


MyBatis cache strategy analysis: best practices for first-level cache and second-level cache
MyBatis cache strategy analysis: best practices for first-level cache and second-level cache
When using MyBatis for development, we often need to consider the choice of cache strategy. The cache in MyBatis is mainly divided into two types: first-level cache and second-level cache. The first-level cache is a SqlSession-level cache, while the second-level cache is a Mapper-level cache. In practical applications, rational use of these two caches is an important means to improve system performance. This article will analyze the best practices of first-level cache and second-level cache in MyBatis through specific code examples.
1. First-level cache
- Principle of first-level cache
In MyBatis, each SqlSession maintains a local cache, and It's the first level cache. When executing the same query in the same SqlSession, MyBatis will first search from the first-level cache. If the corresponding result is found, it will be returned directly without querying the database.
- The life cycle of the first-level cache
The life cycle of the first-level cache is the same as the life cycle of SqlSession. That is to say, as long as the SqlSession is not closed, the life cycle of the first-level cache The data will be retained.
- Code Example
The following is a simple example code that demonstrates the use of the first-level cache:
// 获取SqlSession SqlSession sqlSession = sqlSessionFactory.openSession(); // 创建Mapper接口代理对象 UserMapper userMapper = sqlSession.getMapper(UserMapper.class); // 第一次查询 User user1 = userMapper.selectUserById(1); // 第二次查询,因为是同一个SqlSession,会从一级缓存中获取结果 User user2 = userMapper.selectUserById(1); // 关闭SqlSession sqlSession.close();
In the above code, the The first and second queries use the same id. Since the operation is performed in the same SqlSession, the second query will obtain the results directly from the first-level cache.
2. Second-level cache
- The principle of second-level cache
The second-level cache is a Mapper-level cache. Multiple SqlSession shares the same Mapper. Second level cache of objects. When multiple SqlSession queries the same data of the same Mapper, it will first search from the second-level cache. If found, it will be returned directly without querying the database.
- Configuration of the second-level cache
To use the second-level cache, you need to make the corresponding configuration in the MyBatis configuration file:
<setting name="cacheEnabled" value="true"/>
- Code Example
The following is a simple sample code that demonstrates the use of second-level cache:
// 获取第一个SqlSession SqlSession sqlSession1 = sqlSessionFactory.openSession(); UserMapper userMapper1 = sqlSession1.getMapper(UserMapper.class); User user1 = userMapper1.selectUserById(1); sqlSession1.close(); // 获取第二个SqlSession SqlSession sqlSession2 = sqlSessionFactory.openSession(); UserMapper userMapper2 = sqlSession2.getMapper(UserMapper.class); User user2 = userMapper2.selectUserById(1); sqlSession2.close();
In the above code, the first and second SqlSession queries With the same ID, since the second-level cache is turned on, the second query will directly obtain the results from the second-level cache.
3. Cache Invalidation
- Caching Invalidation
Although caching can improve system performance, cache invalidation may occur in some cases. In this case, the cache needs to be cleared in time to ensure data accuracy. Common cache failure situations include: data update, manual cache cleaning, cache expiration, etc.
- Cache invalidation processing
For the first-level cache, when a cache invalidation occurs, you only need to close the current SqlSession to clear the cache. For the second-level cache, corresponding methods need to be used to clean or update the cache data.
Conclusion
Reasonable use of the first-level cache and the second-level cache can improve system performance, but you need to pay attention to the cache failure and clear the cache in time to avoid data inconsistency. In actual projects, appropriate caching strategies need to be selected based on specific needs to improve system performance and user experience.
The above is about the analysis of MyBatis cache strategy and the best practices of first-level cache and second-level cache. I hope it will be helpful to you.
The above is the detailed content of MyBatis cache strategy analysis: best practices for first-level cache and second-level cache. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
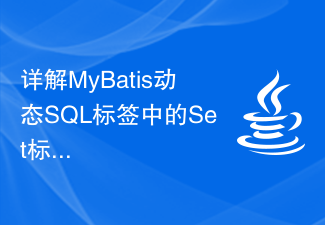
Interpretation of MyBatis dynamic SQL tags: Detailed explanation of Set tag usage MyBatis is an excellent persistence layer framework. It provides a wealth of dynamic SQL tags and can flexibly construct database operation statements. Among them, the Set tag is used to generate the SET clause in the UPDATE statement, which is very commonly used in update operations. This article will explain in detail the usage of the Set tag in MyBatis and demonstrate its functionality through specific code examples. What is Set tag Set tag is used in MyBati
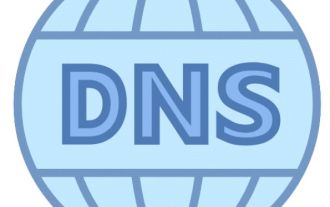
DNS (DomainNameSystem) is a system used on the Internet to convert domain names into corresponding IP addresses. In Linux systems, DNS caching is a mechanism that stores the mapping relationship between domain names and IP addresses locally, which can increase the speed of domain name resolution and reduce the burden on the DNS server. DNS caching allows the system to quickly retrieve the IP address when subsequently accessing the same domain name without having to issue a query request to the DNS server each time, thereby improving network performance and efficiency. This article will discuss with you how to view and refresh the DNS cache on Linux, as well as related details and sample code. Importance of DNS Caching In Linux systems, DNS caching plays a key role. its existence
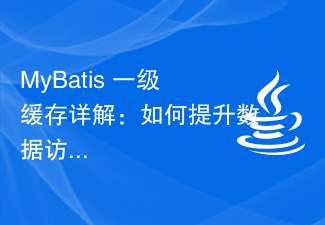
Detailed explanation of MyBatis first-level cache: How to improve data access efficiency? During the development process, efficient data access has always been one of the focuses of programmers. For persistence layer frameworks like MyBatis, caching is one of the key methods to improve data access efficiency. MyBatis provides two caching mechanisms: first-level cache and second-level cache. The first-level cache is enabled by default. This article will introduce the mechanism of MyBatis first-level cache in detail and provide specific code examples to help readers better understand

