Thinking about how to optimize the writing of MyBatis
重新思考MyBatis的写作方式
MyBatis是一个非常流行的Java持久化框架,它能够帮助我们简化数据库操作的编写过程。然而,在日常使用中,我们经常会遇到一些写作方式上的困惑和瓶颈。本文将重新思考MyBatis的写作方式,并提供一些具体的代码示例,以帮助读者更好地理解和应用MyBatis。
- 使用Mapper接口代替SQL语句
在传统的MyBatis写作方式中,我们通常需要在XML文件中编写SQL语句,并通过namespace、select、resultMap等标签将SQL语句与Java代码进行映射。这种方式虽然能够满足基本的数据库操作需求,但在复杂的业务逻辑和大型项目中,XML文件的编写变得冗长而繁琐。
为了简化这一过程,我们可以引入Mapper接口的概念。Mapper接口是一个Java接口,在接口中定义数据库操作的方法。通过接口的方式,我们能够直接在Java代码中调用数据库操作,而无需编写冗长的XML文件。
下面是一个示例,演示了如何定义一个Mapper接口和对应的数据库操作方法:
public interface UserMapper {
// 根据用户名查询用户信息 User getUserByUsername(String username); // 根据用户ID更新用户信息 void updateUserById(User user); // 插入新的用户信息 void insertUser(User user); // 删除用户信息 void deleteUserById(int id);
}
在这个示例中,我们定义了四个数据库操作方法,分别是根据用户名查询用户信息、根据用户ID更新用户信息、插入新的用户信息和删除用户信息。通过使用Mapper接口,我们可以直接在Java代码中调用这些方法,而无需编写繁琐的SQL语句和XML文件。
- 使用注解简化配置
在上述示例中,我们还需要在XML文件中配置Mapper接口的映射关系。为了进一步简化配置过程,我们可以使用注解来代替XML配置。
MyBatis提供了很多注解,可以用于简化配置,例如@Select、@Insert、@Update和@Delete等。通过使用注解,我们可以在Mapper接口的方法上添加对应的注解,来标识数据库操作的类型。
下面是一个示例,演示了如何使用注解来代替XML配置:
public interface UserMapper {
@Select("SELECT * FROM user WHERE username = #{username}") User getUserByUsername(String username); @Update("UPDATE user SET username = #{username}, password = #{password} WHERE id = #{id}") void updateUserById(User user); @Insert("INSERT INTO user(username, password) VALUES(#{username}, #{password})") void insertUser(User user); @Delete("DELETE FROM user WHERE id = #{id}") void deleteUserById(int id);
}
通过使用注解,我们可以直接在Mapper接口的方法上添加对应的SQL语句,从而省去了繁琐的XML配置过程。
- 使用动态SQL
在实际的业务场景中,我们经常会遇到需要根据不同的条件来构建动态SQL语句的情况。MyBatis提供了丰富的动态SQL特性,可以在xml配置文件中编写动态SQL语句。
下面是一个示例,演示了如何使用动态SQL来根据不同条件构建SQL语句:
SELECT * FROM user <where> <if test="username != null"> AND username = #{username} </if> <if test="age != null"> AND age = #{age} </if> </where>
在这个示例中,我们通过
总结
通过重新思考MyBatis的写作方式,我们能够更加灵活和高效地使用这个持久化框架。使用Mapper接口代替XML配置可以简化代码结构和维护成本,使用注解可以进一步简化配置过程,而使用动态SQL可以满足复杂的业务需求。
当然,这些只是一些简单的示例,MyBatis还有很多其他的功能和特性可以探索和应用。希望本文的内容能够帮助读者更深入地理解和应用MyBatis,使数据库操作变得更加简单和高效。
The above is the detailed content of Thinking about how to optimize the writing of MyBatis. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
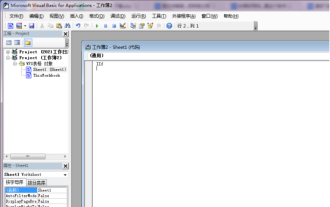
Most users use Excel to process table data. In fact, Excel also has a VBA program. Apart from experts, not many users have used this function. The iif function is often used when writing in VBA. It is actually the same as if The functions of the functions are similar. Let me introduce to you the usage of the iif function. There are iif functions in SQL statements and VBA code in Excel. The iif function is similar to the IF function in the excel worksheet. It performs true and false value judgment and returns different results based on the logically calculated true and false values. IF function usage is (condition, yes, no). IF statement and IIF function in VBA. The former IF statement is a control statement that can execute different statements according to conditions. The latter
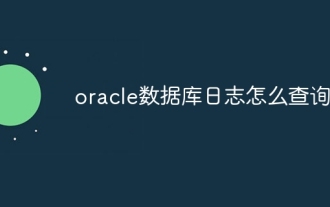
Oracle database log information can be queried by the following methods: Use SQL statements to query from the v$log view; use the LogMiner tool to analyze log files; use the ALTER SYSTEM command to view the status of the current log file; use the TRACE command to view information about specific events; use operations System tools look at the end of the log file.
