JVM memory management key points and precautions
Key points and precautions for mastering JVM memory usage
JVM (Java Virtual Machine) is the environment in which Java applications run, the most important of which is the memory of the JVM manage. Properly managing JVM memory can not only improve application performance, but also avoid problems such as memory leaks and memory overflows. This article will introduce the key points and considerations of JVM memory usage and provide some specific code examples.
- JVM memory partition
JVM memory is mainly divided into the following areas: - Heap: used to store object instances, you can pass the -Xmx and -Xms parameters Adjust the size of the heap.
- Method Area: Storage class information, constant pool, static variables, etc.
- Virtual machine stack (VM Stack): Each thread has a stack used to store method calls and local variables.
- Native Method Stack: used to execute local methods.
- JVM memory parameter configuration
To manage JVM memory reasonably, you need to configure JVM memory parameters reasonably according to the needs of the application. Commonly used parameters are: - -Xmx: Set the maximum value of the heap, which can be adjusted according to the memory requirements of the application.
- -Xms: Set the initial size of the heap, which can be adjusted based on the startup speed of the application.
- -Xmn: Set the size of the young generation, which can affect GC performance by adjusting the size of the young generation.
- -XX:MaxPermSize: Set the maximum value of the method area, which can be adjusted according to the number of classes and static variables of the application.
- Memory Leak and Memory Overflow
A memory leak refers to an application that continues to allocate memory but does not release it, resulting in increasing memory usage. Memory overflow means that the memory required by the application exceeds the memory limit set by the JVM.
Some precautions to avoid memory leaks and memory overflows:
- Release object references in a timely manner: When an object is no longer needed, set its reference to null in a timely manner. In this way, the JVM will recycle the object during the next GC.
- Avoid repeated creation of large objects: For large objects that need to be created frequently, you can use object pools or caches to avoid frequent creation and destruction.
- Pay attention to the use of collection classes: If used improperly, collection classes (such as ArrayList, HashMap, etc.) may cause memory leaks. Pay attention to promptly cleaning up collection objects that are no longer used.
- Use performance analysis tools such as JProfiler: You can view the reference chain of objects through performance analysis tools to help locate the cause of memory leaks or memory overflows.
The following are some specific code examples:
- Examples of timely release of object references:
public void process() { List<String> dataList = new ArrayList<>(); // 处理数据并添加到dataList中 // ... // 处理完毕后将dataList置为null dataList = null; }
- Using object pool Example:
public class ObjectPool { private static final int MAX_SIZE = 100; private static Queue<Object> pool = new LinkedList<>(); public static Object getObject() { if (pool.isEmpty()) { return new Object(); } else { return pool.poll(); } } public static void releaseObject(Object obj) { if (pool.size() < MAX_SIZE) { pool.offer(obj); } } }
- Pay attention to the example of using the collection class:
public void process() { List<Object> dataList = new ArrayList<>(); // 处理数据并添加到dataList中 // ... // 处理完毕后清空dataList dataList.clear(); }
Summary:
Mastering the key points and precautions of JVM memory usage can help We manage memory better, improving application performance and stability. Properly configuring JVM memory parameters, releasing object references in a timely manner, and avoiding memory leaks and memory overflows have become essential skills for an excellent Java developer.
The above is the detailed content of JVM memory management key points and precautions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


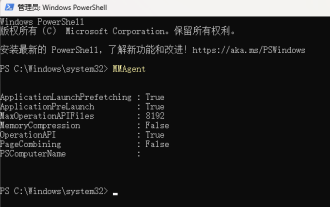
For mechanical hard drives or SATA solid-state drives, you will feel the increase in software running speed. If it is an NVME hard drive, you may not feel it. 1. Import the registry into the desktop and create a new text document, copy and paste the following content, save it as 1.reg, then right-click to merge and restart the computer. WindowsRegistryEditorVersion5.00[HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\Control\SessionManager\MemoryManagement]"DisablePagingExecutive"=d
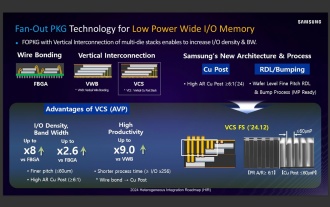
According to news from this website on September 3, Korean media etnews reported yesterday (local time) that Samsung Electronics and SK Hynix’s “HBM-like” stacked structure mobile memory products will be commercialized after 2026. Sources said that the two Korean memory giants regard stacked mobile memory as an important source of future revenue and plan to expand "HBM-like memory" to smartphones, tablets and laptops to provide power for end-side AI. According to previous reports on this site, Samsung Electronics’ product is called LPWide I/O memory, and SK Hynix calls this technology VFO. The two companies have used roughly the same technical route, which is to combine fan-out packaging and vertical channels. Samsung Electronics’ LPWide I/O memory has a bit width of 512
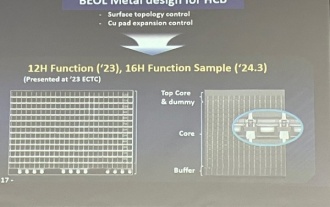
According to the report, Samsung Electronics executive Dae Woo Kim said that at the 2024 Korean Microelectronics and Packaging Society Annual Meeting, Samsung Electronics will complete the verification of the 16-layer hybrid bonding HBM memory technology. It is reported that this technology has passed technical verification. The report also stated that this technical verification will lay the foundation for the development of the memory market in the next few years. DaeWooKim said that Samsung Electronics has successfully manufactured a 16-layer stacked HBM3 memory based on hybrid bonding technology. The memory sample works normally. In the future, the 16-layer stacked hybrid bonding technology will be used for mass production of HBM4 memory. ▲Image source TheElec, same as below. Compared with the existing bonding process, hybrid bonding does not need to add bumps between DRAM memory layers, but directly connects the upper and lower layers copper to copper.