Analysis of MyBatis' caching mechanism: The difference and application of first-level cache and second-level cache In the MyBatis framework, caching is a very important feature that can effectively improve the performance of database operations. Among them, first-level cache and second-level cache are two commonly used caching mechanisms in MyBatis. This article will analyze the differences and applications of first-level cache and second-level cache in detail, and provide specific code examples to illustrate. 1. Level 1 Cache Level 1 cache is also called local cache. It is enabled by default and cannot be turned off. The first level cache is SqlSes
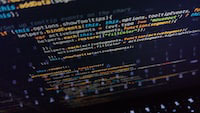
Optimizing Cache Size and Cleanup Strategies It is critical to allocate appropriate cache size to APCu. A cache that is too small cannot cache data effectively, while a cache that is too large wastes memory. Generally speaking, setting the cache size to 1/4 to 1/2 of the available memory is a reasonable range. Additionally, having an effective cleanup strategy ensures that stale or invalid data is not kept in the cache. You can use APCu's automatic cleaning feature or implement a custom cleaning mechanism. Sample code: //Set the cache size to 256MB apcu_add("cache_size",268435456); //Clear the cache every 60 minutes apcu_add("cache_ttl",60*60); Enable compression
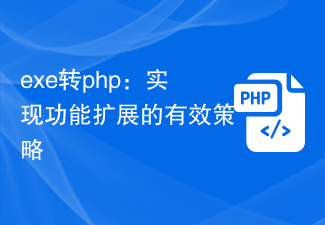
EXE to PHP: An effective strategy to achieve function expansion. With the development of the Internet, more and more applications have begun to migrate to the web to achieve wider user access and more convenient operations. In this process, the demand for converting functions originally run as EXE (executable files) into PHP scripts is also gradually increasing. This article will discuss how to convert EXE to PHP to achieve functional expansion, and give specific code examples. Why Convert EXE to PHP Cross-Platformness: PHP is a cross-platform language
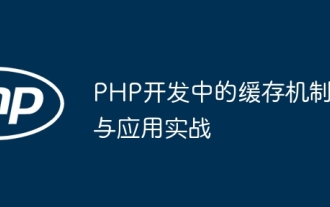
In PHP development, the caching mechanism improves performance by temporarily storing frequently accessed data in memory or disk, thereby reducing the number of database accesses. Cache types mainly include memory, file and database cache. Caching can be implemented in PHP using built-in functions or third-party libraries, such as cache_get() and Memcache. Common practical applications include caching database query results to optimize query performance and caching page output to speed up rendering. The caching mechanism effectively improves website response speed, enhances user experience and reduces server load.

PHPAPCu (replacement of php cache) is an opcode cache and data cache module that accelerates PHP applications. Understanding its advanced features is crucial to utilizing its full potential. 1. Batch operation: APCu provides a batch operation method that can process a large number of key-value pairs at the same time. This is useful for large-scale cache clearing or updates. //Get cache keys in batches $values=apcu_fetch(["key1","key2","key3"]); //Clear cache keys in batches apcu_delete(["key1","key2","key3"]);2 .Set cache expiration time: APCu allows you to set an expiration time for cache items so that they automatically expire after a specified time.
