


Golang data conversion method: Flexible application of type conversion to achieve complex data processing
Golang data conversion method: Flexible application of type conversion to achieve complex data processing
In Golang, data conversion is a very common operation, especially when we need to process complex data data structure. By flexibly applying type conversion, we can process, parse and convert data to achieve the data format and structure we want. In this article, we will explore how to use the type conversion method in Golang to process complex data, and provide specific code examples for your reference.
The importance of data conversion
In software development, we often need to obtain data from different data sources, which may exist in different formats and structures. And we often need to convert these data into the format we need and perform corresponding processing and analysis. The process of data conversion is very critical, and it directly affects our understanding and utilization of data.
As a powerful programming language, Golang provides a wealth of data processing and type conversion methods, which can help us effectively handle various data conversion needs. By flexibly applying these methods, we can process complex data and provide our applications with powerful data processing capabilities.
Basic type conversion
In Golang, conversion between basic data types is very simple and straightforward. For example, to convert an integer into a string, you can directly use strconv.Itoa()
function:
package main import ( "fmt" "strconv" ) func main() { num := 10 str := strconv.Itoa(num) fmt.Println(str) }
Similarly, to convert a string into an integer is also easy, you can use strconv.Atoi()
Function:
package main import ( "fmt" "strconv" ) func main() { str := "20" num, _ := strconv.Atoi(str) fmt.Println(num) }
Through these simple basic type conversions, we can easily handle different types of data.
Custom type conversion
In actual development, we often encounter situations where we need to customize data types. At this time, we also need to implement custom type conversion. For example, we define a custom structure type Person
:
package main import "fmt" type Person struct { Name string Age int } func main() { p := Person{Name: "Alice", Age: 25} fmt.Println(p) }
If we want to convert the Person
type into a string type, we can String()
Method:
func (p Person) String() string { return fmt.Sprintf("Name: %s, Age: %d", p.Name, p.Age) }
So we can convert the Person
type into a string by calling the String()
method.
Conversion of complex data structures
When we need to deal with complex data structures, type conversion will be more complex and important. For example, we have data in JSON format, and we need to parse it into a structure for processing. In Golang, you can use the encoding/json
package to convert between JSON data and structures:
package main import ( "encoding/json" "fmt" ) type User struct { Name string `json:"name"` Age int `json:"age"` } func main() { data := []byte(`{"name": "Bob", "age": 30}`) var user User err := json.Unmarshal(data, &user) if err != nil { fmt.Println("解析错误:", err) } fmt.Println(user) }
This code parses JSON data into User
Structures realize conversion between complex data structures.
Conclusion
Through the explanation and sample code of this article, we can see how to flexibly apply type conversion in Golang to process complex data. Type conversion is a very common and important operation in Golang programming. Mastering the method of type conversion allows us to handle various data structures more flexibly and improve the readability and maintainability of the code.
I hope the content of this article can help everyone better understand the data conversion method in Golang and play a role in practical applications. Let us explore more tips and methods about Golang data processing and improve our programming abilities!
The above is the detailed content of Golang data conversion method: Flexible application of type conversion to achieve complex data processing. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


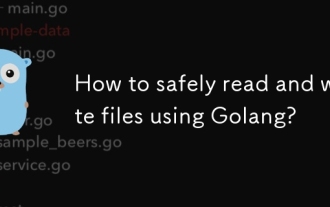
Reading and writing files safely in Go is crucial. Guidelines include: Checking file permissions Closing files using defer Validating file paths Using context timeouts Following these guidelines ensures the security of your data and the robustness of your application.
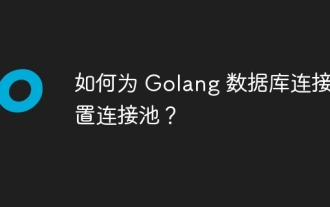
How to configure connection pooling for Go database connections? Use the DB type in the database/sql package to create a database connection; set MaxOpenConns to control the maximum number of concurrent connections; set MaxIdleConns to set the maximum number of idle connections; set ConnMaxLifetime to control the maximum life cycle of the connection.
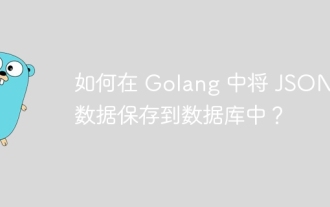
JSON data can be saved into a MySQL database by using the gjson library or the json.Unmarshal function. The gjson library provides convenience methods to parse JSON fields, and the json.Unmarshal function requires a target type pointer to unmarshal JSON data. Both methods require preparing SQL statements and performing insert operations to persist the data into the database.
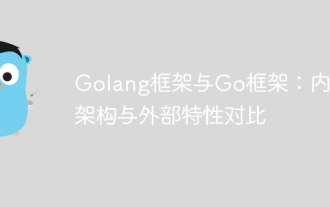
The difference between the GoLang framework and the Go framework is reflected in the internal architecture and external features. The GoLang framework is based on the Go standard library and extends its functionality, while the Go framework consists of independent libraries to achieve specific purposes. The GoLang framework is more flexible and the Go framework is easier to use. The GoLang framework has a slight advantage in performance, and the Go framework is more scalable. Case: gin-gonic (Go framework) is used to build REST API, while Echo (GoLang framework) is used to build web applications.
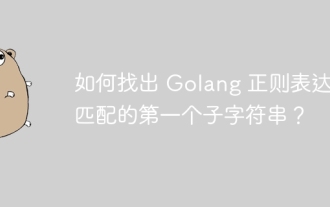
The FindStringSubmatch function finds the first substring matched by a regular expression: the function returns a slice containing the matching substring, with the first element being the entire matched string and subsequent elements being individual substrings. Code example: regexp.FindStringSubmatch(text,pattern) returns a slice of matching substrings. Practical case: It can be used to match the domain name in the email address, for example: email:="user@example.com", pattern:=@([^\s]+)$ to get the domain name match[1].
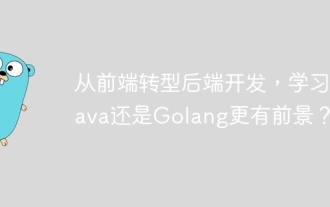
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
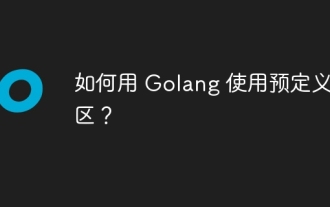
Using predefined time zones in Go includes the following steps: Import the "time" package. Load a specific time zone through the LoadLocation function. Use the loaded time zone in operations such as creating Time objects, parsing time strings, and performing date and time conversions. Compare dates using different time zones to illustrate the application of the predefined time zone feature.
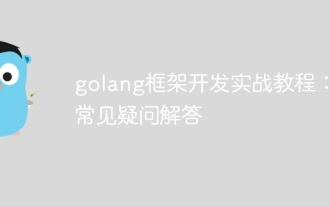
Go framework development FAQ: Framework selection: Depends on application requirements and developer preferences, such as Gin (API), Echo (extensible), Beego (ORM), Iris (performance). Installation and use: Use the gomod command to install, import the framework and use it. Database interaction: Use ORM libraries, such as gorm, to establish database connections and operations. Authentication and authorization: Use session management and authentication middleware such as gin-contrib/sessions. Practical case: Use the Gin framework to build a simple blog API that provides POST, GET and other functions.
