Advantages and limitations of MyBatis reverse engineering
MyBatis is a popular persistence framework that provides reverse engineering functions, which allows developers to automatically generate entity classes, Mapper interfaces and XML based on the table structure in the database mapping file. Reverse engineering is an important feature of MyBatis, which can greatly reduce the developer's workload and improve the maintainability of the code. However, reverse engineering also has some limitations. This article will introduce the advantages and limitations of MyBatis reverse engineering and illustrate it with specific code examples.
First, let's take a look at the advantages of MyBatis reverse engineering. Reverse engineering can automatically generate entity classes, Mapper interfaces and XML mapping files based on the table structure in the database. This way, developers do not need to manually write these codes, thus saving a lot of time and energy. In addition, reverse engineering can also generate code that conforms to specifications, with high code quality and strong readability, which is very helpful for teamwork and long-term maintenance of the project.
Secondly, let's take a look at the limitations of MyBatis reverse engineering. Reverse engineering mainly faces limitations in two aspects: the complexity of the table structure and the customization of reverse engineering. First, if the table structure in the database is very complex, the code generated by reverse engineering may become very large, which will make code management difficult. Secondly, reverse engineering can usually only generate simple addition, deletion, modification and query methods based on the table structure. For some complex business logic, developers also need to manually write code. In addition, the code generated by reverse engineering usually operates on a single table. If multiple table operations are required, developers also need to write the code manually. Therefore, reverse engineering cannot completely replace manual writing of code. It is only a starting point, and developers also need to perform secondary development based on specific needs.
The following is a specific code example that shows how to use the code generated by MyBatis reverse engineering to perform simple database operations.
First, we need to configure reverse engineering related information in the MyBatis configuration file. The specific configuration is as follows:
<!-- 配置逆向工程 --> <generatorConfiguration> <classPathEntry location="/path/to/driver.jar" /> <context id="MyBatis" targetRuntime="MyBatis3"> <jdbcConnection driverClass="com.mysql.jdbc.Driver" connectionURL="jdbc:mysql://localhost:3306/mydatabase" userId="root" password="root" /> <javaModelGenerator targetPackage="com.example.model" targetProject="/path/to/project/src/main/java" /> <sqlMapGenerator targetPackage="com.example.mapper" targetProject="/path/to/project/src/main/resources" /> <javaClientGenerator targetPackage="com.example.mapper" targetProject="/path/to/project/src/main/java" type="XMLMAPPER" /> <table tableName="user"></table> </context> </generatorConfiguration>
The jdbcConnection
tag in the configuration file is used to configure database connection related information, and the javaModelGenerator
tag is used to configure the generation path and package name of the entity class , sqlMapGenerator
tag is used to configure the path and package name generated by the Mapper XML file, javaClientGenerator
tag is used to configure the generated path and package name of the Mapper interface, table
tag Used to configure the table name to be reverse engineered to generate code.
Next, we can use the following code to perform database operations:
public interface UserMapper { int insert(User record); int insertSelective(User record); } public class UserDao { @Resource private UserMapper userMapper; public void saveUser(User user) { userMapper.insert(user); } public void updateUser(User user) { userMapper.updateByPrimaryKeySelective(user); } public void deleteUser(int userId) { userMapper.deleteByPrimaryKey(userId); } public User getUserById(int userId) { return userMapper.selectByPrimaryKey(userId); } } public class Main { public static void main(String[] args) { UserDao userDao = new UserDao(); User user = new User(); user.setId(1); user.setUsername("John"); user.setPassword("123456"); userDao.saveUser(user); User savedUser = userDao.getUserById(1); System.out.println(savedUser.getUsername()); } }
In the above code, UserMapper
is the Mapper interface automatically generated through reverse engineering, UserDao
is an encapsulation class for database operations. Database operations are performed by calling the methods in UserMapper
. The Main
class is a test class that demonstrates how to use UserDao
to perform database operations.
In summary, MyBatis reverse engineering has the advantages of simplicity, speed, and improved development efficiency, but it also has limitations in table structure complexity and customization. When developers use the code generated by reverse engineering, they need to conduct appropriate secondary development based on specific business needs.
