Master the definition and initialization skills of Java arrays
To master the definition and initialization skills of Java arrays, specific code examples are required
In Java programming, arrays are a common and important data structure. It can store multiple elements of the same type, making it easier for us to process and operate data. Mastering the definition and initialization skills of arrays is the basis for writing efficient code. This article will introduce the definition and initialization techniques of Java arrays through specific code examples.
1. Definition and declaration of arrays
To define an array, we need to declare the type and name of the array first, then use the keyword new to create an array object and specify the length of the array. The following example demonstrates how to define an array of integers.
// 定义一个整型数组 int[] arr;
2. Static initialization of array
Static initialization means directly assigning values to array elements when defining an array. During static initialization, we can specify specific values for each element of the array. The following example demonstrates how to use static initialization to define an integer array and assign values to the elements of the array.
// 定义并初始化一个整型数组 int[] arr = {1, 2, 3, 4, 5};
3. Dynamic initialization of array
Dynamic initialization means that only the length of the array is specified when defining the array without initializing the value. During dynamic initialization, Java assigns a default value to each element of the array. The following example demonstrates how to use dynamic initialization to define an integer array.
// 定义一个整型数组,长度为5 int[] arr = new int[5];
4. Initializing the array through loops
Sometimes we need to assign certain rules or specific values to each element of the array. In this case, we can initialize the array through a loop. The following example demonstrates how to initialize an integer array using a loop.
// 定义一个整型数组,长度为5 int[] arr = new int[5]; // 使用循环为数组每个元素赋值 for (int i = 0; i < arr.length; i++) { arr[i] = i + 1; }
5. Initialization of multi-dimensional arrays
In addition to one-dimensional arrays, Java also supports multi-dimensional arrays. Multidimensional arrays can be thought of as arrays of arrays, and we need to specify the length of each dimension. The following example demonstrates how to define and initialize a two-dimensional array of integers.
// 定义并初始化一个二维整型数组 int[][] matrix = {{1, 2, 3}, {4, 5, 6}};
6. Summary
Through the introduction of this article, we have learned about the definition and initialization techniques of Java arrays, including static initialization, dynamic initialization and array initialization through loops. Additionally, we learned how multidimensional arrays are defined and initialized. Mastering these skills will be of great help in developing Java programs.
In actual programming, choosing an appropriate way to define and initialize arrays according to actual needs can improve the efficiency and readability of the program. I hope the sample code in this article can help you better master the definition and initialization skills of Java arrays.
The above is the detailed content of Master the definition and initialization skills of Java arrays. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


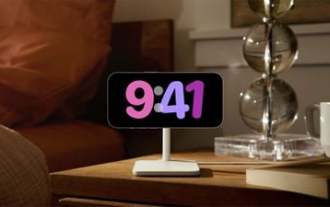
Standby is a lock screen mode that activates when the iPhone is plugged into the charger and oriented in horizontal (or landscape) orientation. It consists of three different screens, one of which is displayed full screen time. Read on to learn how to change the style of your clock. StandBy's third screen displays times and dates in various themes that you can swipe vertically. Some themes also display additional information, such as temperature or next alarm. If you hold down any clock, you can switch between different themes, including Digital, Analog, World, Solar, and Floating. Float displays the time in large bubble numbers in customizable colors, Solar has a more standard font with a sun flare design in different colors, and World displays the world by highlighting
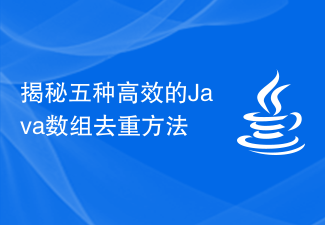
Five efficient Java array deduplication methods revealed In the Java development process, we often encounter situations where we need to deduplicate arrays. Deduplication is to remove duplicate elements in an array and keep only one. This article will introduce five efficient Java array deduplication methods and provide specific code examples. Method 1: Use HashSet to deduplicate HashSet is an unordered, non-duplicate collection that automatically deduplicates when adding elements. Therefore, we can use the characteristics of HashSet to deduplicate arrays. public

The definition of short video refers to high-frequency pushed video content that is played on various new media platforms, suitable for viewing on the move and in a short-term leisure state, and is generally spread on new Internet media within 5 minutes. Video; the content combines skills sharing, humor, fashion trends, social hot spots, street interviews, public welfare education, advertising creativity, business customization and other themes. Short videos have the characteristics of simple production process, low production threshold, and strong participation.
