How many swing layouts are there in total?
Swing, as a development tool for creating graphical user interfaces, has a rich layout manager that can help us flexibly organize and arrange components. The following will introduce several commonly used layout managers in swing and provide corresponding code examples.
- BorderLayout (Border Layout Manager)
BorderLayout is one of the most commonly used layout managers in swing. It divides the container into five areas: north, south, east, west and center. Can be added to different areas by setting components.
The sample code is as follows:
import javax.swing.*; public class BorderLayoutExample { public static void main(String[] args) { JFrame frame = new JFrame("BorderLayout Example"); frame.setLayout(new BorderLayout()); JButton btnNorth = new JButton("North"); JButton btnSouth = new JButton("South"); JButton btnEast = new JButton("East"); JButton btnWest = new JButton("West"); JButton btnCenter = new JButton("Center"); frame.add(btnNorth, BorderLayout.NORTH); frame.add(btnSouth, BorderLayout.SOUTH); frame.add(btnEast, BorderLayout.EAST); frame.add(btnWest, BorderLayout.WEST); frame.add(btnCenter, BorderLayout.CENTER); frame.setSize(300, 200); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
- FlowLayout (Flow Layout Manager)
FlowLayout arranges the components in the order they are added. If the width of the container is not enough Accommodates all components and will automatically wrap and display.
The sample code is as follows:
import javax.swing.*; public class FlowLayoutExample { public static void main(String[] args) { JFrame frame = new JFrame("FlowLayout Example"); frame.setLayout(new FlowLayout()); JButton btn1 = new JButton("Button 1"); JButton btn2 = new JButton("Button 2"); JButton btn3 = new JButton("Button 3"); JButton btn4 = new JButton("Button 4"); frame.add(btn1); frame.add(btn2); frame.add(btn3); frame.add(btn4); frame.setSize(300, 200); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
- GridLayout (Grid Layout Manager)
GridLayout arranges the components in rows and columns, and all components are equal in size. If When the size of the container changes, the size of the component will be automatically adjusted.
The sample code is as follows:
import javax.swing.*; public class GridLayoutExample { public static void main(String[] args) { JFrame frame = new JFrame("GridLayout Example"); frame.setLayout(new GridLayout(3, 3)); for (int i = 1; i <= 9; i++) { JButton btn = new JButton("Button " + i); frame.add(btn); } frame.setSize(300, 200); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
- CardLayout (Card Layout Manager)
CardLayout superimposes multiple components on the same position, by switching different components Display different content, similar to flipping cards.
The sample code is as follows:
import javax.swing.*; import java.awt.*; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; public class CardLayoutExample { public static void main(String[] args) { JFrame frame = new JFrame("CardLayout Example"); frame.setLayout(new CardLayout()); JButton btn1 = new JButton("Card 1"); JButton btn2 = new JButton("Card 2"); JButton btn3 = new JButton("Card 3"); frame.add(btn1, "Card 1"); frame.add(btn2, "Card 2"); frame.add(btn3, "Card 3"); btn1.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { CardLayout cl = (CardLayout) frame.getContentPane().getLayout(); cl.show(frame.getContentPane(), "Card 2"); } }); btn2.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { CardLayout cl = (CardLayout) frame.getContentPane().getLayout(); cl.show(frame.getContentPane(), "Card 3"); } }); btn3.addActionListener(new ActionListener() { @Override public void actionPerformed(ActionEvent e) { CardLayout cl = (CardLayout) frame.getContentPane().getLayout(); cl.show(frame.getContentPane(), "Card 1"); } }); frame.setSize(300, 200); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.setVisible(true); } }
The above are several layout managers commonly used in swing and corresponding code examples. You can choose the appropriate layout manager to arrange components according to actual needs. . At the same time, we can also customize the layout manager to meet special needs by inheriting from LayoutManager.