The above is the detailed content of Advantages and limitations of MyBatis reverse engineering. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


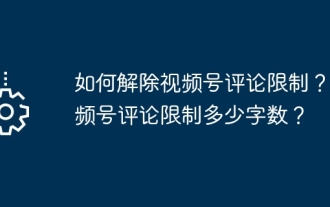
With the popularity of video accounts on social media, more and more people are beginning to use video accounts to share their daily lives, insights and stories. However, some users may experience comments being restricted, which can leave them confused and dissatisfied. 1. How to remove comment restrictions on video accounts? To lift the restriction on commenting on a video account, you must first ensure that the account has been properly registered and real-name authentication has been completed. Video accounts have requirements for comments. Only accounts that have completed real-name authentication can lift comment restrictions. If there are any abnormalities in the account, these issues need to be resolved before comment restrictions can be lifted. 2. Comply with the community standards of the video account. Video accounts have certain standards for comment content. If the comment involves illegal content, you will be restricted from speaking. To lift comment restrictions, you need to abide by the community of the video account
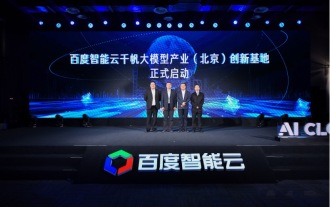
Serving 80,000 enterprise users, it has helped users fine-tune 13,000 large models and helped users develop 160,000 large model applications. Since December 2023, the daily API calls of Baidu Smart Cloud Qianfan Large Model Platform have increased by 97% month-on-month. ..From the "pioneer" of the domestic large model platform a year ago to today's large model "super factory", Baidu Intelligent Cloud Qianfan large model platform firmly occupies a leading position in the domestic large model market, but its pace is slow. Didn't stop. On March 21, Baidu Intelligent Cloud held a Qianfan product launch conference in Beijing Shougang Park. Baidu Intelligent Cloud announced during the conference: 1. Joining hands with Beijing Shijingshan District to build the country's first Baidu Intelligent Cloud Qianfan large-scale model industrial innovation base to help Promote the take-off of regional industries; 2. Satisfy the “valency” of enterprises
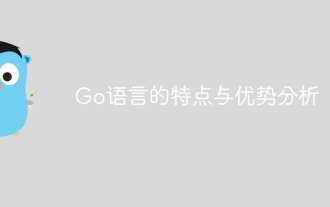
Features of Go language: High concurrency (goroutine) Automatic garbage collection Cross-platform simplicity Modularity Advantages of Go language: High performance Security Scalability Community support
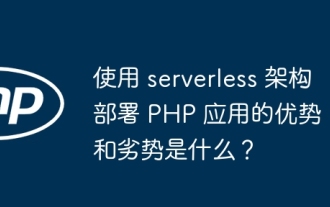
Deploying PHP applications using Serverless architecture has the following advantages: maintenance-free, pay-as-you-go, highly scalable, simplified development and support for multiple services. Disadvantages include: cold start time, debugging difficulties, vendor lock-in, feature limitations, and cost optimization challenges.
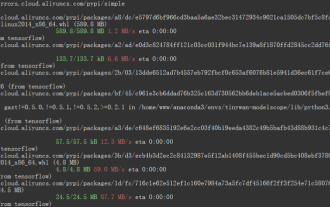
Overview In order to enable ModelScope users to quickly and conveniently use various models provided by the platform, a set of fully functional Python libraries are provided, which includes the implementation of ModelScope official models, as well as the necessary tools for using these models for inference, finetune and other tasks. Code related to data pre-processing, post-processing, effect evaluation and other functions, while also providing a simple and easy-to-use API and rich usage examples. By calling the library, users can complete tasks such as model reasoning, training, and evaluation by writing just a few lines of code. They can also quickly perform secondary development on this basis to realize their own innovative ideas. The algorithm model currently provided by the library is:
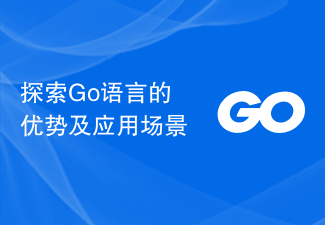
The Go language is an open source programming language developed by Google and first released in 2007. It is designed to be a simple, easy-to-learn, efficient, and highly concurrency language, and is favored by more and more developers. This article will explore the advantages of Go language, introduce some application scenarios suitable for Go language, and give specific code examples. Advantages: Strong concurrency: Go language has built-in support for lightweight threads-goroutine, which can easily implement concurrent programming. Goroutin can be started by using the go keyword
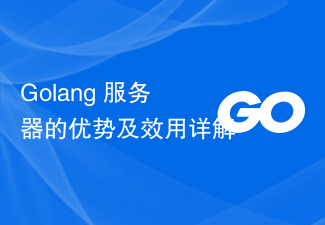
Golang is an open source programming language developed by Google. It is efficient, fast and powerful and is widely used in cloud computing, network programming, big data processing and other fields. As a strongly typed, static language, Golang has many advantages when building server-side applications. This article will analyze the advantages and utility of Golang server in detail, and illustrate its power through specific code examples. 1. The high-performance Golang compiler can compile the code into local code

Golang's single-threaded features and advantages With the booming development of the Internet and mobile applications, the demand for high-performance, high-concurrency programming languages is increasing. Against this background, the Go language (Golang for short) was developed by Google and first released in 2009, and quickly became popular among developers. Golang is an open source programming language that uses static typing and concurrent design. One of its biggest advantages is its single-threaded feature. Golang adopts Goroutine’s concurrency model.
