


Learn more about JVM virtual machines and improve Java development capabilities
To understand the role of the JVM virtual machine and improve Java development skills, specific code examples are needed
JVM (Java Virtual Machine) is one of the core components of the Java platform. Provides an environment for running Java bytecode. The role of the JVM is to compile Java source code into Java bytecode and is responsible for executing the bytecode at runtime. By understanding the internal mechanism of the JVM, you can better understand the running process of Java programs and be able to optimize and tune Java applications.
The main functions of JVM are as follows:
- Cross-platform: One of the biggest advantages of JVM is its cross-platform nature. Since the JVM can run on different operating systems and provides an operating system-independent abstraction layer, the same Java bytecode can be used to run on different platforms. This provides great convenience to Java developers without having to write specific code for each platform.
- Memory management: JVM is responsible for the memory management of Java programs. It uses a garbage collection mechanism to automatically manage memory, so developers no longer need to manually allocate and release memory. By using the garbage collector provided by the JVM, Java programs can use memory resources more efficiently and reduce the risk of memory leaks and memory overflows.
- Exception handling: JVM provides a unified exception handling mechanism for Java programs. By catching and handling exceptions, programs can better respond to errors and exceptions, and ensure program stability and robustness. The exception handling mechanism provided by the JVM can make it easier for developers to locate and solve problems.
The following are some code examples that can help us understand the role and mechanism of the JVM more deeply.
- Cross-platform example:
public class HelloWorld { public static void main(String[] args) { System.out.println("Hello, World!"); } }
In the above code, we wrote a simple Hello World program in Java. By using JVM, we can compile this program into bytecode and run it on different operating systems. Whether on Windows, Linux or Mac OS, you can get the same output by executing the same bytecode.
- Garbage collection example:
public class MemoryExample { public static void main(String[] args) { for (int i = 0; i < 100000; i++) { String temp = new String("Hello World"); System.out.println(temp); } } }
In the above code, we use a loop to create 100000 string objects and output each string. Since the JVM automatically handles memory management, we do not need to manually release the memory occupied by these objects. When the object is no longer referenced, the JVM will automatically reclaim the memory, thus avoiding the problem of memory leaks.
- Exception handling example:
public class ExceptionExample { public static void main(String[] args) { try { int result = divide(10, 0); System.out.println("Result: " + result); } catch (ArithmeticException e) { System.out.println("Error: " + e.getMessage()); } } public static int divide(int num1, int num2) { return num1 / num2; } }
In the above code, we handle the division by zero situation through the exception handling mechanism. When an exception occurs during the division operation, the JVM will throw an ArithmeticException
exception and catch the exception through the catch
block. This allows us to perform specific error handling logic when an exception occurs.
Through the above code examples, we can see the importance and role of JVM in Java development. Understanding the internal mechanisms of the JVM and how to use the features and mechanisms provided by the JVM can not only improve Java development skills, but also optimize and tune Java applications, thereby improving the performance and stability of the program. Therefore, in-depth study and understanding of the JVM is an important ability that every Java developer should master.
The above is the detailed content of Learn more about JVM virtual machines and improve Java development capabilities. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
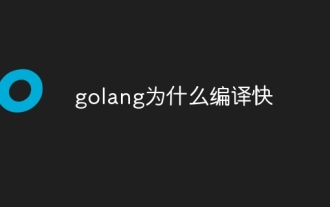
Go has the advantage of fast compilation due to factors such as parallel compilation, incremental compilation, simple syntax, efficient data structures, precompiled headers, garbage collection, and other optimizations.
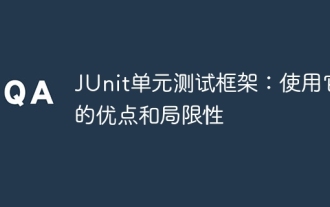
The JUnit unit testing framework is a widely used tool whose main advantages include automated testing, fast feedback, improved code quality, and portability. But it also has limitations, including limited scope, maintenance costs, dependencies, memory consumption, and lack of continuous integration support. For unit testing of Java applications, JUnit is a powerful framework that offers many benefits, but its limitations need to be considered when using it.
