The Alchemy of Python Syntax: Turning Code into Magic
#python is a simple and powerful programming language, known for its concise syntax and rich standard library. By mastering every aspect of Python syntax, you can take advantage of the power of the language and take your code to the next level.
type of data:
Python has a variety of built-in data types, including numbers (int, float), strings (str), lists (list), tuples (tuple), and dictionaries (dict). Understanding these data types and how to use them is critical to working with data effectively. For example:
# 创建一个整数 my_number = 10 # 创建一个字符串 my_string = "Hello world" # 创建一个列表 my_list = [1, 2, 3]
Control flow:
Python uses conditional statements (if, elif, else) and loops (for, while) to control program flow. These statements allow you to execute different blocks of code or to execute blocks of code repeatedly based on conditions. For example:
# 使用 if 语句检查条件 if my_number > 5: print("My number is greater than 5") # 使用 for 循环遍历列表 for item in my_list: print(item)
function:
Functions are powerful tools for organizing code into modular units. They allow you to reuse code and pass and return data as needed. In Python, functions are defined using the def keyword. For example:
# 定义一个函数来计算平方 def square(number): return number * number # 调用函数 result = square(5) print(result)# 输出:25
Object-Oriented Programming:
Python is an object-orientedprogramming language that allows you to create objects and classes to represent real-world entities and behaviors. Objects have state (properties) and behavior (methods). For example:
# 创建一个 Person 类 class Person: def __init__(self, name, age): self.name = name self.age = age def get_name(self): return self.name def get_age(self): return self.age # 创建一个 Person 对象 person = Person("John", 30) # 访问对象属性和方法 print(person.get_name())# 输出:John print(person.get_age())# 输出:30
More advanced features:
In addition to the above basics, Python also provides a series of advanced features that can greatly improve the efficiency and readability of your code. These features include:
- List comprehensions and generator expressions
- Exception handling
- Class inheritance
- Decorator
in conclusion:
Mastering all aspects of Python syntax is key to becoming a skilled Python developer. By learning about data types, control flow, functions, object-oriented programming, and advanced features, you can take your code to the next level and unlock the full potential of Python.
The above is the detailed content of The Alchemy of Python Syntax: Turning Code into Magic. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
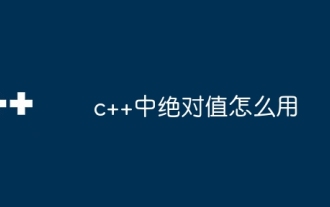
There are two ways to obtain absolute values in C++: 1. Use the built-in function abs() to obtain the absolute value of an integer or floating point type; 2. Use the generic function std::abs() to obtain various supported absolute values. Operates on absolute values of data types.
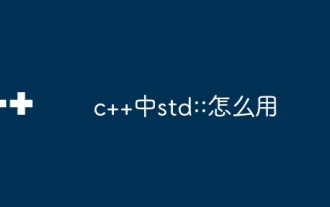
std is the namespace in C++ that contains components of the standard library. In order to use std, use the "using namespace std;" statement. Using symbols directly from the std namespace can simplify your code, but is recommended only when needed to avoid namespace pollution.
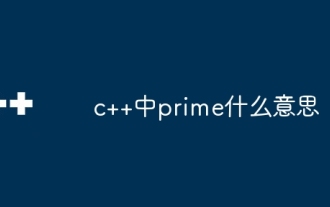
prime is a keyword in C++, indicating the prime number type, which can only be divided by 1 and itself. It is used as a Boolean type to indicate whether the given value is a prime number. If it is a prime number, it is true, otherwise it is false.
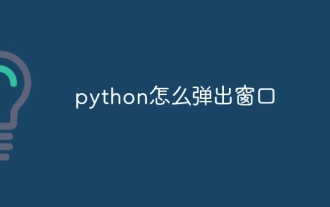
There are two ways to create popups in Python: Tkinter: Use the Tkinter library to create Tk or TopLevel widgets. Pyglet: Use the Pyglet library to create Window windows.
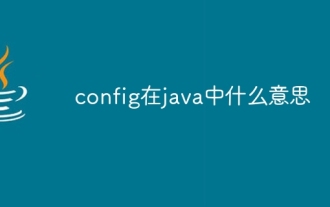
Config represents configuration information in Java and is used to adjust application behavior. It is usually stored in external files or databases and can be managed through Java Properties, PropertyResourceBundle, Java Configuration Framework or third-party libraries. Its benefits include decoupling and flexibility. , environmental awareness, manageability, scalability.

The fabs() function is a mathematical function in C++ that calculates the absolute value of a floating point number, removes the negative sign and returns a positive value. It accepts a floating point parameter and returns an absolute value of type double. For example, fabs(-5.5) returns 5.5. This function works with floating point numbers, whose accuracy is affected by the underlying hardware.
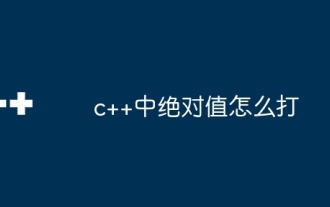
There are three ways to find the absolute value in C++: Using the abs() function, you can calculate the absolute value of any type of number. Using the std::abs() function, you can calculate the absolute value of integers, floating point numbers, and complex numbers. Manual calculation of absolute values, suitable for simple integers.
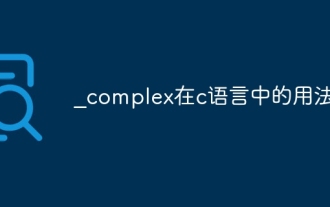
The complex type is used to represent complex numbers in C language, including real and imaginary parts. Its initialization form is complex_number = 3.14 + 2.71i, the real part can be accessed through creal(complex_number), and the imaginary part can be accessed through cimag(complex_number). This type supports common mathematical operations such as addition, subtraction, multiplication, division, and modulo. In addition, a set of functions for working with complex numbers is provided, such as cpow, csqrt, cexp, and csin.
