


Python inheritance and polymorphism: starting a fantastic journey of object-oriented programming
Inheritance: The Art of Code Reuse
Inheritance is an OOP mechanism that allows you to create new classes, called subclasses or derived classes, from existing classes. Subclasses inherit the properties and methods of the parent class and can extend or modify them. This way, you can create code reuse and layers of specialization.
Demo code:
class Animal: def __init__(self, name): self.name = name class Dog(Animal): def bark(self): print(f"{self.name} barks!")
In the above example, the Dog
class inherits the __init__
method of the Animal
class to initialize the name attribute. Additionally, it defines a bark
method, which is behavior unique to subclasses.
Polymorphism: Flexibility in Code
Polymorphism is an OOP concept that allows the behavior of an object to vary depending on its type. This means you can write code once and it will work with different types of objects, depending on the runtime object type.
Demo code:
def make_animal_sound(animal): animal.make_sound()
In this example, the make_animal_sound
function works with any object that implements the make_sound
method. If animal
is an instance of Dog
, it prints bark, and if it is an instance of Cat
, it prints meow.
Advantages of polymorphism
- Improve code flexibility: Polymorphism allows you to handle different types of objects without modifying your code.
- Simplify code maintenance: By separating behavior from object type, you can easily update or extend behavior in subclasses without affecting the parent class.
- Promote code reuse: You can define a public interface for a group of related objects, and then create subclasses to customize the behavior.
The combination of inheritance and polymorphism
Inheritance and polymorphism complement each other. Inheritance allows you to create class hierarchies, while polymorphism allows you to write dynamic code that works with different types of objects. By combining these concepts, you can create highly reusable, flexible, and maintainable code.
Advantages of object-oriented programming
Following OOP principles brings the following benefits:
- Encapsulation: Hide internal implementation details, thereby improving code security.
- Abstraction: Create abstract classes and interfaces to define the public interface of an object.
- Reusable: Reuse code through inheritance and polymorphism, thereby improving development efficiency.
- Maintainability: Make the code easier to maintain by using clearly defined classes and interfaces.
in conclusion
python Inheritance and polymorphism are powerful OOP tools that can significantly enhance the flexibility and reusability of your code. By understanding and becoming proficient in using these concepts, you can improve your programming skills and create more elegant and robust applications.
The above is the detailed content of Python inheritance and polymorphism: starting a fantastic journey of object-oriented programming. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


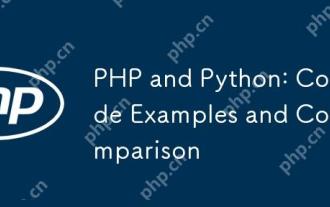
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
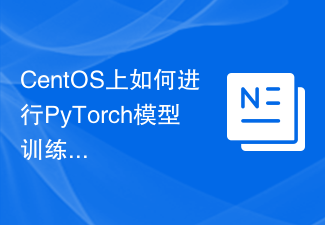
Efficient training of PyTorch models on CentOS systems requires steps, and this article will provide detailed guides. 1. Environment preparation: Python and dependency installation: CentOS system usually preinstalls Python, but the version may be older. It is recommended to use yum or dnf to install Python 3 and upgrade pip: sudoyumupdatepython3 (or sudodnfupdatepython3), pip3install--upgradepip. CUDA and cuDNN (GPU acceleration): If you use NVIDIAGPU, you need to install CUDATool
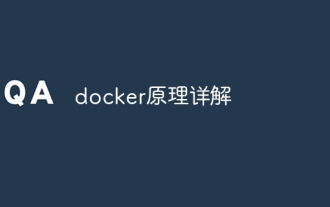
Docker uses Linux kernel features to provide an efficient and isolated application running environment. Its working principle is as follows: 1. The mirror is used as a read-only template, which contains everything you need to run the application; 2. The Union File System (UnionFS) stacks multiple file systems, only storing the differences, saving space and speeding up; 3. The daemon manages the mirrors and containers, and the client uses them for interaction; 4. Namespaces and cgroups implement container isolation and resource limitations; 5. Multiple network modes support container interconnection. Only by understanding these core concepts can you better utilize Docker.
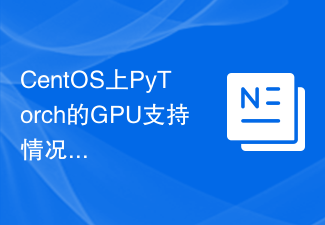
Enable PyTorch GPU acceleration on CentOS system requires the installation of CUDA, cuDNN and GPU versions of PyTorch. The following steps will guide you through the process: CUDA and cuDNN installation determine CUDA version compatibility: Use the nvidia-smi command to view the CUDA version supported by your NVIDIA graphics card. For example, your MX450 graphics card may support CUDA11.1 or higher. Download and install CUDAToolkit: Visit the official website of NVIDIACUDAToolkit and download and install the corresponding version according to the highest CUDA version supported by your graphics card. Install cuDNN library:
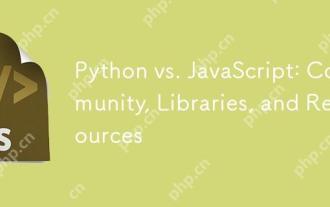
Python and JavaScript have their own advantages and disadvantages in terms of community, libraries and resources. 1) The Python community is friendly and suitable for beginners, but the front-end development resources are not as rich as JavaScript. 2) Python is powerful in data science and machine learning libraries, while JavaScript is better in front-end development libraries and frameworks. 3) Both have rich learning resources, but Python is suitable for starting with official documents, while JavaScript is better with MDNWebDocs. The choice should be based on project needs and personal interests.

When selecting a PyTorch version under CentOS, the following key factors need to be considered: 1. CUDA version compatibility GPU support: If you have NVIDIA GPU and want to utilize GPU acceleration, you need to choose PyTorch that supports the corresponding CUDA version. You can view the CUDA version supported by running the nvidia-smi command. CPU version: If you don't have a GPU or don't want to use a GPU, you can choose a CPU version of PyTorch. 2. Python version PyTorch

MinIO Object Storage: High-performance deployment under CentOS system MinIO is a high-performance, distributed object storage system developed based on the Go language, compatible with AmazonS3. It supports a variety of client languages, including Java, Python, JavaScript, and Go. This article will briefly introduce the installation and compatibility of MinIO on CentOS systems. CentOS version compatibility MinIO has been verified on multiple CentOS versions, including but not limited to: CentOS7.9: Provides a complete installation guide covering cluster configuration, environment preparation, configuration file settings, disk partitioning, and MinI
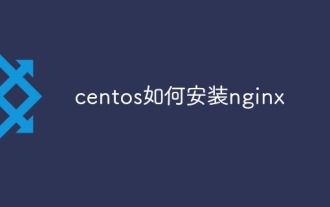
CentOS Installing Nginx requires following the following steps: Installing dependencies such as development tools, pcre-devel, and openssl-devel. Download the Nginx source code package, unzip it and compile and install it, and specify the installation path as /usr/local/nginx. Create Nginx users and user groups and set permissions. Modify the configuration file nginx.conf, and configure the listening port and domain name/IP address. Start the Nginx service. Common errors need to be paid attention to, such as dependency issues, port conflicts, and configuration file errors. Performance optimization needs to be adjusted according to the specific situation, such as turning on cache and adjusting the number of worker processes.
