


Conquer the programming world with one move using Python Lambda expressions
Feb 19, 2024 pm 05:15 PMA Lambda expression is an anonymous function in python that allows you to pass a block of code as a parameter to another function. This allows you to write cleaner, more readable code and make it easier to combine code into more complex structures.
The syntax of Lambda expression is as follows:
lambda arguments : expression
Among them, arguments is the parameter list of the lambda expression, and expression is the code block of the lambda expression.
For example, the following code uses a lambda expression to calculate the sum of two numbers:
sum = lambda x, y: x + y
This lambda expression can be passed to another function as a parameter, for example:
def apply_function(function, x, y): return function(x, y) result = apply_function(sum, 1, 2)
This code snippet passes the lambda expression sum
to the function apply_function
as an argument and applies it to the numbers 1 and 2. The result will be stored in the variable result
.
Lambda expressions can also be used to operate on elements in lists or tuples. For example, the following code uses a lambda expression to square each element in a list:
numbers = [1, 2, 3, 4, 5] squared_numbers = list(map(lambda x: x ** 2, numbers))
This code snippet uses the built-in function map()
to apply the lambda expression lambda x: x ** 2
to each of the list numbers
element. The result is stored in the variable squared_numbers
.
Lambda expressions are a very powerful tool that can be used to write cleaner, more readable code. If you want to learn how to use lambda expressions, there are many resources to help you get started. There are many tutorials and articles about lambda expressions on the Internet, and you can also find more information about lambda expressions in the Python documentation.
Advantages of lambda expressions:
- Simple: lambda expressions are very simple. This makes them easy to read and understand.
- Anonymity: lambda expressions are anonymous. This means they don't have names, so you don't have to worry about naming conflicts.
- Readability: lambda expressions are very readable. This makes them easy to understand and maintain.
- Flexible: lambda expressions are very flexible. They can be used for a variety of purposes, including filtering, mapping, and reduction.
Disadvantages of lambda expressions:
- Difficult to debug: lambda expressions are difficult to debug. This is because they don't have names, so you can't trace them using a debugger.
- Not suitable for complex code: lambda expressions are not suitable for complex code. This is because they don't have names, so you can't break them into smaller parts.
in conclusion:
Lambda expression is a very powerful tool in Python. They can be used to write cleaner, more readable code. If you want to learn how to use lambda expressions, there are many resources to help you get started.The above is the detailed content of Conquer the programming world with one move using Python Lambda expressions. For more information, please follow other related articles on the PHP Chinese website!

Hot Article

Hot tools Tags

Hot Article

Hot Article Tags

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

What are the advantages and disadvantages of templating?
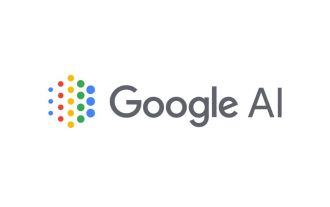
Google AI announces Gemini 1.5 Pro and Gemma 2 for developers
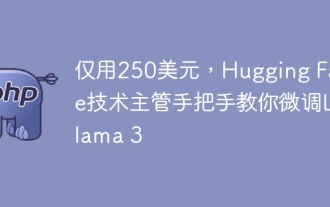
For only $250, Hugging Face's technical director teaches you how to fine-tune Llama 3 step by step
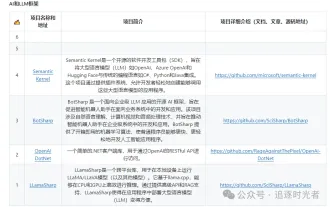
Share several .NET open source AI and LLM related project frameworks
