PHP SPL data structure: improve your code efficiency
php editor Banana has launched a new article "PHP SPL Data Structure: Improve Your Code Efficiency", which deeply discusses the data structures in the PHP standard library to help developers improve code efficiency. SPL (Standard PHP Library) provides a series of powerful data structures and algorithms, allowing you to process data and optimize code logic more efficiently. By learning and applying SPL, you can better understand PHP's data processing capabilities, providing more convenience and possibilities for code development.
PHP The Standard Library (SPL) provides a series of data structure classes that can be used to manage and process data. These structures are optimized to efficiently perform common operations such as insertion, deletion, and lookup. By using SPL data structures, you can improve the efficiency, readability, and maintainability of your code.
Stack
Stacks follow the last-in-first-out (LIFO) principle, which means that the last element added is removed first. The SplStack
class in SPL represents a stack and provides the following methods:
// 创建堆栈 $stack = new SplStack(); // 入栈元素 $stack->push(10); $stack->push(20); $stack->push(30); // 出栈元素并获取 echo $stack->pop() . php_EOL; // 输出 30 echo $stack->pop() . PHP_EOL; // 输出 20 echo $stack->pop() . PHP_EOL; // 输出 10
queue
The queue follows the first-in-first-out (FIFO) principle, which means that the oldest added element is removed first. The SplQueue
class in SPL represents a queue and provides the following methods:
// 创建队列 $queue = new SplQueue(); // 入队元素 $queue->enqueue(10); $queue->enqueue(20); $queue->enqueue(30); // 出队元素并获取 echo $queue->dequeue() . PHP_EOL; // 输出 10 echo $queue->dequeue() . PHP_EOL; // 输出 20 echo $queue->dequeue() . PHP_EOL; // 输出 30
dictionary
Dictionary is a data structure based on key-value pairs. The SplObjectStorage
class in SPL represents a dictionary and provides the following methods:
// 创建字典 $dict = new SplObjectStorage(); // 添加键值对 $obj1 = new stdClass(); $obj2 = new stdClass(); $dict->attach($obj1, 10); $dict->attach($obj2, 20); // 获取键的值 echo $dict[$obj1] . PHP_EOL; // 输出 10 echo $dict[$obj2] . PHP_EOL; // 输出 20
Array object
Array The object provides advanced access and manipulation of ordinary PHP arrays. The SplArray
class in SPL represents an array object and provides the following features:
- Iteration: Use
foreach
to easily iterate over array elements. - Comparison: Use
==
and!=
to compare the contents of arrays. - Clone: Cloning an array object will create a new object instead of referencing the original array.
// 创建数组对象 $arrObj = new SplArray(); $arrObj[] = 10; $arrObj[] = 20; $arrObj[] = 30; // 迭代数组 foreach ($arrObj as $item) { echo $item . PHP_EOL; }
Collection object
Collection The object is an extension of the array object, providing additional features, such as:
- Set operations: Perform set operations such as union, intersection, and complement.
- Filtering: Filter array elements based on conditions.
- Mapping: Map each element in the collection to a new value.
// 创建集合对象 $setObj = new SplObjectStorage(); $setObj->attach(10); $setObj->attach(20); $setObj->attach(30); // 求并集 $s1 = $setObj->count(); $setObj->addAll($arrObj); $s2 = $setObj->count(); echo $s2 - $s1 . PHP_EOL; // 输出 3
in conclusion
PHP SPL data structures provide efficient and easy-to-use mechanisms to manage and process data. By leveraging these structures, you can significantly improve the efficiency, readability, and maintainability of your code. Therefore, it is highly recommended to integrate SPL data structures into your PHP applications.
