


A Deeper Understanding of PHP SPL Data Structures: Tips for Solving Common Problems
Explore PHP SPL Powerful functions of data structure
php editor Banana will help you deeply understand the PHP SPL data structure and master the secrets of solving common problems. SPL (Standard PHP Library), as the standard library of PHP, provides rich data structures and algorithms to help developers process data efficiently. By learning the usage methods and principles of SPL, you can better cope with various challenges encountered in actual development and improve code quality and development efficiency. In this article, we will explore the core concepts and common application scenarios of SPL data structures to help readers better use SPL to solve problems.
Understanding PHP Arrays
SPL's ArrayObject class extends built-in arrays, providing additional functionality such as iterator support and type checking. It can be created via:
$array = new ArrayObject(["foo", "bar", "baz"]);
Mastering the Stack: Last In, First Out (LIFO)
SPL's Stack class implements a last-in-first-out (LIFO) data structure, which can be created in the following ways:
$stack = new SplStack(); $stack->push("a"); $stack->push("b"); $stack->push("c");
Use queue: first in first out (FIFO)
SPL’s Queue class implements a first-in, first-out (FIFO) data structure, which can be created in the following ways:
$queue = new SplQueue(); $queue->enqueue("a"); $queue->enqueue("b"); $queue->enqueue("c");
Traversing linked lists: efficient linear data structure
SPL’s LinkedList class implements a linear data structure that can be created in the following ways:
$list = new SplDoublyLinkedList(); $list->push("a"); $list->push("b"); $list->push("c");
Using hash tables: fast search and insertion
SPL's HashTable class implements a hash table, which uses a hash function to map keys to values, allowing for fast lookups and insertions. Can be created via:
$hashtable = new SplHashTable(); $hashtable["foo"] = "bar"; $hashtable["baz"] = "qux";
Practical combat: Use SPL to solve common problems
Find the unique element in the array:
$array = new ArrayObject(["a", "b", "c", "b"]); $unique = array_unique(iterator_to_array($array));
Reverse linked list:
$list = new SplDoublyLinkedList(); $list->push("a"); $list->push("b"); $list->push("c"); $list->rewind(); while ($list->valid()) { $reversedList->unshift($list->current()); $list->next(); }
Get all keys from hash table:
$hashtable = new SplHashTable(); $hashtable["foo"] = "bar"; $hashtable["baz"] = "qux"; $keys = array_keys(iterator_to_array($hashtable));
in conclusion
PHP SPL data structures provide a powerful set of tools for processing complex data. By understanding and leveraging these constructs, developers can simplify code, improve performance, and solve a variety of programming problems. From managing arrays to working with linked lists and hash tables, SPL provides a comprehensive solution for modern PHP applications.
The above is the detailed content of A Deeper Understanding of PHP SPL Data Structures: Tips for Solving Common Problems. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
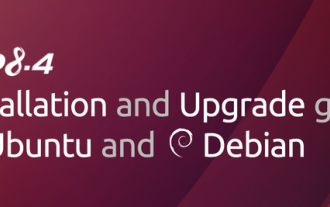
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
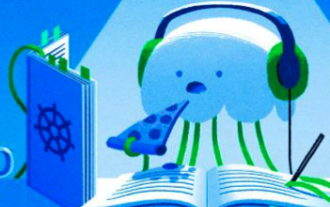
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
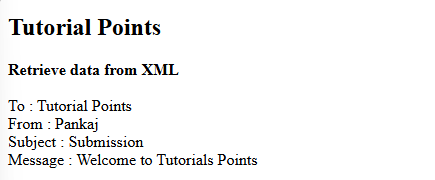
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
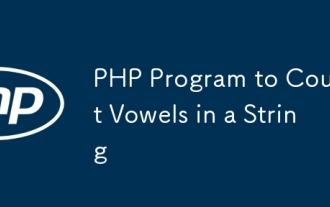
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
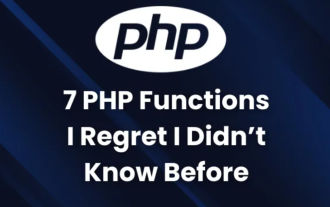
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
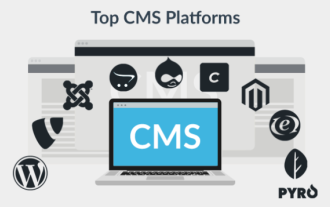
CMS stands for Content Management System. It is a software application or platform that enables users to create, manage, and modify digital content without requiring advanced technical knowledge. CMS allows users to easily create and organize content
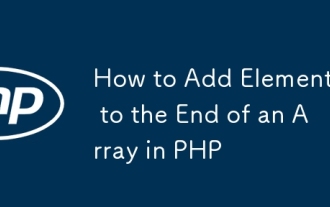
Arrays are linear data structures used to process data in programming. Sometimes when we are processing arrays we need to add new elements to the existing array. In this article, we will discuss several ways to add elements to the end of an array in PHP, with code examples, output, and time and space complexity analysis for each method. Here are the different ways to add elements to an array: Use square brackets [] In PHP, the way to add elements to the end of an array is to use square brackets []. This syntax only works in cases where we want to add only a single element. The following is the syntax: $array[] = value; Example
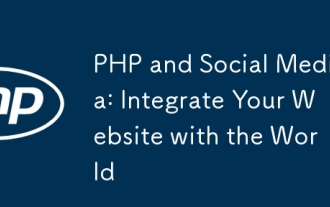
PHP provides tools to allow websites to easily integrate social media functions: 1. Dynamically generate social media sharing buttons for users to share content; 2. Integrate with the OAuth library to achieve seamless social media login; 3. Use the HTTP library to capture social media Data, obtain user profile, posts and other information.
