Learn common commands to stop bubbling events!
Learn about common commands to prevent bubbling events!
In web development, event bubbling is one of the common phenomena. When an element triggers an event, such as a click event, if the element's parent element is also bound to the same event, the click event will bubble up from the child element to the parent element. This bubbling behavior sometimes causes unnecessary problems, such as triggering multiple click events or unexpected style changes.
In order to solve these problems, we can use some common instructions to prevent events from bubbling. Some common methods are described below.
- stopPropagation()
stopPropagation() is a built-in method in JavaScript that can be used to stop the bubbling process of events. When the event is triggered, call this method and the event will no longer be propagated to the parent element. We can use the following code in the event handling function to prevent bubbling:
function handleClick(event) { event.stopPropagation(); // 其他处理代码 }
- stopImmediatePropagation()
stopImmediatePropagation() is a further extension of stopPropagation(), in addition to preventing events from bubbling In addition to the bubble, it can also prevent the execution of subsequent event processing functions. When the event is triggered and this method is called, the bubbling process of the event will stop immediately, and other bound event handling functions will not be executed. The usage is as follows:
function handleClick(event) { event.stopImmediatePropagation(); // 其他处理代码 }
- return false
In some special cases, we can also use return false to prevent events from bubbling. For example, use return false in the event handling attribute of the HTML element, such as:
<button onclick="return false;"></button>
This method is relatively simple and direct, but it only applies to the event handling attribute of the HTML element and cannot be used in JavaScript code.
It should be noted that although the above methods can prevent the event from bubbling, they cannot prevent the default behavior of the event, such as clicking a link to jump to the page. If you need to prevent event bubbling and default behavior at the same time, you can use the preventDefault() method:
function handleClick(event) { event.stopPropagation(); event.preventDefault(); // 其他处理代码 }
In actual development, we can choose an appropriate method to prevent event bubbling according to the specific situation. When we need to bind the same event to multiple parent elements and only want the event to be triggered on a specific element, we can use stopPropagation(). If you not only need to prevent bubbling, but also prevent the execution of subsequent event handling functions, you can use stopImmediatePropagation(). Return false is suitable for simple HTML element event handling attributes.
To summarize, understanding the common instructions to prevent bubbling events can help us handle events better. Choosing the appropriate method according to the specific situation can avoid unnecessary problems and improve the user experience of web applications. At the same time, it is necessary to pay attention to the scope of use and precautions of the method to avoid causing other unexpected situations.
The above is the detailed content of Learn common commands to stop bubbling events!. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
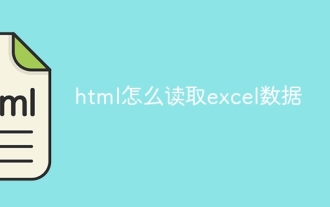
How to read excel data in html: 1. Use JavaScript library to read Excel data; 2. Use server-side programming language to read Excel data.
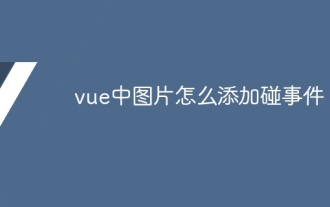
How to add click event to image in Vue? Import the Vue instance. Create a Vue instance. Add images to HTML templates. Add click events using the v-on:click directive. Define the handleClick method in the Vue instance.
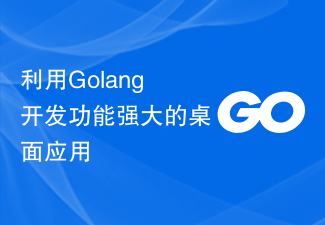
Use Golang to develop powerful desktop applications. With the continuous development of the Internet, people have become inseparable from various types of desktop applications. For developers, it is crucial to use efficient programming languages to develop powerful desktop applications. This article will introduce how to use Golang (Go language) to develop powerful desktop applications and provide some specific code examples. Golang is an open source programming language developed by Google. It has the characteristics of simplicity, efficiency, strong concurrency, etc., and is very suitable for
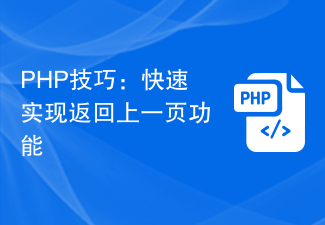
PHP Tips: Quickly implement the function of returning to the previous page. In web development, we often encounter the need to implement the function of returning to the previous page. Such operations can improve the user experience and make it easier for users to navigate between web pages. In PHP, we can achieve this function through some simple code. This article will introduce how to quickly implement the function of returning to the previous page and provide specific PHP code examples. In PHP, we can use $_SERVER['HTTP_REFERER'] to get the URL of the previous page
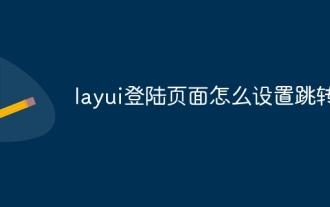
Layui login page jump setting steps: Add jump code: Add judgment in the login form submit button click event, and jump to the specified page through window.location.href after successful login. Modify the form configuration: add a hidden input field to the form element of lay-filter="login", with the name "redirect" and the value being the target page address.
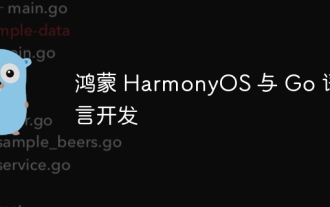
Introduction to HarmonyOS and Go language development HarmonyOS is a distributed operating system developed by Huawei, and Go is a modern programming language. The combination of the two provides a powerful solution for developing distributed applications. This article will introduce how to use Go language for development in HarmonyOS, and deepen understanding through practical cases. Installation and Setup To use Go language to develop HarmonyOS applications, you need to install GoSDK and HarmonyOSSDK first. The specific steps are as follows: #Install GoSDKgogetgithub.com/golang/go#Set PATH
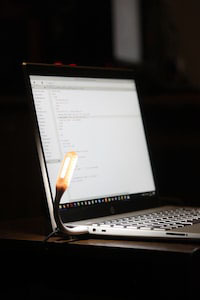
We will also cover another way to execute a PHP function through the onclick() event using the Jquery library. This method calls a javascript function, which will output the content of the php function in the web page. We will also demonstrate another way to execute a PHP function using the onclick() event, calling the PHP function using pure JavaScript. This article will introduce a way to execute a PHP function, use the GET method to send the data in the URL, and use the isset() function to check the GET data. This method calls a PHP function if the data is set and the function is executed. Using jQuery to execute a PHP function through the onclick() event we can use
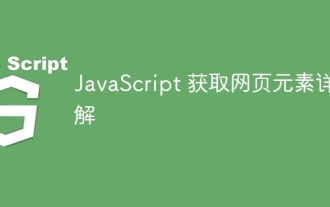
Answer: JavaScript provides a variety of methods for obtaining web page elements, including using ids, tag names, class names, and CSS selectors. Detailed description: getElementById(id): Get elements based on unique id. getElementsByTagName(tag): Gets the element group with the specified tag name. getElementsByClassName(class): Gets the element group with the specified class name. querySelector(selector): Use CSS selector to get the first matching element. querySelectorAll(selector): Get all matches using CSS selector