The above is the detailed content of PHP SPL data structure: improve your code efficiency. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
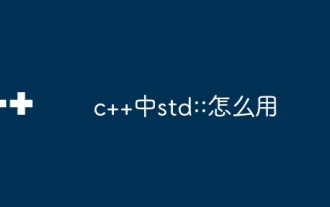
std is the namespace in C++ that contains components of the standard library. In order to use std, use the "using namespace std;" statement. Using symbols directly from the std namespace can simplify your code, but is recommended only when needed to avoid namespace pollution.
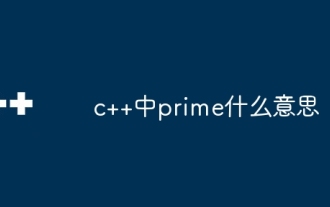
prime is a keyword in C++, indicating the prime number type, which can only be divided by 1 and itself. It is used as a Boolean type to indicate whether the given value is a prime number. If it is a prime number, it is true, otherwise it is false.

The fabs() function is a mathematical function in C++ that calculates the absolute value of a floating point number, removes the negative sign and returns a positive value. It accepts a floating point parameter and returns an absolute value of type double. For example, fabs(-5.5) returns 5.5. This function works with floating point numbers, whose accuracy is affected by the underlying hardware.
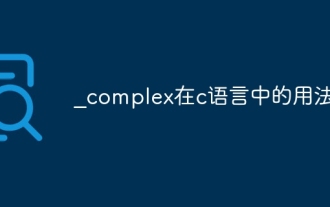
The complex type is used to represent complex numbers in C language, including real and imaginary parts. Its initialization form is complex_number = 3.14 + 2.71i, the real part can be accessed through creal(complex_number), and the imaginary part can be accessed through cimag(complex_number). This type supports common mathematical operations such as addition, subtraction, multiplication, division, and modulo. In addition, a set of functions for working with complex numbers is provided, such as cpow, csqrt, cexp, and csin.
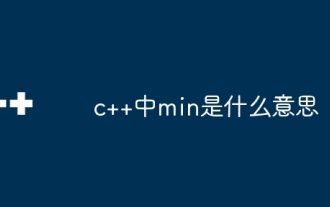
The min function in C++ returns the minimum of multiple values. The syntax is: min(a, b), where a and b are the values to be compared. You can also specify a comparison function to support types that do not support the < operator. C++20 introduced the std::clamp function, which handles the minimum of three or more values.
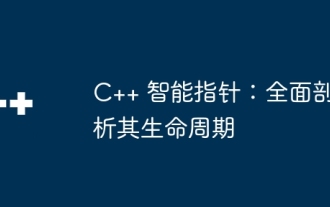
Life cycle of C++ smart pointers: Creation: Smart pointers are created when memory is allocated. Ownership transfer: Transfer ownership through a move operation. Release: Memory is released when a smart pointer goes out of scope or is explicitly released. Object destruction: When the pointed object is destroyed, the smart pointer becomes an invalid pointer.
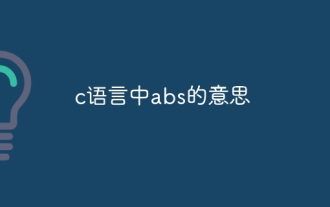
The abs() function in c language is used to calculate the absolute value of an integer or floating point number, i.e. its distance from zero, which is always a non-negative number. It takes a number argument and returns the absolute value of that number.
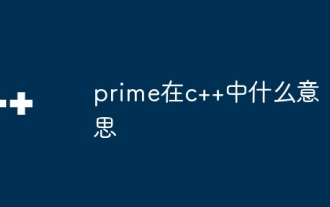
In C++, prime refers to a prime number, a natural number that is greater than 1 and is only divisible by 1 and itself. Prime numbers are widely used in cryptography, mathematical problems and algorithms. Methods for generating prime numbers include Eratostheian sieve, Fermat's Little Theorem, and the Miller-Rabin test. The C++ standard library provides the isPrime function to determine whether it is a prime number, the nextPrime function returns the smallest prime number greater than a given value, and the prevPrime function returns the smallest prime number less than a given value.