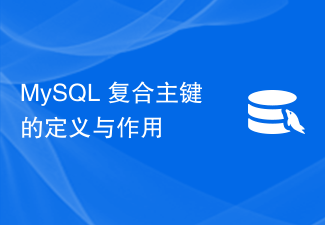
The composite primary key in MySQL refers to the primary key composed of multiple fields in the table, which is used to uniquely identify each record. Unlike a single primary key, a composite primary key is formed by combining the values of multiple fields. When creating a table, you can define a composite primary key by specifying multiple fields as primary keys. In order to demonstrate the definition and function of composite primary keys, we first create a table named users, which contains three fields: id, username and email, where id is an auto-incrementing primary key and user
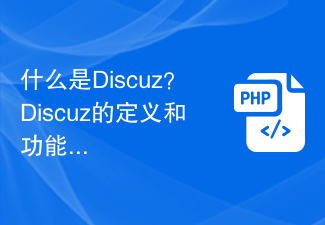
"Exploring Discuz: Definition, Functions and Code Examples" With the rapid development of the Internet, community forums have become an important platform for people to obtain information and exchange opinions. Among the many community forum systems, Discuz, as a well-known open source forum software in China, is favored by the majority of website developers and administrators. So, what is Discuz? What functions does it have, and how can it help our website? This article will introduce Discuz in detail and attach specific code examples to help readers learn more about it.
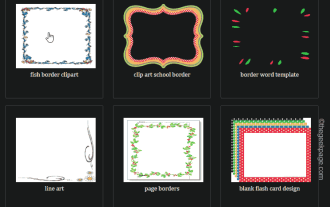
Want to make the front page of your school project look exciting? Nothing makes it stand out from other submissions like a nice, elegant border on the homepage of your workbook. However, the standard single-line borders in Microsoft Word have become very obvious and boring. Therefore, we show you the steps to create and use custom borders in Microsoft Word documents. How to Make Custom Borders in Microsoft Word Creating custom borders is very easy. However, you will need a boundary. Step 1 – Download Custom Borders There are tons of free borders on the internet. We have downloaded a border like this. Step 1 – Search the Internet for custom borders. Alternatively, you can go to clipping
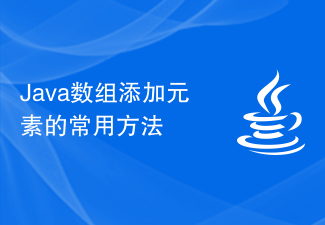
Common ways to add elements to Java arrays, specific code examples are required In Java, an array is a common data structure that can store multiple elements of the same type. In actual development, we often need to add new elements to the array. This article will introduce common methods of adding elements to arrays in Java and provide specific code examples. A simple way to create a new array using a loop is to create a new array, copy the elements of the old array into the new array, and add the new elements. The code example is as follows: //original array i
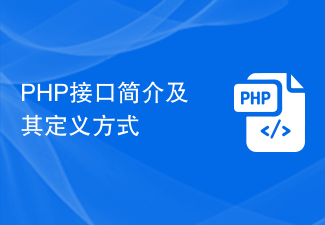
Introduction to PHP interface and how it is defined. PHP is an open source scripting language widely used in Web development. It is flexible, simple, and powerful. In PHP, an interface is a tool that defines common methods between multiple classes, achieving polymorphism and making code more flexible and reusable. This article will introduce the concept of PHP interfaces and how to define them, and provide specific code examples to demonstrate their usage. 1. PHP interface concept Interface plays an important role in object-oriented programming, defining the class application
