JIT compiler optimization: the secret weapon to speed up Java programs
JIT compiler optimization: the secret weapon to speed up the running of Java programs
Introduction:
In today's computer science field, the Java programming language has become One of the preferred languages for developing all types of applications. However, sometimes we may encounter the problem that Java programs do not run fast enough, especially when processing large amounts of data or requiring high performance. To solve this problem, Java provides a powerful secret weapon, the Just-In-Time Compiler (JIT compiler for short). This article will introduce in detail how the JIT compiler works, and give some specific code examples to show how to optimize the running speed of Java programs through the JIT compiler.
1. What is a JIT compiler?
The JIT compiler is an important component in the Java Virtual Machine (Java Virtual Machine, referred to as JVM). Its main task is to instantly compile the bytecode in the Java program into machine code for direct execution on the computer hardware. Unlike traditional compilers, the JIT compiler does not compile the entire program into machine code in advance, but optimizes and compiles hotspot codes at runtime based on the execution of the program.
2. The working principle of the JIT compiler
The working principle of the JIT compiler can be simply summarized in the following steps:
- Explanation and execution: Java program first It is interpreted and executed, that is, the bytecode is converted into machine code line by line and executed. The purpose of this stage is to identify hot code in the program.
- Hot spot detection: During the interpretation and execution process, the JIT compiler will analyze the running status of the program and record which codes are frequently executed. These frequently executed codes are called hot codes.
- Compilation optimization: Once the hot code is identified, the JIT compiler will optimize and compile this part of the code to generate efficient machine code. These optimizations include removing dead code, inlining function calls, loop unrolling, and constant folding based on runtime data.
- Localized execution: The optimized machine code will be stored in the local cache. The optimized machine code can be directly executed the next time the program runs without the need to interpret and execute it again.
3. Examples of JIT compiler optimization
The following are some common JIT compiler optimization examples:
- Method inlining: JIT compiler Frequently called small methods will be inlined to the calling site to reduce the overhead of method calls.
Sample code:
public class InlineExample { public static int add(int a, int b) { return a + b; } public static void main(String[] args) { int result = 0; for (int i = 0; i < 1000; i++) { result = add(result, i); } System.out.println(result); } }
In the above example, the JIT compiler will inline the add()
method into main()
loop in the method, thereby avoiding the overhead of method calls.
- Loop unrolling: The JIT compiler will expand frequently executed loops into multiple repeated code blocks to reduce the overhead of the loop.
Sample code:
public class LoopUnrollingExample { public static void main(String[] args) { int result = 0; for (int i = 0; i < 10; i++) { result += i; } System.out.println(result); } }
In the above example, the JIT compiler will expand the loop into code in the following form:
public class LoopUnrollingExample { public static void main(String[] args) { int result = 0; result += 0; result += 1; result += 2; result += 3; result += 4; result += 5; result += 6; result += 7; result += 8; result += 9; System.out.println(result); } }
Through loop expansion, the The number of iterations of the loop improves the execution speed of the program.
Conclusion:
The JIT compiler is an important means to optimize the running speed of Java programs. By optimizing compilation of hot code, the JIT compiler can greatly improve the execution speed of Java programs. This article introduces how the JIT compiler works and gives some specific code examples to demonstrate the effect of JIT compiler optimization. I hope that readers can better understand and apply the JIT compiler to optimize their Java programs through the introduction of this article.
The above is the detailed content of JIT compiler optimization: the secret weapon to speed up Java programs. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


