP editor Yuzai will introduce you to the PHP SPL data structure, which will give your code a new look and improve development efficiency and code quality.
Main data structure
Stack
A stack is an ordered collection that follows the last-in-first-out (LIFO) principle. In the stack, the last element added will be the first element removed. SPL provides a SplStack
class to represent a stack. The following example shows how to use SplStack
:
$stack = new SplStack(); $stack->push(1); $stack->push(2); $stack->push(3); // 访问堆栈的最后一个元素 echo $stack->top() . " "; // 输出:3 // 弹出堆栈的最后一个元素 $stack->pop(); // 检查堆栈是否为空 if ($stack->isEmpty()) { echo "堆栈为空" . " "; }
queue
A queue is an ordered collection that follows the first-in, first-out (FIFO) principle. In the queue, the first element added will be the first element removed. SPL provides a SplQueue
class to represent a queue. The following example shows how to use SplQueue
:
$queue = new SplQueue(); $queue->enqueue(1); $queue->enqueue(2); $queue->enqueue(3); // 访问队列的第一个元素 echo $queue->bottom() . " "; // 输出:1 // 出队队列的第一个元素 $queue->dequeue(); // 检查队列是否为空 if ($queue->isEmpty()) { echo "队列为空" . " "; }
Array
SPL provides a SplFixedArray
class that represents a fixed-size array. Unlike standard PHP arrays, SplFixedArray
specifies its size when created and cannot be resized dynamically. This restriction improves performance while preventing accidental array modifications.
$fixedArray = new SplFixedArray(3); $fixedArray[0] = 1; $fixedArray[1] = 2; $fixedArray[2] = 3; // 访问数组元素 echo $fixedArray[1] . " "; // 输出:2 // 尝试设置超出范围的数组元素 try { $fixedArray[3] = 4; } catch (OutOfRangeException $e) { echo "元素索引超出范围" . " "; }
Hash table
SPL provides a SplObjectStorage
class, which represents a hash table, which is an unordered collection of key-value pairs. Both keys and values can be objects.
$objectStorage = new SplObjectStorage(); $objectStorage->attach($object1, "值1"); $objectStorage->attach($object2, "值2"); // 访问哈希表的值 echo $objectStorage[$object1] . " "; // 输出:"值1" // 检查哈希表是否包含键 if ($objectStorage->contains($object2)) { echo "哈希表包含键 $object2" . " "; }
Advantage
Using PHP SPL data structures provides the following main advantages:
- Consistency: The SPL data structure provides a standardized and consistent interface that simplifies data processing regardless of data type.
- Performance Optimization: These data structures are optimized to efficiently handle large data sets, improving overall application performance.
- Concise code: Using SPL data structure can reduce code duplication and make the code more concise and readable.
- Scalability: SPL data structures can be easily integrated into existing code, supporting future scalability of applications.
in conclusion
PHP SPL Data Structures is a powerful tools package that helps developers create efficient, scalable, and easy-to-maintain applications. By providing standardized and consistent data structures, SPL significantly improves code organization, performance, and readability. Therefore, the use of PHP SPL data structures is highly recommended for developers who need to handle complex data and optimize application performance.
The above is the detailed content of PHP SPL data structures: a toolkit to give your code a new look. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


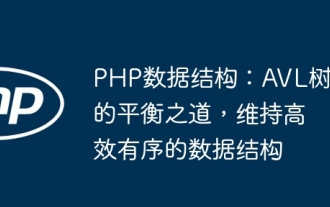
AVL tree is a balanced binary search tree that ensures fast and efficient data operations. To achieve balance, it performs left- and right-turn operations, adjusting subtrees that violate balance. AVL trees utilize height balancing to ensure that the height of the tree is always small relative to the number of nodes, thereby achieving logarithmic time complexity (O(logn)) search operations and maintaining the efficiency of the data structure even on large data sets.
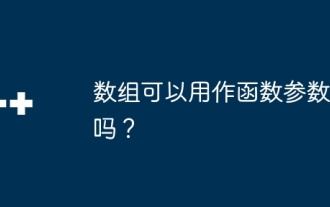
Yes, in many programming languages, arrays can be used as function parameters, and the function will perform operations on the data stored in it. For example, the printArray function in C++ can print the elements in an array, while the printArray function in Python can traverse the array and print its elements. Modifications made to the array by these functions are also reflected in the original array in the calling function.
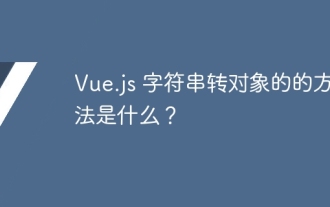
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.
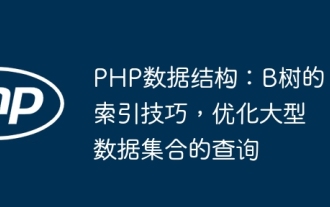
B-tree is a balanced search tree used for fast storage and retrieval of data. The performance of B-tree indexes can be optimized using joint indexes, prefix indexes and the correct balancing strategy. Specifically, choosing the appropriate order, using union indexes, using prefix indexes, and choosing the right balancing strategy can significantly improve the performance of B-tree indexes.
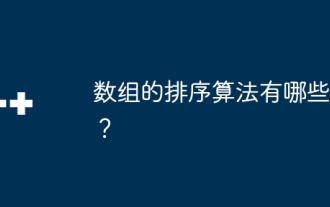
Array sorting algorithms are used to arrange elements in a specific order. Common types of algorithms include: Bubble sort: swap positions by comparing adjacent elements. Selection sort: Find the smallest element and swap it to the current position. Insertion sort: Insert elements one by one to the correct position. Quick sort: divide and conquer method, select the pivot element to divide the array. Merge Sort: Divide and Conquer, Recursive Sorting and Merging Subarrays.
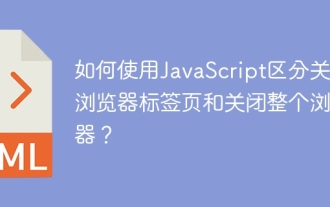
How to distinguish between closing tabs and closing entire browser using JavaScript on your browser? During the daily use of the browser, users may...
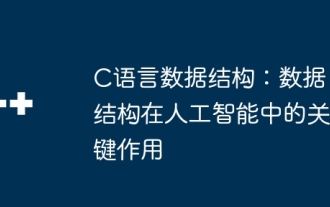
C Language Data Structure: Overview of the Key Role of Data Structure in Artificial Intelligence In the field of artificial intelligence, data structures are crucial to processing large amounts of data. Data structures provide an effective way to organize and manage data, optimize algorithms and improve program efficiency. Common data structures Commonly used data structures in C language include: arrays: a set of consecutively stored data items with the same type. Structure: A data type that organizes different types of data together and gives them a name. Linked List: A linear data structure in which data items are connected together by pointers. Stack: Data structure that follows the last-in first-out (LIFO) principle. Queue: Data structure that follows the first-in first-out (FIFO) principle. Practical case: Adjacent table in graph theory is artificial intelligence
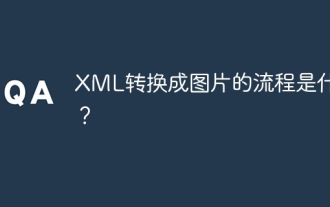
To convert XML images, you need to determine the XML data structure first, then select a suitable graphical library (such as Python's matplotlib) and method, select a visualization strategy based on the data structure, consider the data volume and image format, perform batch processing or use efficient libraries, and finally save it as PNG, JPEG, or SVG according to the needs.