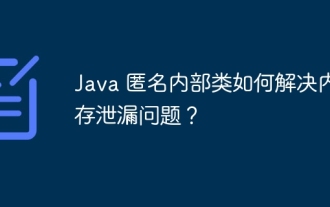
Anonymous inner classes can cause memory leaks. The problem is that they hold a reference to the outer class, preventing the outer class from being garbage collected. Solutions include: 1. Use weak references. When the external class is no longer held by a strong reference, the garbage collector will immediately recycle the weak reference object; 2. Use soft references. The garbage collector will recycle the weak reference object when it needs memory during garbage collection. Only then the soft reference object is recycled. In actual combat, such as in Android applications, the memory leak problem caused by anonymous inner classes can be solved by using weak references, so that the anonymous inner class can be recycled when the listener is not needed.
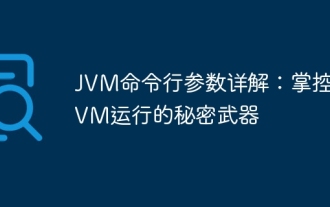
JVM command line parameters allow you to adjust JVM behavior at a fine-grained level. The common parameters include: Set the Java heap size (-Xms, -Xmx) Set the new generation size (-Xmn) Enable the parallel garbage collector (-XX:+UseParallelGC) Reduce the memory usage of the Survivor area (-XX:-ReduceSurvivorSetInMemory) Eliminate redundancy Eliminate garbage collection (-XX:-EliminateRedundantGCs) Print garbage collection information (-XX:+PrintGC) Use the G1 garbage collector (-XX:-UseG1GC) Set the maximum garbage collection pause time (-XX:MaxGCPau
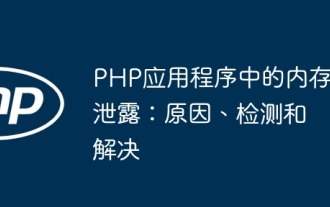
A PHP memory leak occurs when an application allocates memory and fails to release it, resulting in a reduction in the server's available memory and performance degradation. Causes include circular references, global variables, static variables, and expansion. Detection methods include Xdebug, Valgrind and PHPUnitMockObjects. The resolution steps are: identify the source of the leak, fix the leak, test and monitor. Practical examples illustrate memory leaks caused by circular references, and specific methods to solve the problem by breaking circular references through destructors.
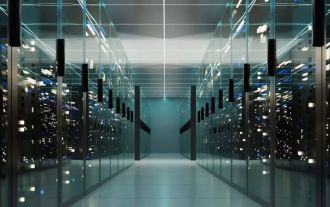
According to news from this site on May 24, Broadcom carried out drastic reforms after acquiring VMware. It sold non-core businesses such as the end-user computing department for US$4 billion, ended 59 products, and focused on providing support for large enterprises. The subscription method attracts enterprises to adopt. Technology media Techspot reported that large enterprises may not be able to afford VMware's price increases. Computershare, an Australian company with 24,000 virtual machines, may abandon VMware and focus on Nutanix products. Note from this site: Computershare mainly provides financial products and investor services to stock exchanges around the world. After Broadcom acquired VMware
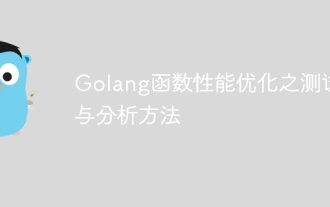
Optimizing function performance in Go is crucial. Functions can be tested and analyzed using performance analysis tools and benchmarks: Benchmark: Use the Benchmark function to compare the performance of function implementations. Performance analysis: Use tools in the pprof package (such as CPUProfile) to generate performance analysis configuration files. Practical case: Analyze the Add function to find performance bottlenecks, and optimize the function through external loops. Optimization tips: use efficient data structures, reduce allocations, parallelize execution, and disable the garbage collector.
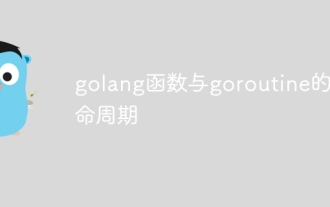
Function Lifecycle: Declaration and Compilation: The compiler verifies the syntax and type of the function. Execution: Executed when the function is called. Return: Return to the calling location after execution. Goroutine life cycle: Creation and startup: Create and start through the go keyword. Execution: Runs asynchronously until the task is completed. End: The task ends when it is completed or an error occurs. Cleanup: The garbage collector cleans up the memory occupied by the completed Goroutine.
