How to use try and catch in C
How to use try and catch in C requires specific code examples
In C language, there is no built-in try and catch mechanism for exception handling. However, the functionality of try and catch can be simulated by using the setjmp and longjmp functions. Below I will introduce in detail how to use these two functions for exception handling and give corresponding code examples.
First of all, we need to understand the principles of setjmp and longjmp functions. When the setjmp function is called, it saves the state of the current program and returns a mark, which can be used to identify the point where the exception occurs in subsequent code. The longjmp function can jump to the previously set exception handling point according to the mark, thereby realizing exception capture and processing.
The following is a simple example that demonstrates how to use the setjmp and longjmp functions to implement the try and catch functions:
#include <stdio.h> #include <setjmp.h> jmp_buf jump_buffer; void divide(int num, int denom) { if (denom == 0) { longjmp(jump_buffer, 1); // 发生异常,跳转到异常处理点 } printf("Result: %d ", num / denom); } int main() { if (setjmp(jump_buffer) == 0) { // 设置异常处理点 divide(10, 0); } else { printf("Error: Division by zero. "); } return 0; }
In the above code, we define a divide function for Perform division operations. If the divisor is 0, a divide-by-zero exception will occur. We use the setjmp function to set an exception handling point in the main function, and then call the divide function. If an exception occurs, calling the longjmp function will jump to setjmp and return a value of 1 to indicate that an exception occurred. When handling exceptions, we output relevant error information.
Through the above code, we have implemented a simple exception handling mechanism. Of course, in practice, there may be more complex abnormal situations that require more comprehensive processing. This requires expansion on the basis of setjmp and longjmp, including the definition of exception types, the registration of exception handling functions, etc.
To summarize, although the C language itself does not have a built-in try and catch mechanism, exception handling can be simulated through the setjmp and longjmp functions. By using these two functions properly, we can write more robust and reliable programs in C language.
The above is an introduction and code examples on how to use try and catch in C. Hope this helps!
The above is the detailed content of How to use try and catch in C. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


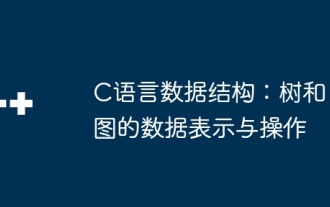
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
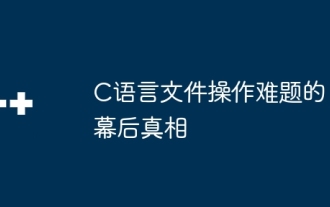
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
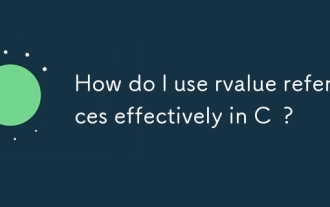
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
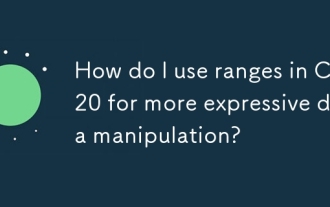
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
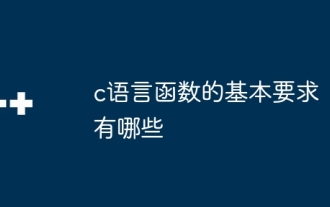
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
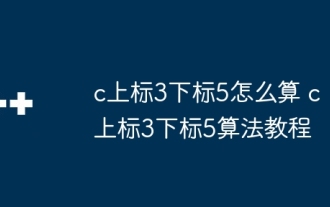
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
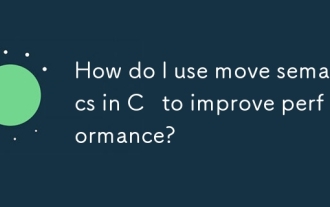
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
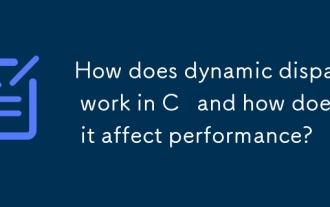
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