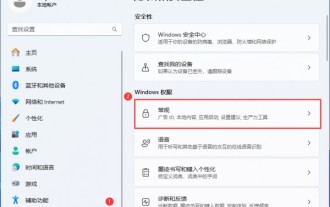
How do we set up and optimize performance after receiving a new computer? Users can directly open Privacy and Security, and then click General (Advertising ID, Local Content, Application Launch, Setting Recommendations, Productivity Tools or directly open Local Group Policy Just use the editor to operate it. Let me introduce to you in detail how to optimize settings and improve performance after receiving a new Win11 computer. How to optimize settings and improve performance after receiving a new Win11 computer. One: 1. Press the [Win+i] key combination to open Settings, then click [Privacy and Security] on the left, and click [General (Advertising ID, Local Content, App Launch, Setting Suggestions, Productivity) under Windows Permissions on the right Tools)】.Method 2
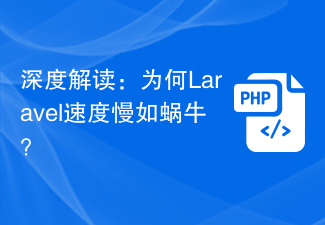
Laravel is a popular PHP development framework, but it is sometimes criticized for being as slow as a snail. What exactly causes Laravel's unsatisfactory speed? This article will provide an in-depth explanation of the reasons why Laravel is as slow as a snail from multiple aspects, and combine it with specific code examples to help readers gain a deeper understanding of this problem. 1. ORM query performance issues In Laravel, ORM (Object Relational Mapping) is a very powerful feature that allows
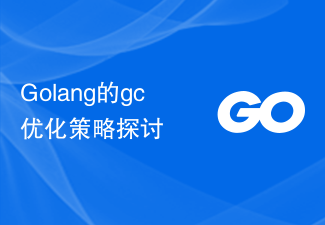
Golang's garbage collection (GC) has always been a hot topic among developers. As a fast programming language, Golang's built-in garbage collector can manage memory very well, but as the size of the program increases, some performance problems sometimes occur. This article will explore Golang’s GC optimization strategies and provide some specific code examples. Garbage collection in Golang Golang's garbage collector is based on concurrent mark-sweep (concurrentmark-s
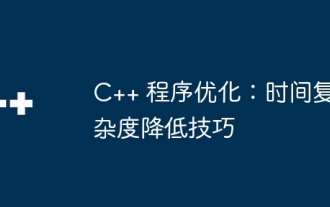
Time complexity measures the execution time of an algorithm relative to the size of the input. Tips for reducing the time complexity of C++ programs include: choosing appropriate containers (such as vector, list) to optimize data storage and management. Utilize efficient algorithms such as quick sort to reduce computation time. Eliminate multiple operations to reduce double counting. Use conditional branches to avoid unnecessary calculations. Optimize linear search by using faster algorithms such as binary search.

Decoding Laravel performance bottlenecks: Optimization techniques fully revealed! Laravel, as a popular PHP framework, provides developers with rich functions and a convenient development experience. However, as the size of the project increases and the number of visits increases, we may face the challenge of performance bottlenecks. This article will delve into Laravel performance optimization techniques to help developers discover and solve potential performance problems. 1. Database query optimization using Eloquent delayed loading When using Eloquent to query the database, avoid
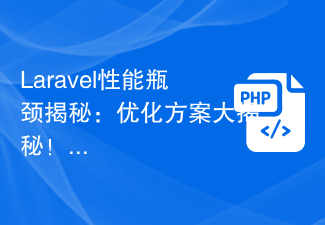
Laravel performance bottleneck revealed: optimization solution revealed! With the development of Internet technology, the performance optimization of websites and applications has become increasingly important. As a popular PHP framework, Laravel may face performance bottlenecks during the development process. This article will explore the performance problems that Laravel applications may encounter, and provide some optimization solutions and specific code examples so that developers can better solve these problems. 1. Database query optimization Database query is one of the common performance bottlenecks in Web applications. exist
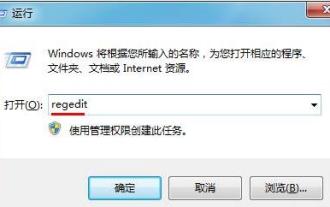
1. Press the key combination (win key + R) on the desktop to open the run window, then enter [regedit] and press Enter to confirm. 2. After opening the Registry Editor, we click to expand [HKEY_CURRENT_USERSoftwareMicrosoftWindowsCurrentVersionExplorer], and then see if there is a Serialize item in the directory. If not, we can right-click Explorer, create a new item, and name it Serialize. 3. Then click Serialize, then right-click the blank space in the right pane, create a new DWORD (32) bit value, and name it Star

Vivox100s parameter configuration revealed: How to optimize processor performance? In today's era of rapid technological development, smartphones have become an indispensable part of our daily lives. As an important part of a smartphone, the performance optimization of the processor is directly related to the user experience of the mobile phone. As a high-profile smartphone, Vivox100s's parameter configuration has attracted much attention, especially the optimization of processor performance has attracted much attention from users. As the "brain" of the mobile phone, the processor directly affects the running speed of the mobile phone.
