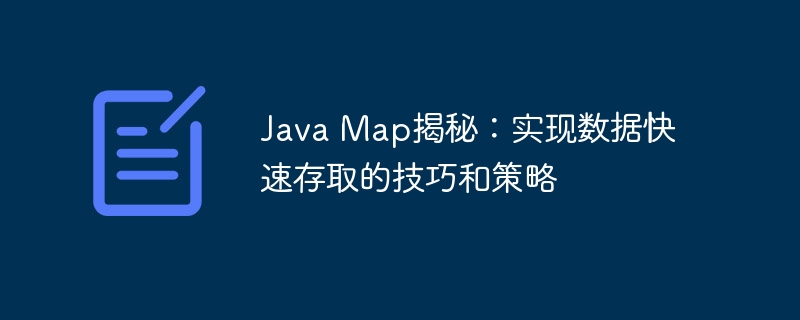
php Xiaobian Xigua will take you to have an in-depth understanding of Java Map and master the techniques and strategies for fast data access. The Map collection in Java is a data structure used to store key-value pairs, which can achieve efficient data access operations. Through in-depth study of the implementation principles of Map and different types of Map collections, it can help developers make better use of Map collections and improve code efficiency and performance. In this article, we will reveal the implementation details of Java Map and analyze the techniques and strategies for data access for you.
Map has two main implementations:
-
HashMap: Use a hash table to store key-value pairs. The performance of HashMap depends on how the hash table is implemented, and in most cases HashMap performs better than TreeMap.
- TreeMap: Use red-black trees to store key-value pairs. The performance of TreeMap is similar to HashMap, but in some cases, TreeMap may perform better than HashMap, such as when the keys are strings or numbers.
Tips and strategies for using Map
In order to optimize the performance of applications that use Map, developers can follow the following tips and strategies:
-
Choose an appropriate Map implementation: When choosing a Map implementation, developers need to consider the type of keys and the performance requirements of the application. If the keys are strings or numbers, you can use a TreeMap to improve performance. If the keys are other types of data, you can use a HashMap to improve performance.
-
Optimize Map keys: Map keys should select attributes that can uniquely identify values. If the key is not unique, it may cause duplicate data in the Map.
-
Use Map's get() method to retrieve data: Map's get() method is used to retrieve the value of a specified key. Developers should use the get() method to retrieve data instead of the [] operator because the performance of the get() method is better than the [] operator.
-
Use Map's put() method to store data: Map's put() method is used to store new key-value pairs or update the value of existing key-value pairs. Developers should use the put() method to store data instead of using the [] operator because the performance of the put() method is better than the [] operator.
-
Use Map's remove() method to delete data: Map's remove() method is used to delete key-value pairs of specified keys. Developers should use the remove() method to delete data instead of the clear() method because the performance of the remove() method is better than the clear() method.
Demo code
The following code demonstrates how to use Map:
import java.util.HashMap;
import java.util.Map;
public class Main {
public static void main(String[] args) {
// 创建一个Map
Map<String, Integer> map = new HashMap<>();
// 向Map中添加键值对
map.put("John", 25);
map.put("Mary", 30);
map.put("Bob", 35);
// 从Map中检索数据
Integer age = map.get("John");
// 更新Map中的数据
map.put("John", 30);
// 从Map中删除数据
map.remove("Bob");
// 遍历Map
for (Map.Entry<String, Integer> entry : map.entrySet()) {
System.out.println(entry.geTKEy() + ": " + entry.getValue());
}
}
}
Copy after login
This code creates a Map and adds three key-value pairs to the Map. The code then retrieves data from the Map, updates the data in the Map, deletes data from the Map, and iterates over the Map.
The above is the detailed content of Java Map Revealed: Tips and Strategies for Fast Data Access. For more information, please follow other related articles on the PHP Chinese website!