


Demystifying the Java Memory Model: Mastering the Secrets Behind Multi-Threaded Programming
php Xiaobian Yuzai takes you to reveal the Java memory model and gain an in-depth understanding of the mysteries behind multi-threaded programming. Multi-threaded programming is an important skill in Java development, and understanding the memory model is crucial to writing efficient and reliable multi-threaded programs. Let's explore the Java memory model together and uncover the mystery of multi-threaded programming!
The main goal of JMM is to ensure the correctness and predictability of multi-threaded programs. It prevents data races and memory consistency issues by defining a set of rules to regulate thread access to shared memory. The basic principles of JMM include:
- Visibility: Modifications of shared variables by threads must be reflected in the visible range of other threads in a timely manner.
- Atomicity: Read and write operations on shared variables are atomic, that is, uninterruptible.
- Ordering: The order of thread access to shared variables must be consistent with the order of execution in the program.
In order to realize these basic principles, JMM introduces the following key concepts:
- Main memory (main memory): Main memory is the physical memory space shared by all threads.
- Working memory: Each thread has its own working memory, which stores a copy of the thread's private variables.
- cache coherence protocol: Cache The coherence protocol is a protocol used to ensure that data in the caches of multiple processors remains consistent.
When a thread modifies a shared variable, it writes the modified value to main memory. Other threads can get the latest value by reading the value in main memory. However, other threads may not see the modified value immediately due to the latency of the cache coherence protocol. To solve this problem, JMM introduces the concept of memory barrier. A memory barrier forces a thread to immediately write modified values to main memory and ensures that other threads can see the modified values.
The Java language provides two keywords, synchronized
and volatile
, to achieve thread synchronization and visibility. The synchronized
keyword can ensure that access to shared variables is atomic, and the volatile
keyword can ensure that modifications to shared variables are visible.
Here is some demo code showing how to use the synchronized
and volatile
keywords to achieve thread synchronization and visibility:
class SharedCounter { private int count = 0; public synchronized void increment() { count++; } public int getCount() { return count; } } public class Main { public static void main(String[] args) { SharedCounter counter = new SharedCounter(); Thread thread1 = new Thread(() -> { for (int i = 0; i < 10000; i++) { counter.increment(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 10000; i++) { counter.increment(); } }); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } System.out.println("Final count: " + counter.getCount()); } }
In this example, we use the synchronized
keyword to ensure that access to the count
variable is atomic, thus avoiding data race issues.
class SharedCounter { private volatile int count = 0; public void increment() { count++; } public int getCount() { return count; } } public class Main { public static void main(String[] args) { SharedCounter counter = new SharedCounter(); Thread thread1 = new Thread(() -> { for (int i = 0; i < 10000; i++) { counter.increment(); } }); Thread thread2 = new Thread(() -> { for (int i = 0; i < 10000; i++) { System.out.println("Current count: " + counter.getCount()); } }); thread1.start(); thread2.start(); try { thread1.join(); thread2.join(); } catch (InterruptedException e) { e.printStackTrace(); } } }
In this example, we use the volatile
keyword to ensure that modifications to the count
variable are visible, so that Thread 2 can see Thread 1's changes to ## in a timely manner #count Modification of variables.
problems in concurrent programming . By mastering the basic principles and key concepts of JMM, programmers can write more robust and predictable multi-threaded programs.
The above is the detailed content of Demystifying the Java Memory Model: Mastering the Secrets Behind Multi-Threaded Programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