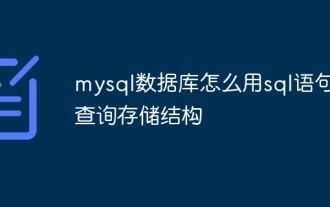
To query the MySQL database storage structure, you can use the following SQL statement: SHOW CREATE TABLE table_name; this statement will return the column definition and table option information of the table, including column name, data type, constraints and general properties of the table, such as storage engine and character set.
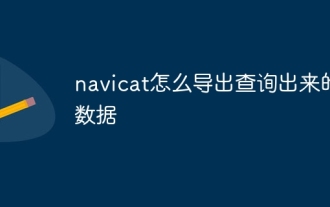
Export query results in Navicat: Execute query. Right-click the query results and select Export Data. Select the export format as needed: CSV: Field separator is comma. Excel: Includes table headers, using Excel format. SQL script: Contains SQL statements used to recreate query results. Select export options (such as encoding, line breaks). Select the export location and file name. Click "Export" to start the export.
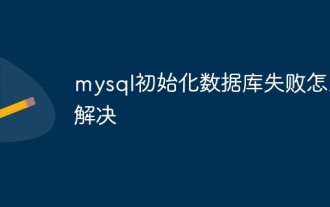
To resolve the MySQL database initialization failure issue, follow these steps: Check permissions and make sure you are using a user with appropriate permissions. If the database already exists, delete it or choose a different name. If the table already exists, delete it or choose a different name. Check the SQL statement for syntax errors. Confirm that the MySQL server is running and connectable. Verify that you are using the correct port number. Check the MySQL log file or Error Code Finder for details of other errors.
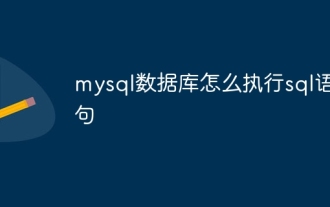
MySQL SQL statements can be executed by: Using the MySQL CLI (Command Line Interface): Log in to the database and enter the SQL statement. Using MySQL Workbench: Start the application, connect to the database, and execute statements. Use a programming language: import the MySQL connection library, create a database connection, and execute statements. Use other tools such as DB Browser for SQLite: download and install the application, open the database file, and execute the statements.
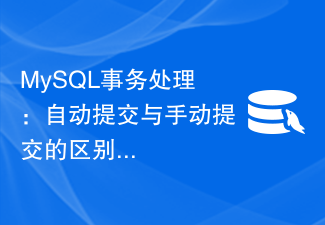
MySQL transaction processing: the difference between automatic submission and manual submission. In the MySQL database, a transaction is a set of SQL statements. Either all executions are successful or all executions fail, ensuring the consistency and integrity of the data. In MySQL, transactions can be divided into automatic submission and manual submission. The difference lies in the timing of transaction submission and the scope of control over the transaction. The following will introduce the difference between automatic submission and manual submission in detail, and give specific code examples to illustrate. 1. Automatically submit in MySQL, if it is not displayed
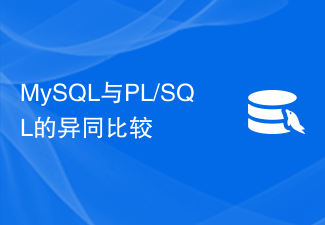
MySQL and PL/SQL are two different database management systems, representing the characteristics of relational databases and procedural languages respectively. This article will compare the similarities and differences between MySQL and PL/SQL, with specific code examples to illustrate. MySQL is a popular relational database management system that uses Structured Query Language (SQL) to manage and operate databases. PL/SQL is a procedural language unique to Oracle database and is used to write database objects such as stored procedures, triggers and functions. same