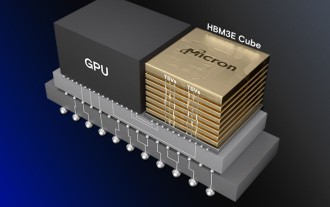
This site reported on March 21 that Micron held a conference call after releasing its quarterly financial report. At the conference, Micron CEO Sanjay Mehrotra said that compared to traditional memory, HBM consumes significantly more wafers. Micron said that when producing the same capacity at the same node, the current most advanced HBM3E memory consumes three times more wafers than standard DDR5, and it is expected that as performance improves and packaging complexity intensifies, in the future HBM4 This ratio will further increase. Referring to previous reports on this site, this high ratio is partly due to HBM’s low yield rate. HBM memory is stacked with multi-layer DRAM memory TSV connections. A problem with one layer means that the entire

According to news from this website on May 6, Lexar launched the Ares Wings of War series DDR57600CL36 overclocking memory. The 16GBx2 set will be available for pre-sale at 0:00 on May 7 with a deposit of 50 yuan, and the price is 1,299 yuan. Lexar Wings of War memory uses Hynix A-die memory chips, supports Intel XMP3.0, and provides the following two overclocking presets: 7600MT/s: CL36-46-46-961.4V8000MT/s: CL38-48-49 -1001.45V In terms of heat dissipation, this memory set is equipped with a 1.8mm thick all-aluminum heat dissipation vest and is equipped with PMIC's exclusive thermal conductive silicone grease pad. The memory uses 8 high-brightness LED beads and supports 13 RGB lighting modes.
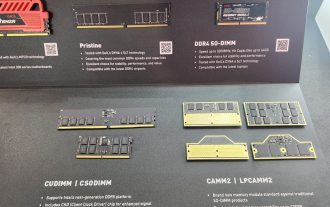
According to news from this site on June 7, GEIL launched its latest DDR5 solution at the 2024 Taipei International Computer Show, and provided SO-DIMM, CUDIMM, CSODIMM, CAMM2 and LPCAMM2 versions to choose from. ▲Picture source: Wccftech As shown in the picture, the CAMM2/LPCAMM2 memory exhibited by Jinbang adopts a very compact design, can provide a maximum capacity of 128GB, and a speed of up to 8533MT/s. Some of these products can even be stable on the AMDAM5 platform Overclocked to 9000MT/s without any auxiliary cooling. According to reports, Jinbang’s 2024 Polaris RGBDDR5 series memory can provide up to 8400
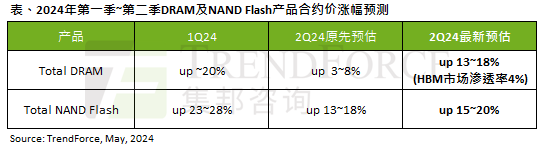
According to a TrendForce survey report, the AI wave has a significant impact on the DRAM memory and NAND flash memory markets. In this site’s news on May 7, TrendForce said in its latest research report today that the agency has increased the contract price increases for two types of storage products this quarter. Specifically, TrendForce originally estimated that the DRAM memory contract price in the second quarter of 2024 will increase by 3~8%, and now estimates it at 13~18%; in terms of NAND flash memory, the original estimate will increase by 13~18%, and the new estimate is 15%. ~20%, only eMMC/UFS has a lower increase of 10%. ▲Image source TrendForce TrendForce stated that the agency originally expected to continue to
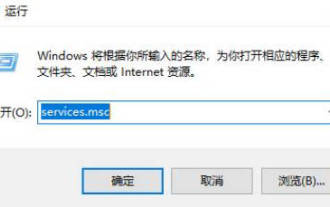
Some users encountered a memory error prompt when using Win10, called "The memory cannot be written". What is going on? Below, the editor will share with you the solution to the Windows 10 system prompt "The memory cannot be written" 1. Press win+r to open the run function on the computer, enter services and msc, and then click OK. 2. Find the Windows Management Instrumentation service in the services window, click "Stop", and then click OK. 3. Still press win+r to open the computer’s run function and enter
