Uncovering the secrets of PHP design patterns
PHP design pattern, as an important concept in development, is crucial to improving code quality and maintainability. PHP editor Xinyi will reveal the secrets of PHP design patterns, lead readers to have an in-depth understanding of the principles and applications of various design patterns, unveil the mystery of design patterns for developers, and help them flexibly use design patterns in projects and improve code Quality and efficiency.
PHP Design patterns are predefined code templates designed to solve common software development problems. They provide proven solutions that improve code reusability, maintainability, and scalability.
2. Types of PHP design patterns
There are many different design patterns inphp, and each pattern has its specific purpose. The most common patterns include:
- Single case mode: Ensure that there is only one instance of a class.
- Factory pattern: Create objects of different types based on the data passed to it.
- Strategy Mode: Allows a program to change its behavior at runtime.
- Observer pattern: Allows objects to subscribe to events and get notified when events occur.
3. Singleton pattern example
class SingleInstance { private static $instance; private function __construct() {} public static function getInstance() { if (!isset(self::$instance)) { self::$instance = new SingleInstance(); } return self::$instance; } }
By using the getInstance()
method, you can ensure that there is only one SingleInstance
object in your program.
4. Factory pattern example
class ShapeFactory { public static function createShape($type) { switch ($type) { case "square": return new Square(); case "circle": return new Circle(); default: throw new Exception("Unsupported shape type"); } } }
This factory pattern allows you to create different types of shape objects based on an input parameter.
5. Strategy pattern example
class SortAlGorithm { public function sort($array) { // Implement the specific sorting algorithm here } } class BubbleSortAlgorithm extends SortAlgorithm {} class MergeSortAlgorithm extends SortAlgorithm {} class Sorter { private $algorithm; public function __construct(SortAlgorithm $algorithm) { $this->algorithm = $algorithm; } public function sort($array) { $this->algorithm->sort($array); } }
Strategy mode allows you to change the sorting algorithm at runtime.
6. Observer pattern example
class Subject { private $observers = []; public function addObserver(Observer $observer) { $this->observers[] = $observer; } public function notifyObservers() { foreach ($this->observers as $observer) { $observer->update(); } } } class Observer { public function update() { // Handle the event notification here } }
The observer pattern allows objects to subscribe to topics and receive event notifications.
7. Advantages of PHP design patterns
PHP design patterns provide many benefits, including:
- Reusability: Patterns provide predefined code templates that can be easily reused.
- Maintainability: Patterns make code easier to read, understand, and modify.
- Scalability: Patterns allow code to be easily expanded as requirements change.
8. Conclusion
PHP design patterns are valuable tools for improving the quality of your PHP applications. By understanding and applying these patterns, developers can create more reusable, maintainable, and scalable code.
The above is the detailed content of Uncovering the secrets of PHP design patterns. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
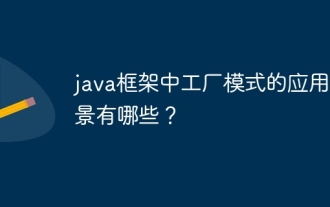
The factory pattern is used to decouple the creation process of objects and encapsulate them in factory classes to decouple them from concrete classes. In the Java framework, the factory pattern is used to: Create complex objects (such as beans in Spring) Provide object isolation, enhance testability and maintainability Support extensions, increase support for new object types by adding new factory classes
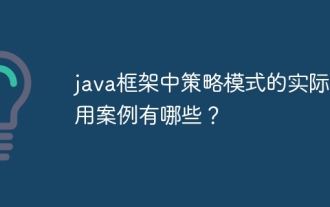
The strategy pattern in the Java framework is used to dynamically change class behavior. Specific applications include: Spring framework: data validation and cache management JakartaEE framework: transaction management and dependency injection JSF framework: converters and validators, response life cycle management
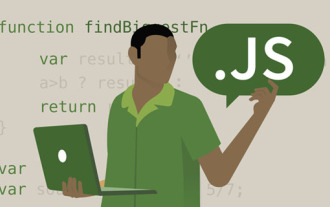
The JS singleton pattern is a commonly used design pattern that ensures that a class has only one instance. This mode is mainly used to manage global variables to avoid naming conflicts and repeated loading. It can also reduce memory usage and improve code maintainability and scalability.
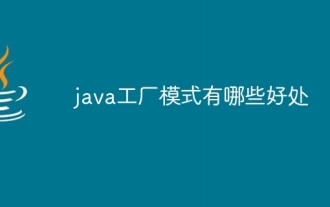
The benefits of the Java factory pattern: 1. Reduce system coupling; 2. Improve code reusability; 3. Hide the object creation process; 4. Simplify the object creation process; 5. Support dependency injection; 6. Provide better performance; 7. Enhance testability; 8. Support internationalization; 9. Promote the open and closed principle; 10. Provide better scalability. Detailed introduction: 1. Reduce the coupling of the system. The factory pattern reduces the coupling of the system by centralizing the object creation process into a factory class; 2. Improve the reusability of code, etc.
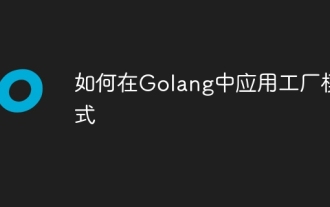
Factory Pattern In Go, the factory pattern allows the creation of objects without specifying a concrete class: define an interface (such as Shape) that represents the object. Create concrete types (such as Circle and Rectangle) that implement this interface. Create a factory class to create objects of a given type (such as ShapeFactory). Use factory classes to create objects in client code. This design pattern increases the flexibility of the code without directly coupling to concrete types.
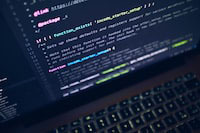
In modern software development, creating scalable, maintainable applications is crucial. PHP design patterns provide a set of proven best practices that help developers achieve code reuse and increase scalability, thereby reducing complexity and development time. What are PHP design patterns? Design patterns are reusable programming solutions to common software design problems. They provide a unified and common way to organize and structure code, thereby promoting code reuse, extensibility, and maintainability. SOLID Principles The PHP design pattern follows the SOLID principles: S (Single Responsibility): Each class or function should be responsible for a single responsibility. O (Open-Closed): The class should be open for extension, but closed for modification. L (Liskov replacement): subclasses should
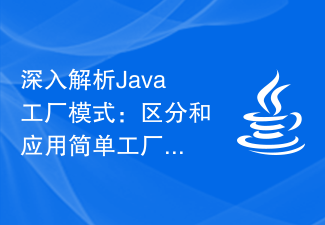
Detailed explanation of Java Factory Pattern: Understand the differences and application scenarios of simple factories, factory methods and abstract factories Introduction In the software development process, when faced with complex object creation and initialization processes, we often need to use the factory pattern to solve this problem. As a commonly used object-oriented programming language, Java provides a variety of factory pattern implementations. This article will introduce in detail the three common implementation methods of the Java factory pattern: simple factory, factory method and abstract factory, and conduct an in-depth analysis of their differences and application scenarios. one,
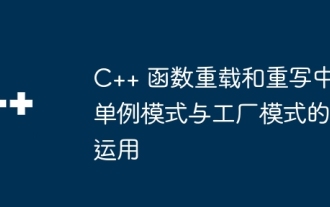
Singleton pattern: Provide singleton instances with different parameters through function overloading. Factory pattern: Create different types of objects through function rewriting to decouple the creation process from specific product classes.
