


Take advantage of the Go language to build efficient and scalable web applications
Go language, as an efficient and highly concurrent programming language, has gradually emerged in the field of Web development. This article will explore how to take advantage of the Go language to build efficient and scalable web applications, and provide specific code examples.
When building web applications, we often encounter challenges in the following aspects: high performance requirements, complex concurrency processing, strong scalability, etc. The Go language precisely meets these needs. It natively supports concurrent programming and encapsulates a standard library with excellent performance, allowing us to better meet these challenges.
First, let us look at a simple HTTP server example to demonstrate the concurrent processing capabilities of the Go language:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
The above code shows a simple HTTP server. When a request is received , will return "Hello, world!". Through the http.ListenAndServe
function, we start an HTTP server that can handle multiple concurrent requests without us having to worry about thread management and other issues ourselves.
Next, we will introduce how to use the Gin framework to build a more complete web application:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
|
In this example, we use the Gin framework to quickly build an HTTP server. The Gin framework provides middleware support, request processing functions and other features, allowing us to process HTTP requests and build routes more conveniently.
In addition to the basic HTTP server, the Go language also provides a wealth of third-party libraries to help us build efficient and scalable web applications. For example, using the gorm
library can make it easier to operate the database, and using the jwt-go
library can implement functions such as user authentication and authorization.
In general, the Go language has great advantages in building efficient and scalable web applications. By making full use of its concurrency features and rich third-party libraries and frameworks, we can quickly build web application systems with excellent performance and easy maintenance. We hope that the code examples provided in this article can help you better take advantage of the Go language and build web applications that meet your needs.
The above is the detailed content of Take advantage of the Go language to build efficient and scalable web applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
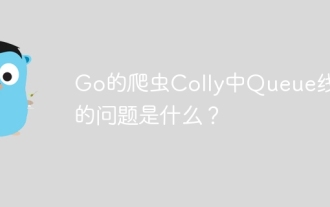
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
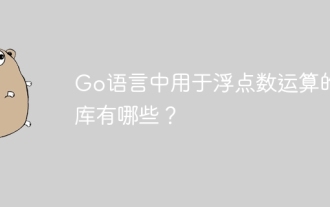
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
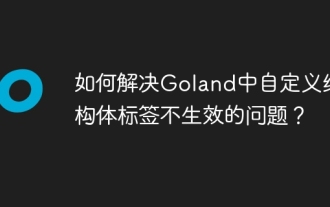
Regarding the problem of custom structure tags in Goland When using Goland for Go language development, you often encounter some configuration problems. One of them is...
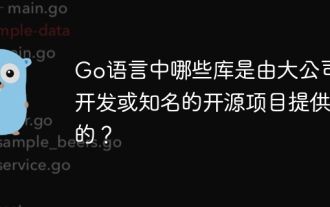
Which libraries in Go are developed by large companies or well-known open source projects? When programming in Go, developers often encounter some common needs, ...
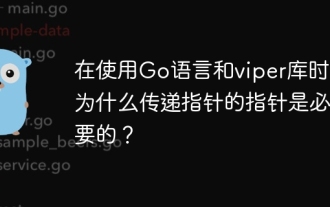
Go pointer syntax and addressing problems in the use of viper library When programming in Go language, it is crucial to understand the syntax and usage of pointers, especially in...
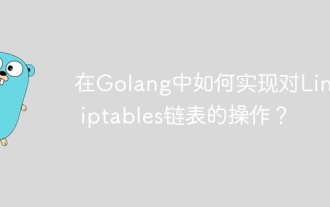
Using Golang to implement Linux...
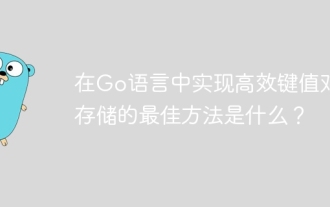
The correct way to implement efficient key-value pair storage in Go language How to achieve the best performance when developing key-value pair memory similar to Redis in Go language...
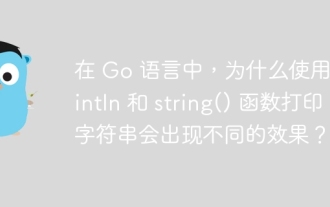
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