The above is the detailed content of How many swing layouts are there in total?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










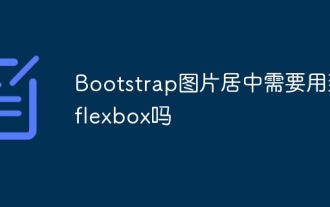
There are many ways to center Bootstrap pictures, and you don’t have to use Flexbox. If you only need to center horizontally, the text-center class is enough; if you need to center vertically or multiple elements, Flexbox or Grid is more suitable. Flexbox is less compatible and may increase complexity, while Grid is more powerful and has a higher learning cost. When choosing a method, you should weigh the pros and cons and choose the most suitable method according to your needs and preferences.
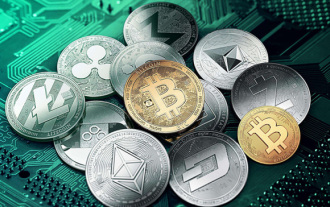
Top Ten Virtual Currency Trading Platforms 2025: 1. OKX, 2. Binance, 3. Gate.io, 4. Kraken, 5. Huobi, 6. Coinbase, 7. KuCoin, 8. Crypto.com, 9. Bitfinex, 10. Gemini. Security, liquidity, handling fees, currency selection, user interface and customer support should be considered when choosing a platform.
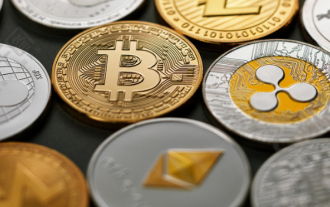
The top ten cryptocurrency trading platforms include: 1. OKX, 2. Binance, 3. Gate.io, 4. Kraken, 5. Huobi, 6. Coinbase, 7. KuCoin, 8. Crypto.com, 9. Bitfinex, 10. Gemini. Security, liquidity, handling fees, currency selection, user interface and customer support should be considered when choosing a platform.
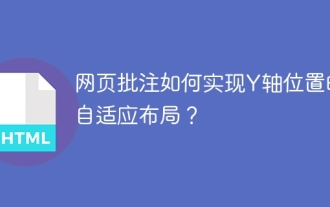
The Y-axis position adaptive algorithm for web annotation function This article will explore how to implement annotation functions similar to Word documents, especially how to deal with the interval between annotations...
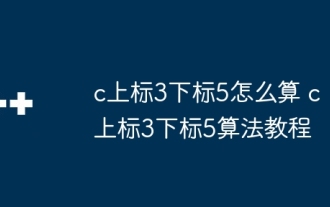
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
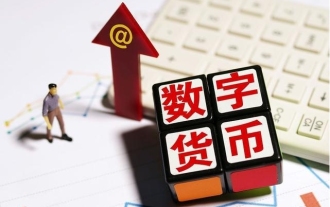
Recommended safe virtual currency software apps: 1. OKX, 2. Binance, 3. Gate.io, 4. Kraken, 5. Huobi, 6. Coinbase, 7. KuCoin, 8. Crypto.com, 9. Bitfinex, 10. Gemini. Security, liquidity, handling fees, currency selection, user interface and customer support should be considered when choosing a platform.
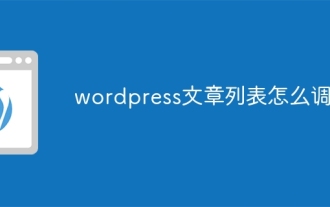
There are four ways to adjust the WordPress article list: use theme options, use plugins (such as Post Types Order, WP Post List, Boxy Stuff), use code (add settings in the functions.php file), or modify the WordPress database directly.
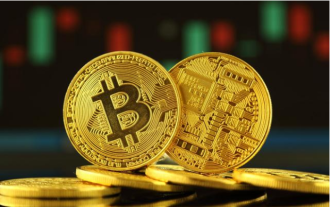
A safe and reliable digital currency platform: 1. OKX, 2. Binance, 3. Gate.io, 4. Kraken, 5. Huobi, 6. Coinbase, 7. KuCoin, 8. Crypto.com, 9. Bitfinex, 10. Gemini. Security, liquidity, handling fees, currency selection, user interface and customer support should be considered when choosing a platform.