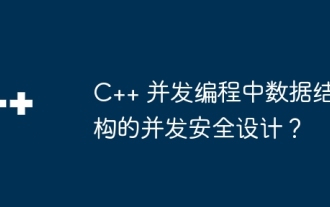
In C++ concurrent programming, the concurrency-safe design of data structures is crucial: Critical section: Use a mutex lock to create a code block that allows only one thread to execute at the same time. Read-write lock: allows multiple threads to read at the same time, but only one thread to write at the same time. Lock-free data structures: Use atomic operations to achieve concurrency safety without locks. Practical case: Thread-safe queue: Use critical sections to protect queue operations and achieve thread safety.
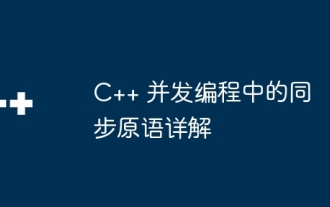
In C++ multi-threaded programming, the role of synchronization primitives is to ensure the correctness of multiple threads accessing shared resources. It includes: Mutex (Mutex): protects shared resources and prevents simultaneous access; Condition variable (ConditionVariable): thread Wait for specific conditions to be met before continuing execution; atomic operation: ensure that the operation is executed in an uninterruptible manner.
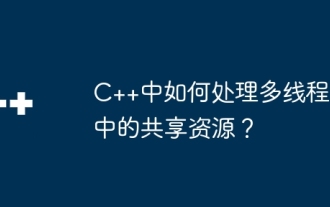
Mutexes are used in C++ to handle multi-threaded shared resources: create mutexes through std::mutex. Use mtx.lock() to obtain a mutex and provide exclusive access to shared resources. Use mtx.unlock() to release the mutex.
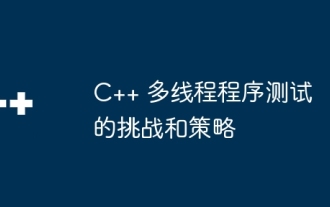
Multi-threaded program testing faces challenges such as non-repeatability, concurrency errors, deadlocks, and lack of visibility. Strategies include: Unit testing: Write unit tests for each thread to verify thread behavior. Multi-threaded simulation: Use a simulation framework to test your program with control over thread scheduling. Data race detection: Use tools to find potential data races, such as valgrind. Debugging: Use a debugger (such as gdb) to examine the runtime program status and find the source of the data race.
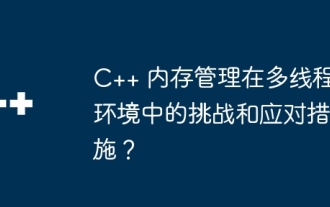
In a multi-threaded environment, C++ memory management faces the following challenges: data races, deadlocks, and memory leaks. Countermeasures include: 1. Use synchronization mechanisms, such as mutexes and atomic variables; 2. Use lock-free data structures; 3. Use smart pointers; 4. (Optional) implement garbage collection.

In multithreaded C++, exception handling follows the following principles: timeliness, thread safety, and clarity. In practice, you can ensure thread safety of exception handling code by using mutex or atomic variables. Additionally, consider reentrancy, performance, and testing of your exception handling code to ensure it runs safely and efficiently in a multi-threaded environment.
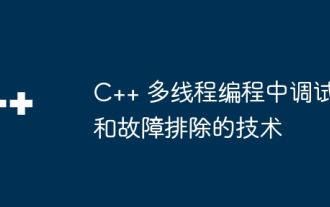
Debugging techniques for C++ multi-threaded programming include using a data race analyzer to detect read and write conflicts and using synchronization mechanisms (such as mutex locks) to resolve them. Use thread debugging tools to detect deadlocks and resolve them by avoiding nested locks and using deadlock detection mechanisms. Use the Data Race Analyzer to detect data races and resolve them by moving write operations into critical sections or using atomic operations. Use performance analysis tools to measure context switch frequency and resolve excessive overhead by reducing the number of threads, using thread pools, and offloading tasks.
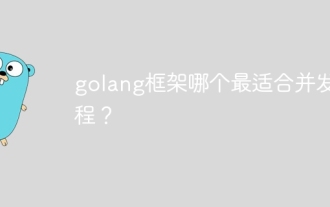
Golang concurrent programming framework guide: Goroutines: lightweight coroutines to achieve parallel operation; Channels: pipelines, used for communication between goroutines; WaitGroups: allows the main coroutine to wait for multiple goroutines to complete; Context: provides goroutine context information, such as cancellation and deadline.
